The Media Session API allows websites to let users know what's currently playing in their browser and control it without returning to the page that launched it. The user experience can be customized through metadata in custom media notifications, media events such as playing, pausing, seeking, and track changing. These customizations are available in several contexts, including desktop media hubs, media notifications on mobile, and even on wearable devices.
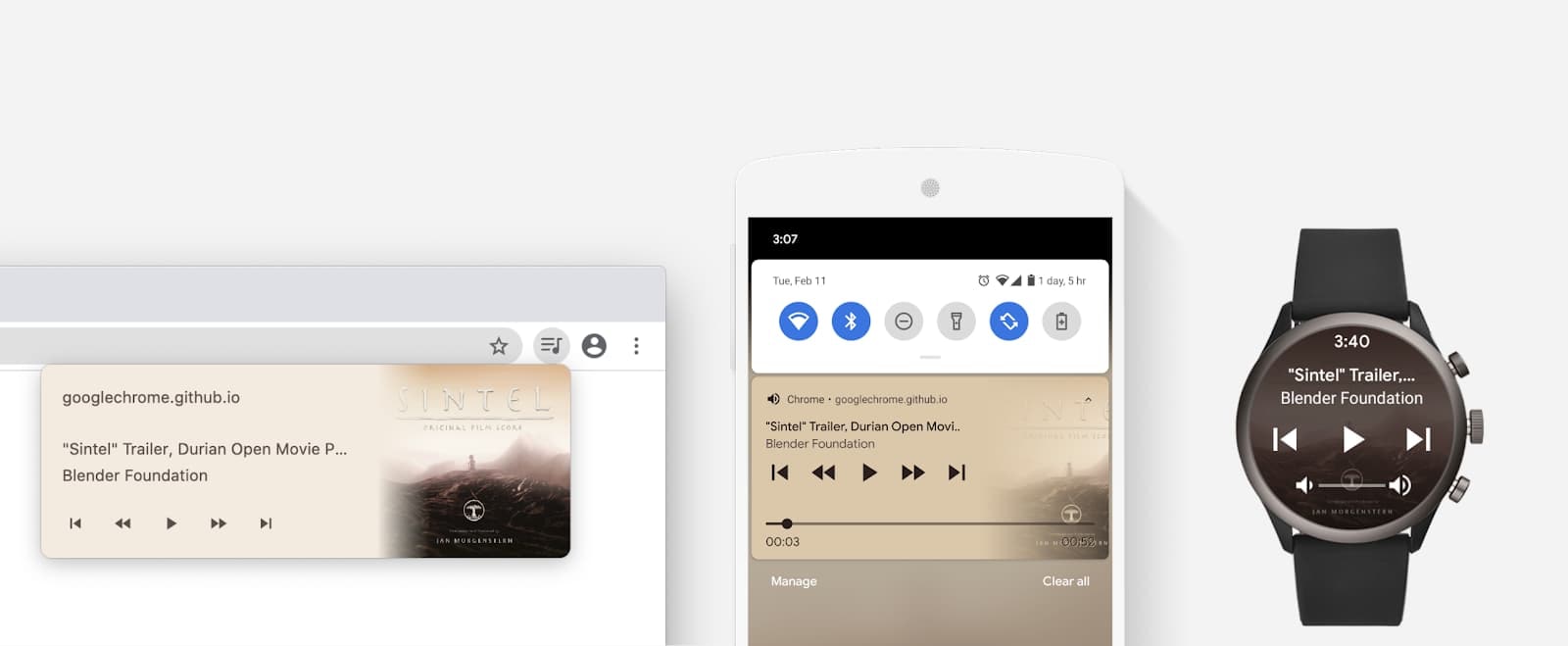
When a website is playing audio or video, users automatically get media notifications either in the notification tray on mobile, or the media hub on desktop. The browser does its best to show appropriate information by using the document's title and the largest icon image it can find. The Media Session API allows you to customize the media notification with some richer media metadata such as the title, artist name, album name, and artwork as shown below.
