When a browser parses a web page and begins to discover and download resources
such as images, scripts, or CSS, it assigns them a fetch priority
so it can
download them in an optimal order. A resource's priority usually depends on what
it is and where it is in the document. For example, in-viewport images might
have a High
priority, and the priority for early loaded, render-blocking CSS
using <link>
s in the <head>
might be Very High
. Automatic priority
assignment usually works well, but there are use cases where the assigned order
isn't optimal.
This page discusses the Fetch Priority API and the fetchpriority
HTML
attribute, which help you optimize the Core Web Vitals by letting you hint at
the relative priority of a resource (high
or low
).
Summary
A few key areas where Fetch Priority can help:
- Boosting the priority of the LCP image by specifying
fetchpriority="high"
on the image element, causing LCP to happen sooner. - Increasing the priority of
async
scripts, using better semantics than the current most common hack (inserting a<link rel="preload">
for theasync
script). - Decreasing the priority of late-body scripts to sequence images better.
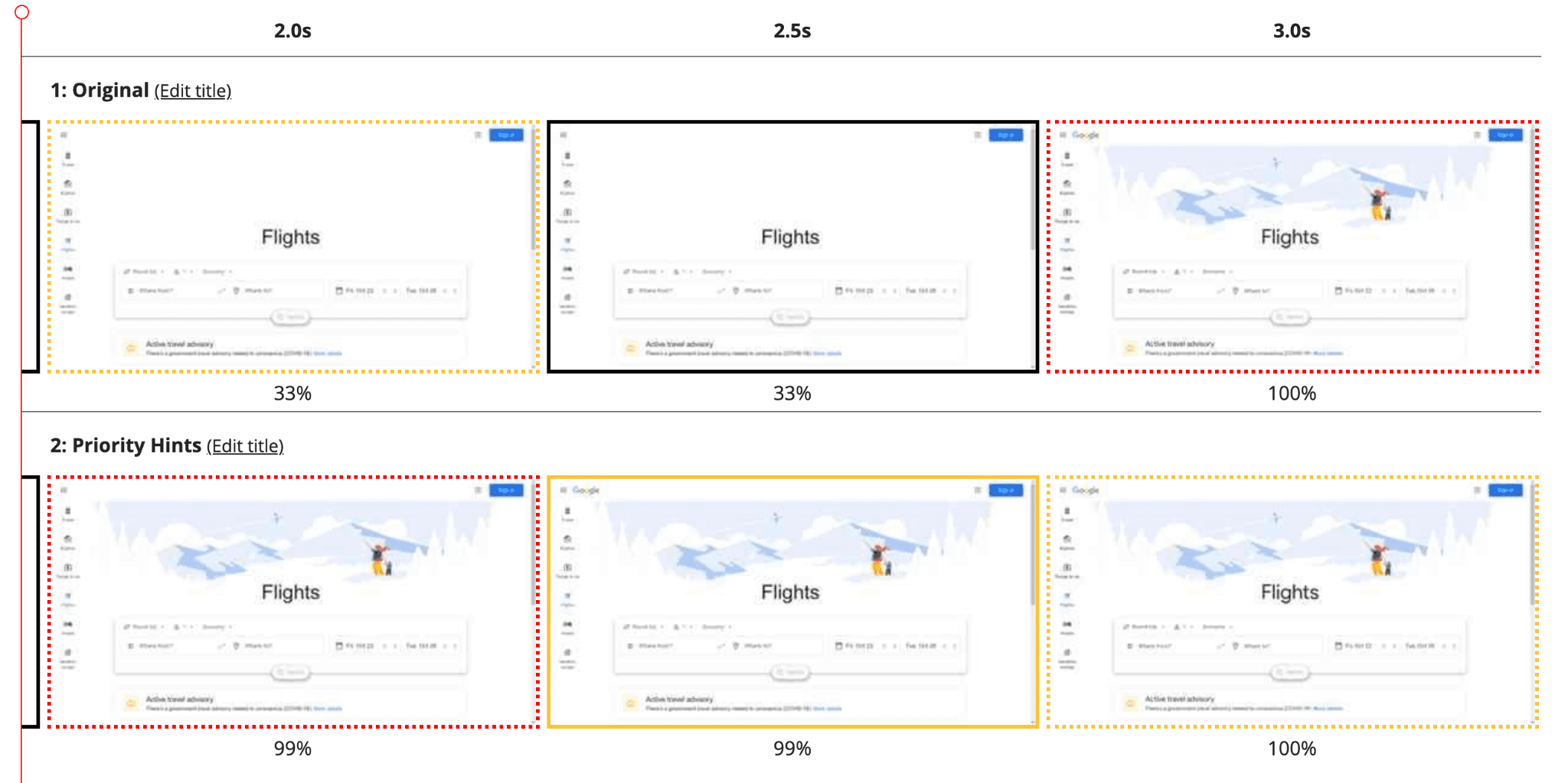
Historically, developers have had limited influence over resource priority using preload and preconnect. Preload lets you tell the browser about critical resources you want to load early before the browser would naturally discover them. This is especially useful for resources that are harder to discover, such as fonts included in stylesheets, background images, or resources loaded from a script. Preconnect helps warm up connections to cross-origin servers and can help improve metrics like Time to first byte. It's useful when you know an origin but not necessarily the exact URL of a resource that will be needed.
Fetch Priority complements these Resource Hints.
It's a markup-based signal available through the fetchpriority
attribute that
