في حين أنّ معظم ميزات الذكاء الاصطناعي على الويب تعتمد على الخوادم، يتم تشغيل الذكاء الاصطناعي من جهة العميل مباشرةً في متصفّح المستخدم. ويقدّم ذلك العديد من المزايا، بما في ذلك وقت الاستجابة المنخفض، وانخفاض التكاليف من جهة الخادم، وعدم الحاجة إلى مفتاح واجهة برمجة التطبيقات، وزيادة خصوصية المستخدمين، واستخدام التطبيقات بلا إنترنت. يمكنك تنفيذ تقنيات الذكاء الاصطناعي من جهة العميل التي تعمل على جميع المتصفّحات باستخدام مكتبات JavaScript، مثل TensorFlow.js وTransformers.js وMediaPipe GenAI.
تؤدي تكنولوجيات الذكاء الاصطناعي من جهة العميل أيضًا إلى ظهور تحديات في الأداء: على المستخدمين تنزيل المزيد من الملفات، وعلى المتصفّح العمل بجهد أكبر. لكي تعمل هذه الميزة بشكل جيد، ضع في اعتبارك ما يلي:
- حالة الاستخدام: هل الذكاء الاصطناعي من جهة العميل هو الخيار المناسب لميزتك؟ هل يندرج العنصر ضمن رحلة مستخدِم مهمة؟ وإذا كان الأمر كذلك، هل لديك عنصر احتياطي؟
- الممارسات الجيدة لتنزيل النماذج واستخدامها يُرجى متابعة القراءة لمعرفة المزيد من المعلومات.
قبل تنزيل النموذج
مكتبة "العقل" وحجم النموذج
لتنفيذ الذكاء الاصطناعي من جهة العميل، ستحتاج إلى نموذج وعادةً ما تكون مكتبة. عند اختيار المكتبة، قيِّم حجمها كما تفعل مع أي أداة أخرى.
يُرجى مراعاة حجم النموذج أيضًا. يعتمد ما يُعدّ كبيرًا لنموذج الذكاء الاصطناعي على ما يلي: يمكن أن تكون 5 ميغابايت قاعدة أساسية مفيدة: وهي أيضًا الشريحة المئوية الخامسة والسبعين من متوسط حجم صفحة الويب. يمكن استخدام رقم أقل 10 ميغابايت.
في ما يلي بعض النقاط المهمة التي يجب مراعاتها بشأن حجم النموذج:
- يمكن أن تكون العديد من نماذج الذكاء الاصطناعي المخصّصة للمهام صغيرة جدًا. إنّ نموذجًا مثل BudouX، الذي يُستخدَم لفصل الأحرف بدقة في اللغات الآسيوية، لا يتجاوز حجمه 9.4 كيلوبايت بعد ضغطه بتنسيق GZipped. حجم نموذج التعرّف على اللغة في MediaPipe هو 315 كيلوبايت.
- حتى نماذج الرؤية يمكن أن تكون بحجم معقول. يبلغ حجم نموذج وضع اليد وجميع الموارد ذات الصلة 13.4 ميغابايت. على الرغم من أنّ هذا الحجم أكبر بكثير من معظم حِزم الواجهة الأمامية المُكثَّفة، إلا أنّه يُقارَن بمتوسط حجم صفحة الويب، وهو 2.2 ميغابايت (2.6 ميغابايت على أجهزة الكمبيوتر المكتبي).
- يمكن أن تتجاوز نماذج الذكاء الاصطناعي التوليدي الحجم المُقترَح لموارد الويب. تبلغ مساحة DistilBERT، التي تُعدّ إما نموذجًا صغيرًا جدًا للمعالجة اللغوية الآلية أو نموذجًا بسيطًا للمعالجة اللغوية الآلية (تختلف الآراء)، 67 ميغابايت. حتى النماذج اللغوية الكبيرة الحجم، مثل Gemma 2B، يمكن أن تصل إلى 1.3 غيغابايت. وهذا يتجاوز حجم متوسط صفحة الويب بأكثر من 100 مرة.
