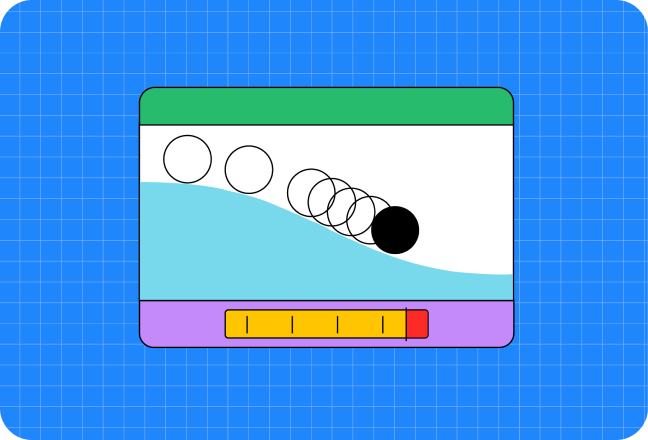
Animation patterns
These patterns demonstrate how you can create engaging animations and interactions, while respecting user's preferences for motion. They support all modern browsers, so you can use them with confidence.
Animated letters
Create infinitely animated letter effects that respect user's motion preferences.
Animated words
Create infinitely animated word effects that respect user's motion preferences.
Interactive letters
Create interactive letter effects that respect user's motion preferences.
Interactive words
Create interactive word effects that respect user's motion preferences.