developers can use to indicate the relative priority of a particular resource.
You can also use these hints through JavaScript and the
Fetch API with the priority
property to
influence the priority of resource fetches made for data. Fetch Priority can
also complement preload. Take a Largest Contentful Paint image, which, when
preloaded, will still get a low priority. If it is pushed back by other early
low-priority resources, using Fetch Priority can help how soon the image gets
loaded.
Resource priority
The resource download sequence depends on the browser's assigned priority for every resource on the page. The factors that can affect priority computation logic include the following:
- The type of resource, such as CSS, fonts, scripts, images, and third-party resources.
- The location or order the document references resources in.
- The
preload
resource hint, which helps the browser to discover a resource faster and load it earlier. - Priority computation changes for
async
ordefer
scripts.
The following table shows how Chrome prioritizes and sequences most resources:
Load in layout-blocking phase | Load one-at-a-time in layout-blocking phase | ||||
---|---|---|---|---|---|
Blink Priority |
VeryHigh | High | Medium | Low | VeryLow |
DevTools Priority |
Highest | High | Medium | Low | Lowest |
Main resource | |||||
CSS (early**) | CSS (late**) | CSS (media mismatch***) | |||
Script (early** or not from preload scanner) | Script (late**) | Script (async) | |||
Font | Font (rel=preload) | ||||
Import | |||||
Image (in viewport) | Image (first 5 images > 10,000px2) | Image | |||
Media (video/audio) | |||||
Prefetch | |||||
XSL | |||||
XHR (sync) | XHR/fetch* (async) |
The browser downloads resources with the same computed priority in the order they're discovered. You can check the priority assigned to different resources when loading a page under the Chrome Dev Tools Network tab. (Make sure you include the priority column by right-clicking the table headings).