يمكنك تقييم حجم التنزيل الدقيق للنماذج التي تخطّط لاستخدامها باستخدام أدوات المطوّرين في متصفّحاتك.


تحسين حجم النموذج
- مقارنة جودة النماذج وأحجام التنزيل قد يكون للنموذج الأصغر حجمًا دقة كافية لحالة الاستخدام، مع أنّه أصغر بكثير. تتوفر أساليب تصغير النماذج والتحسين الدقيق بهدف تقليل حجم النموذج بشكل كبير مع الحفاظ على دقة كافية.
- اختَر نماذج مخصّصة متى أمكن. وتكون النماذج المصمّمة خصيصًا لهدف معيّن أصغر حجمًا. على سبيل المثال، إذا كنت تريد تنفيذ مهام محدّدة، مثل تحليل المشاعر أو السمية، استخدِم نماذج متخصّصة في هذه المهام بدلاً من نموذج لغوي كبير عام.
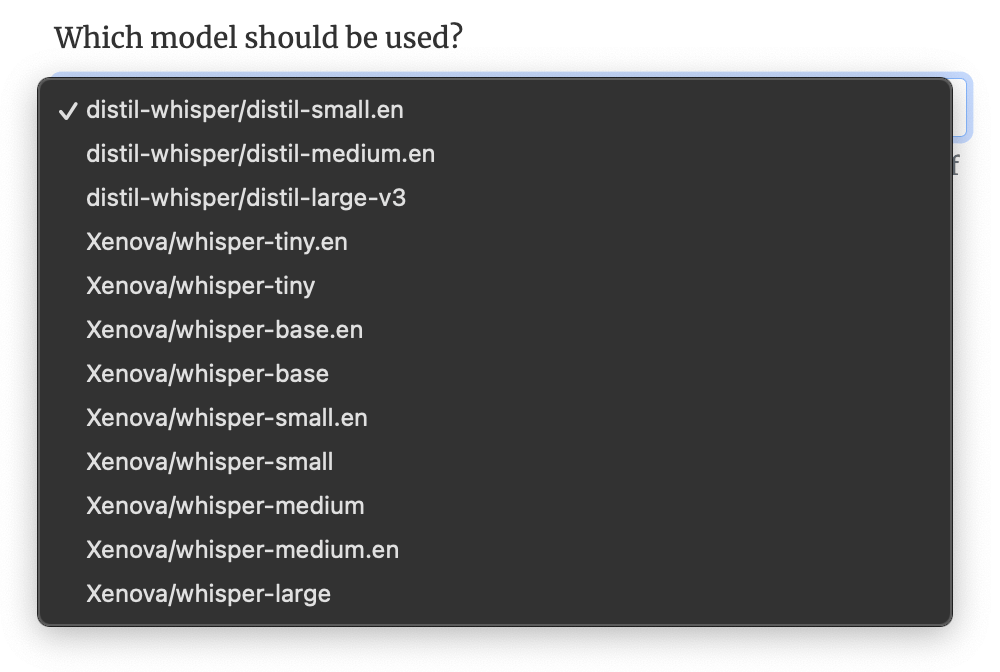
على الرغم من أنّ جميع هذه النماذج تؤدي المهمة نفسها بدقة متفاوتة، إلا أنّ أحجامها تختلف اختلافًا كبيرًا: من 3 ميغابايت إلى 1.5 غيغابايت.
التحقّق من إمكانية تشغيل النموذج
لا يمكن تشغيل نماذج الذكاء الاصطناعي على بعض الأجهزة. حتى الأجهزة التي تتضمّن مواصفات مناسبة للأجهزة قد تواجه مشاكل، إذا كانت هناك عمليات أخرى باهظة التكلفة قيد التشغيل أو يتم بدء تشغيلها أثناء استخدام النموذج.