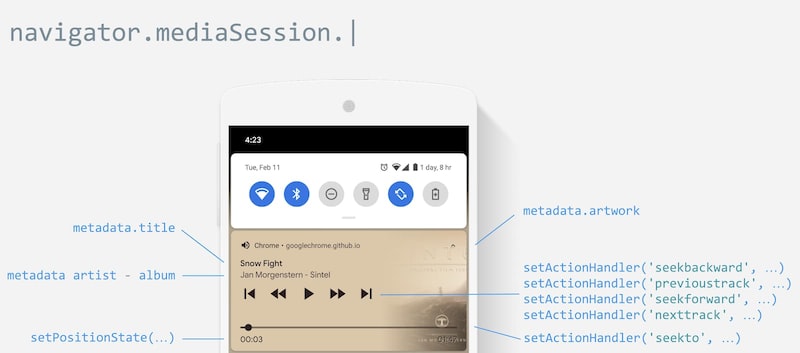
The example below shows you how to create a custom media notification and respond to basic media actions such as play and pause.
const video = document.querySelector("video");
navigator.mediaSession.metadata = new MediaMetadata({
title: "Never Gonna Give You Up",
artist: "Rick Astley",
album: "Whenever You Need Somebody",
artwork: [
{ src: "https://via.placeholder.com/96", sizes: "96x96" },
{ src: "https://via.placeholder.com/128", sizes: "128x128" },
{ src: "https://via.placeholder.com/256", sizes: "256x256" },
{ src: "https://via.placeholder.com/512", sizes: "512x512" },
],
});
navigator.mediaSession.setActionHandler("play", async () => {
// Resume playback
try {
await video.play();
} catch (err) {
console.error(err.name, err.message);
}
});
navigator.mediaSession.setActionHandler("pause", () => {
// Pause active playback
video.pause();
});
video.addEventListener("play", () => {
navigator.mediaSession.playbackState = "playing";
});
video.addEventListener("pause", () => {
navigator.mediaSession.playbackState = "paused";
});
Further reading
- W3C Media Session Specification
- Customize media notifications and playback controls with the Media Session API
- MDN Media Session API
Demo
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<link
rel="icon"
href="data:image/svg+xml,<svg xmlns=%22http://www.w3.org/2000/svg%22 viewBox=%220 0 100 100%22><text y=%22.9em%22 font-size=%2290%22>📺</text></svg>"
/>
<title>How to customize media notifications</title>
</head>
<body>
<h1>How to customize media notifications</h1>
<video controls playsinline src="https://storage.googleapis.com/media-session/caminandes/short.mp4"></video>
<p>Credits: Media files are © copyright Blender Foundation | <a href="https://www.blender.org">www.blender.org</a>.</p>
</body>
</html>
CSS
:root {
color-scheme: dark light;
}
html {
box-sizing: border-box;
}
*,
*:before,
*:after {
box-sizing: inherit;
}
body {
margin: 1rem;
font-family: system-ui, sans-serif;
}
video {
display: block;
margin-top: 10px;
max-width: 100%;
}
JS
const video = document.querySelector("video");
navigator.mediaSession.metadata = new MediaMetadata({
title: "Caminandes 2: Gran Dillama - Blender Animated Short",
artist: "Blender Foundation",
artwork: [
{ src: "https://storage.googleapis.com/media-session/caminandes/artwork-96.png", sizes: "96x96" },
{ src: "https://storage.googleapis.com/media-session/caminandes/artwork-128.png", sizes: "128x128" },
{ src: "https://storage.googleapis.com/media-session/caminandes/artwork-256.png", sizes: "256x256" },
{ src: "https://storage.googleapis.com/media-session/caminandes/artwork-512.png", sizes: "512x512" },
],
});
navigator.mediaSession.setActionHandler("play", async () => {
// Resume playback
try {
await video.play();
} catch (err) {
console.error(err.name, err.message);
}
});
navigator.mediaSession.setActionHandler("pause", () => {
// Pause active playback
video.pause();
});
video.addEventListener("play", () => {
navigator.mediaSession.playbackState = "playing";
});
video.addEventListener("pause", () => {
navigator.mediaSession.playbackState = "paused";
});