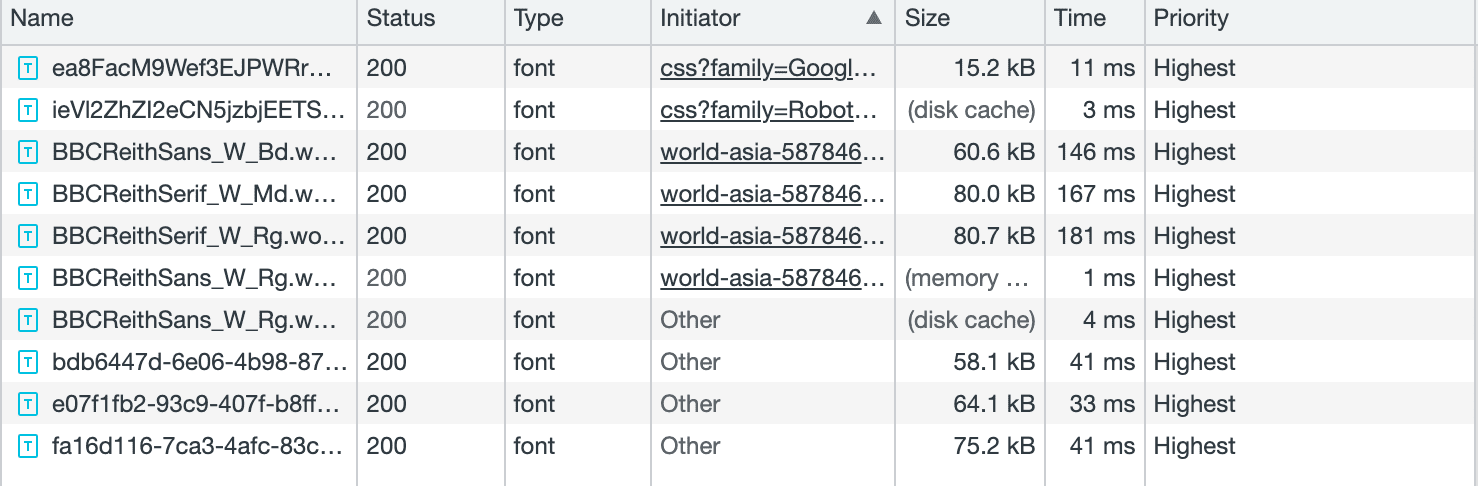
type = "font"
on BBC news detail page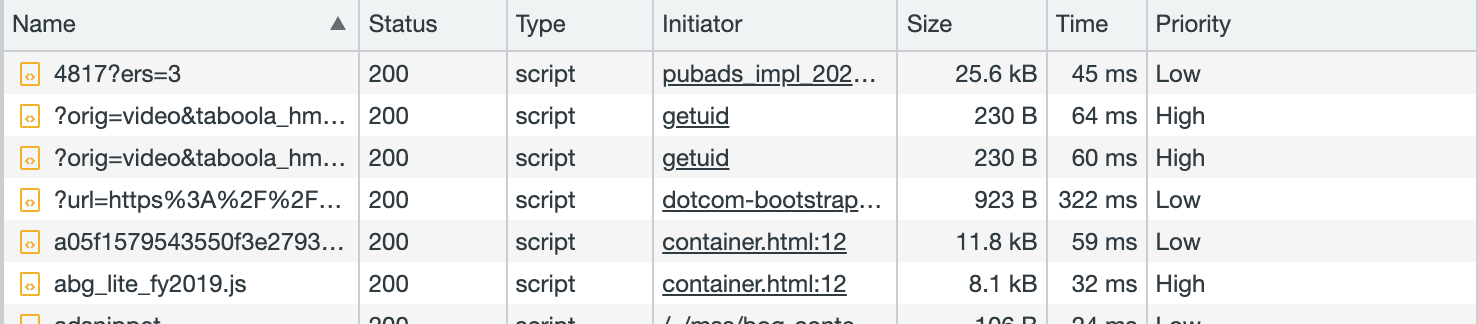
When priorities change, you can see both the initial and final priority in the Big request rows setting or in a tooltip.