إلى أن يتوفّر حلّ، إليك ما يمكنك فعله الآن:
- التحقّق من توفّر WebGPU تستخدم WebGPU العديد من مكتبات الذكاء الاصطناعي من جهة العميل، بما في ذلك الإصدار 3 من Transformers.js و MediaPipe. في الوقت الحالي، لا تلجأ بعض هذه المكتبات تلقائيًا إلى استخدام Wasm إذا لم يكن WebGPU متوافقًا. يمكنك تخفيف ذلك من خلال تضمين الرمز البرمجي المرتبط بالذكاء الاصطناعي في فحص لرصد ميزات WebGPU، إذا كنت تعلم أنّ مكتبة الذكاء الاصطناعي من جهة العميل تحتاج إلى WebGPU.
- استبعاد الأجهزة ذات الأداء المنخفض استخدِم Navigator.hardwareConcurrency، Navigator.deviceMemory وCompute Pressure API لتقدير قدرات الجهاز ومستوى الضغط. لا تتوفّر واجهات برمجة التطبيقات هذه في جميع المتصفّحات وهي غير دقيقة عمدًا لمنع وضع بصمة الجهاز، ولكن يمكن أن تساعد في استبعاد الأجهزة التي تبدو ضعيفة جدًا.
الإشارة إلى عمليات التنزيل الكبيرة
بالنسبة إلى النماذج الكبيرة، يجب تحذير المستخدمين قبل التنزيل. من المرجّح أن يقبل مستخدمو أجهزة الكمبيوتر المكتبي تنزيل الملفات الكبيرة أكثر من مستخدمي الأجهزة الجوّالة. لرصد الأجهزة الجوّالة، استخدِم mobile
من User-Agent Client Hints API (أو سلسلة User-Agent إذا كانت User-Agent Client Hints API
غير متوافقة).
الحد من عمليات التنزيل الكبيرة
- تنزيل المحتوى الضروري فقط خاصةً إذا كان النموذج كبيرًا، لا تنزله إلا بعد التأكّد بشكل معقول من أنّه سيتم استخدام ميزات الذكاء الاصطناعي. على سبيل المثال، إذا كانت لديك ميزة اقتراحات ذكاء اصطناعي (AI) للكتابة التلقائية، لا يتم تنزيل البيانات إلا عندما يبدأ المستخدم في استخدام ميزات الكتابة.
- تخزين النموذج مؤقتًا بشكل صريح على الجهاز باستخدام Cache API، لتجنُّب تنزيله في كل زيارة لا تعتمد فقط على ذاكرة التخزين المؤقت الضمنية لبروتوكول HTTP المتصفّح.
- تقسيم عملية تنزيل النموذج إلى أجزاء: تؤدي دالة fetch-in-chunks إلى تقسيم عملية تنزيل كبيرة إلى أجزاء أصغر.
تنزيل النموذج وإعداده
عدم حظر المستخدم
إعطاء الأولوية لتجربة المستخدم السلسة: السماح بتشغيل الميزات الرئيسية حتى إذا لم يتم تحميل نموذج الذكاء الاصطناعي بالكامل بعد
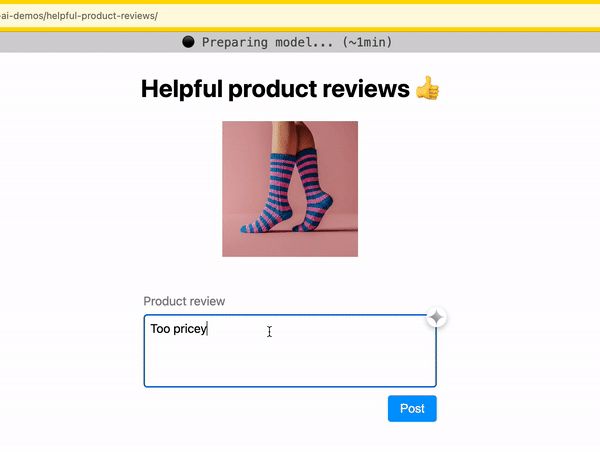
الإشارة إلى مستوى التقدّم
أثناء تنزيل النموذج، يمكنك الإشارة إلى مستوى التقدّم الذي تمّه والوقت المتبقّي.