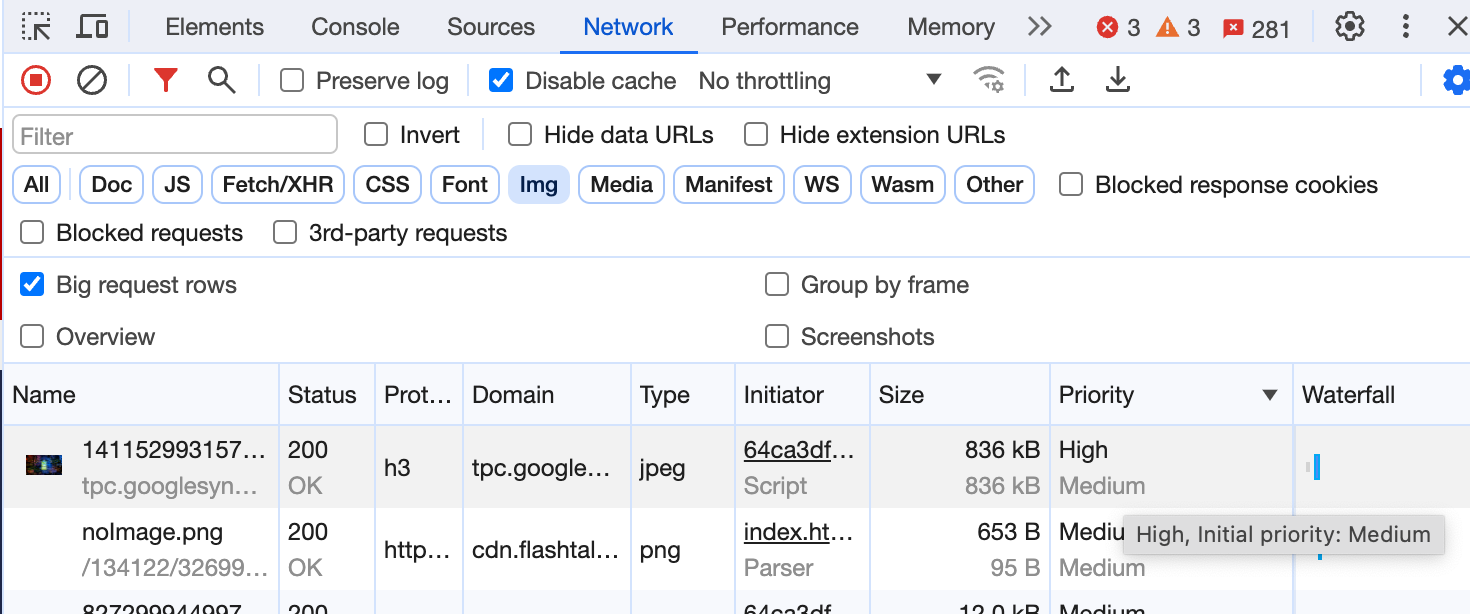
When might you need Fetch Priority?
Now that you understand the browser's prioritization logic, you can adjust your page's download order to optimize its performance and Core Web Vitals. Here are some examples of things you can change without using Fetch Priority:
- Place resource tags like
<script>
and<link>
in the order you want the browser to download them. - Use the
preload
resource hint to download necessary resources earlier, especially resources that are harder for the browser to discover. - Use
async
ordefer
to download scripts without blocking other resources. - Lazy-load below-the-fold content so the browser can use the available bandwidth for more critical above-the-fold resources.
Here are some more complex cases where Fetch Priority can help you get the resource priority order you need:
- You have several above-the-fold images, but not all of them should have the same priority. For example, in an image carousel, only the first visible image needs a higher priority.
- Hero images inside the viewport typically start at a
Low
orMedium
priority. After the layout is complete, Chrome discovers that they're in the viewport and boosts their priority. This usually adds a significant delay to loading the images. Providing the Fetch Priority in markup lets the image start at a "High" priority and start loading much earlier.
Preload is still required for early discovery of LCP images included as CSS backgrounds. To boost your background images' priority, includefetchpriority='high'
on the preload. - Declaring scripts as
async
ordefer
tells the browser to load them asynchronously. However, as shown in the priority table, these scripts are also assigned a "Low" priority. You might want to increase their priority while ensuring asynchronous download, especially for scripts that are critical for the user experience. - If you use the JavaScript
fetch()
API to fetch resources or data asynchronously, the browser assigns itHigh
priority. You might want some of your fetches to run with lower priority, especially if you're mixing background API calls with API calls that respond to user input. Mark the background API calls asLow
priority and the interactive API calls asHigh
priority. - The browser assigns CSS and fonts a
High" priority
, but some of those resources might be more important than others. You can use Fetch Priority to lower the priority of non-critical resources.
The fetchpriority
attribute
Use the fetchpriority
HTML attribute to specify download priority for resource
types such as CSS, fonts, scripts, and images when downloaded using link
,
img
, or script
tags. It can take the following values:
high
: The resource is a high priority, and you want the browser to prioritize it as long as the browser's own heuristics don't prevent that from happening.low
: The resource is a low priority, and you want the browser to deprioritize it if its heuristics let it.auto
: The default value, which lets the browser choose the appropriate priority.
Here are a few examples of using the fetchpriority
attribute in markup, as
well as the script-equivalent priority
property.
<!-- We don't want a high priority for this above-the-fold image -->
<img src="/images/in_viewport_but_not_important.svg" fetchpriority="low" alt="I'm an unimportant image!">
<!-- We want to initiate an early fetch for a resource, but also deprioritize it -->
<link rel="preload" href="/js/script.js" as="script" fetchpriority="low">
<script>
fetch('https://example.com/', {priority: 'low'})
.then(data => {
// Trigger a low priority fetch
});
</script>
Effects of browser priority and fetchpriority
You can apply the fetchpriority
attribute to different resources as shown in
the following table to increase or reduce their computed priority.
fetchpriority="auto"
(◉) in each row marks the default priority for that type
of resource.
Load in layout-blocking phase | Load one at a time in layout-blocking phase | ||||
---|---|---|---|---|---|
Blink Priority |
VeryHigh | High | Medium | Low | VeryLow |
DevTools Priority |
Highest | High | Medium | Low | Lowest |
Main Resource | ◉ | ||||
CSS (early**) | ⬆◉ | ⬇ | |||
CSS (late**) | ⬆ | ◉ | ⬇ | ||
CSS (media mismatch***) | ⬆*** | ◉⬇ | |||
Script (early** or not from preload scanner) | ⬆◉ | ⬇ | |||
Script (late**) | ⬆ | ◉ | ⬇ | ||
Script (async/defer) | ⬆ | ◉⬇ | |||
Font | ◉ | ||||
Font (rel=preload) | ⬆◉ | ⬇ | |||
Import | ◉ | ||||
Image (in viewport - after layout) | ⬆◉ | ⬇ | |||
Image (first 5 images > 10,000px2) | ⬆ | ◉ | ⬇ | ||
Image | ⬆ | ◉⬇ | |||
Media (video/audio) | ◉ | ||||
XHR (sync) - deprecated | ◉ | ||||
XHR/fetch* (async) | ⬆◉ | ⬇ | |||
Prefetch | ◉ | ||||
XSL | ◉ |
fetchpriority
sets relative priority, meaning it raises or lowers the
default priority by an appropriate amount, rather instead of explicitly setting
the priority to High
or Low
. This often results in High
or Low
priority,
but not always. For example, critical CSS with fetchpriority="high"
retains
the "VeryHigh"/"Highest" priority, and using fetchpriority="low"
on these
elements retains the "High" priority. Neither of these cases involve explicitly
setting priority to High
or Low
.
Use cases
Use the fetchpriority
attribute when you want to give the browser an extra
hint about what priority to fetch a resource with.
Increase the priority of the LCP image
You can specify fetchpriority="high"
to boost the priority of the LCP or other
critical images.
<img src="lcp-image.jpg" fetchpriority="high">
The following comparison shows the Google Flights page with an LCP background image loaded with and without Fetch Priority. With the priority set to high, the LCP improved from 2.6s to 1.9s.
Lower the priority of above-the-fold images
Use fetchpriority="low"
to lower the priority of above-the-fold images that
aren't immediately important, for example in an image carousel.
<ul class="carousel">
<img src="img/carousel-1.jpg" fetchpriority="high">
<img src="img/carousel-2.jpg" fetchpriority="low">
<img src="img/carousel-3.jpg" fetchpriority="low">
<img src="img/carousel-4.jpg" fetchpriority="low">
</ul>
In an earlier experiment with the Oodle app, we used this to lower the priority of images that don't appear on load. It decreased page load time by 2 seconds.
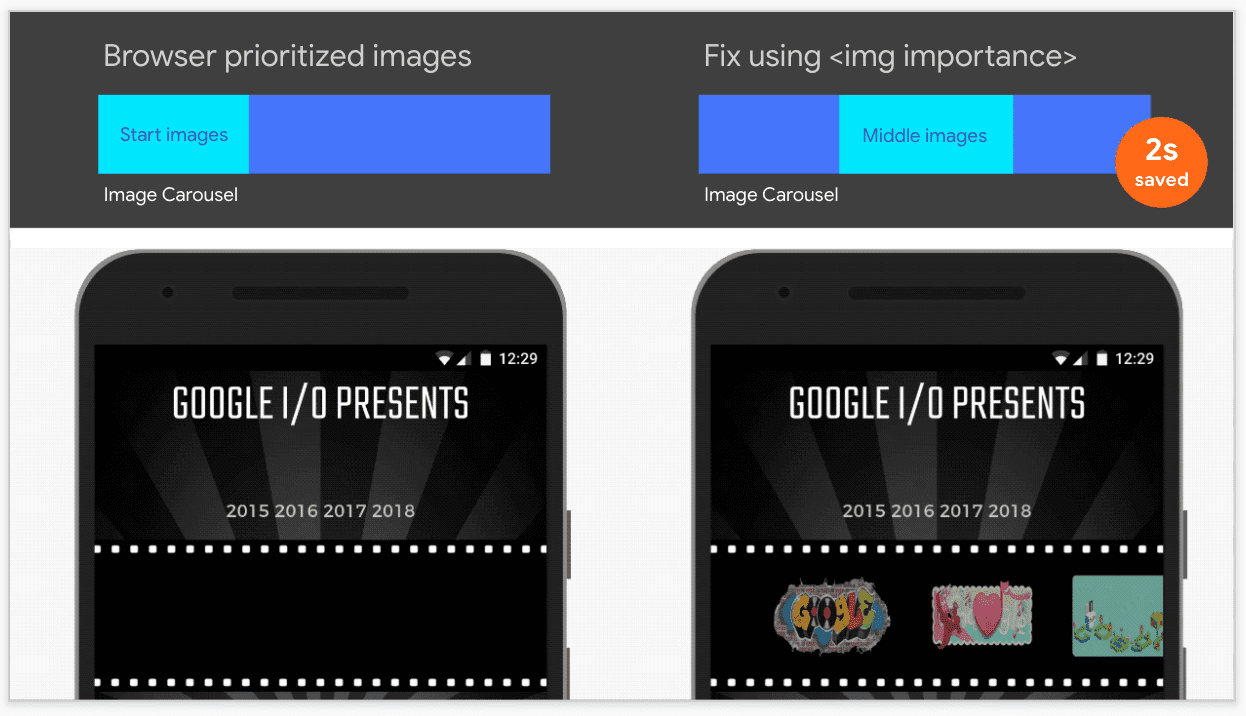
Lower the priority of preloaded resources
To stop preloaded resources from competing with other critical resources, you can reduce their priority. Use this technique with images, scripts, and CSS.
<!-- Lower priority only for non-critical preloaded scripts -->
<link rel="preload" as="script" href="critical-script.js">
<link rel="preload" href="/js/script.js" as="script" fetchpriority="low">
<!-- Preload CSS without blocking other resources -->
<link rel="preload" as="style" href="theme.css" fetchpriority="low" onload="this.rel='stylesheet'">
Reprioritize scripts
Scripts your page needs to be interactive have to load quickly, but shouldn't
block other resources. You can mark these as async
with high priority.
<script src="async_but_important.js" async fetchpriority="high"></script>
You can't mark a script as async
if it relies on specific DOM states. However,
if they run later on the page, you can load them with lower priority:
<script src="blocking_but_unimportant.js" fetchpriority="low"></script>
Lower the priority for non-critical data fetches
The browser executes fetch
with a high priority. If you have multiple fetches
that might fire simultaneously, you can use the high default priority for the
more important data fetches and lower the priority of less critical data.
// Important validation data (high by default)
let authenticate = await fetch('/user');
// Less important content data (suggested low)
let suggestedContent = await fetch('/content/suggested', {priority: 'low'});
Fetch Priority implementation notes
Here are some things to be aware of when using Fetch Priority:
- The
fetchpriority
attribute is a hint, not a directive. The browser tries to respect the developer's preference, but it can also apply its resource priority preferences for resource priority to resolve conflicts. Don't confuse Fetch Priority with preloading:
- Preload is a mandatory fetch, not a hint.
- Preload lets the browser discover a resource early, but it still fetches the resource with the default priority. Meanwhile, Fetch Priority doesn't help with discoverability, but it does let you increase or decrease the fetch priority.
- It's easier to observe and measure the effects of a preload than the effects of a priority change.
Fetch Priority can complement preloads by increasing the granularity of prioritization. If you've already specified a preload as one of the first items in the
<head>
for an LCP image, then ahigh
Fetch Priority might not improve LCP significantly. However, if the preload happens after other resources load, ahigh
Fetch Priority can improve LCP more. If a critical image is a CSS background image, preload it withfetchpriority = "high"
.Load time improvements from prioritization are more relevant in environments where more resources compete for the available network bandwidth. This is common for HTTP/1.x connections where parallel downloads aren't possible, or on low bandwidth HTTP/2 connections. In these cases, prioritization can help resolve bottlenecks.
CDNs don't implement HTTP/2 prioritization uniformly. Even if the browser communicates the priority from Fetch Priority, the CDN might not reprioritize resources in the specified order. This makes testing Fetch Priority difficult. The priorities are applied both internally within the browser and with protocols that support prioritization (HTTP/2 and HTTP/3). It's still worth using Fetch Priority just for the internal browser prioritization independent of CDN or origin support, because priorities often change when the browser requests resources. For example, low-priority resources like images are often held back from being requested while the browser processes critical
<head>
items.You might not be able to introduce Fetch Priority as a best practice in your initial design. Later in your development cycle, you can the priorities being assigned to different resources on the page, and if they don't match your expectations, you can introduce Fetch Priority for further optimization.
Tips for using preloads
Keep the following in mind when using preloads:
- Including a preload in HTTP headers puts it before everything else in the load order.
- Generally, preloads load in the order the parser gets to them for anything above "Medium" priority. Be careful if you're including preloads at the beginning of your HTML.
- Font preloads probably work best toward the end of the head or beginning of the body.
- Import preloads (dynamic
import()
ormodulepreload
) should run after the script tag that needs the import, so make sure the script gets loaded or parsed first so it can be evaluated while its dependencies are loading. - Image preloads have a "Low" or "Medium" priority by default. Order them relative to async scripts and other low or lowest priority tags.
History
Fetch Priority was first experimented with in Chrome as an origin trial in 2018,
and then again in 2021 using the importance
attribute. At that time it was
called Priority Hints. The interface
has since changed to fetchpriority
for HTML and priority
for JavaScript's
Fetch API as part of the web standards process. To reduce confusion, we now call
this API Fetch Priority.