- إذا كانت مكتبة الذكاء الاصطناعي من جهة العميل هي التي تعالج عمليات تنزيل النماذج، استخدِم حالة تقدّم التنزيل لعرضها على المستخدم. إذا لم تكن هذه الميزة متوفرة، ننصحك بفتح مشكلة لطلبها (أو المساهمة بها).
- إذا كنت تتعامل مع عمليات تنزيل النماذج في الرمز البرمجي الخاص بك، يمكنك جلب النموذج في
أجزاء باستخدام مكتبة، مثل
fetch-in-chunks،
وعرض مستوى تقدّم التنزيل للمستخدم.
- للحصول على مزيد من النصائح، يُرجى الاطّلاع على مقالتَي أفضل الممارسات المتعلّقة بمؤشرات التقدّم المتحرّكة والتصميم المناسب للانتظار الطويل والمقاطعات.
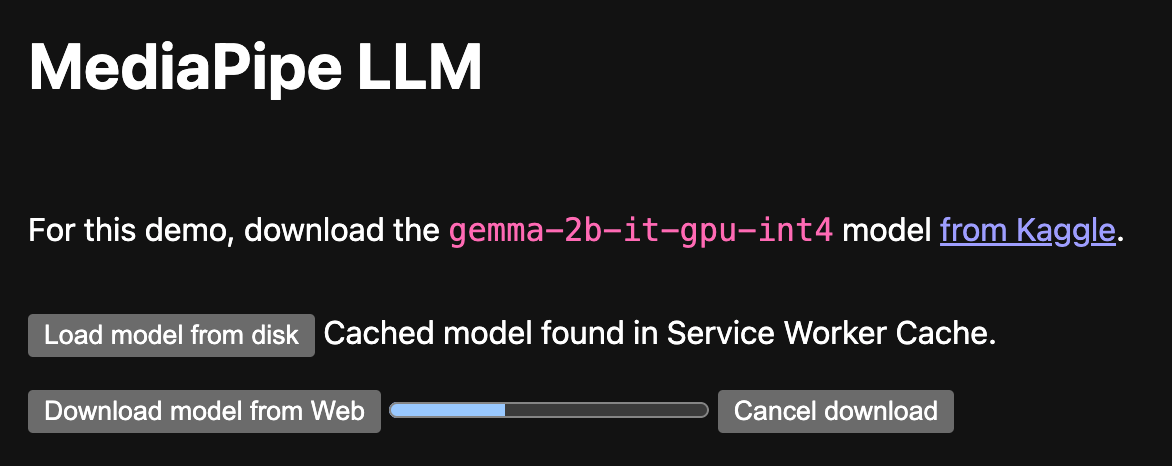
التعامل مع انقطاعات الشبكة بسلاسة
يمكن أن تستغرق عمليات تنزيل النماذج فترات زمنية مختلفة، حسب حجمها. ضع في اعتبارك كيفية التعامل مع انقطاعات الشبكة في حال انقطاع الاتصال بالإنترنت لدى المستخدم. عند الإمكان، أطلِع المستخدم على انقطاع الاتصال، واستئنِف التنزيل عند استعادة الاتصال.
يشكّل الاتصال غير المستقر سببًا آخر لتنزيل المحتوى على أجزاء.
تفريغ المهام المستهلكة للطاقة إلى عامل ويب
يمكن أن تؤدي المهام المستهلكة للوقت، مثل خطوات إعداد النموذج بعد التنزيل، إلى حظر سلسلة المحادثات الرئيسية، ما يتسبب في تجربة استخدام متقطّعة. ويساعد نقل هذه المهام إلى عامل ويب.
يمكنك العثور على عرض توضيحي وتنفيذ كامل استنادًا إلى مشغّل ويب:
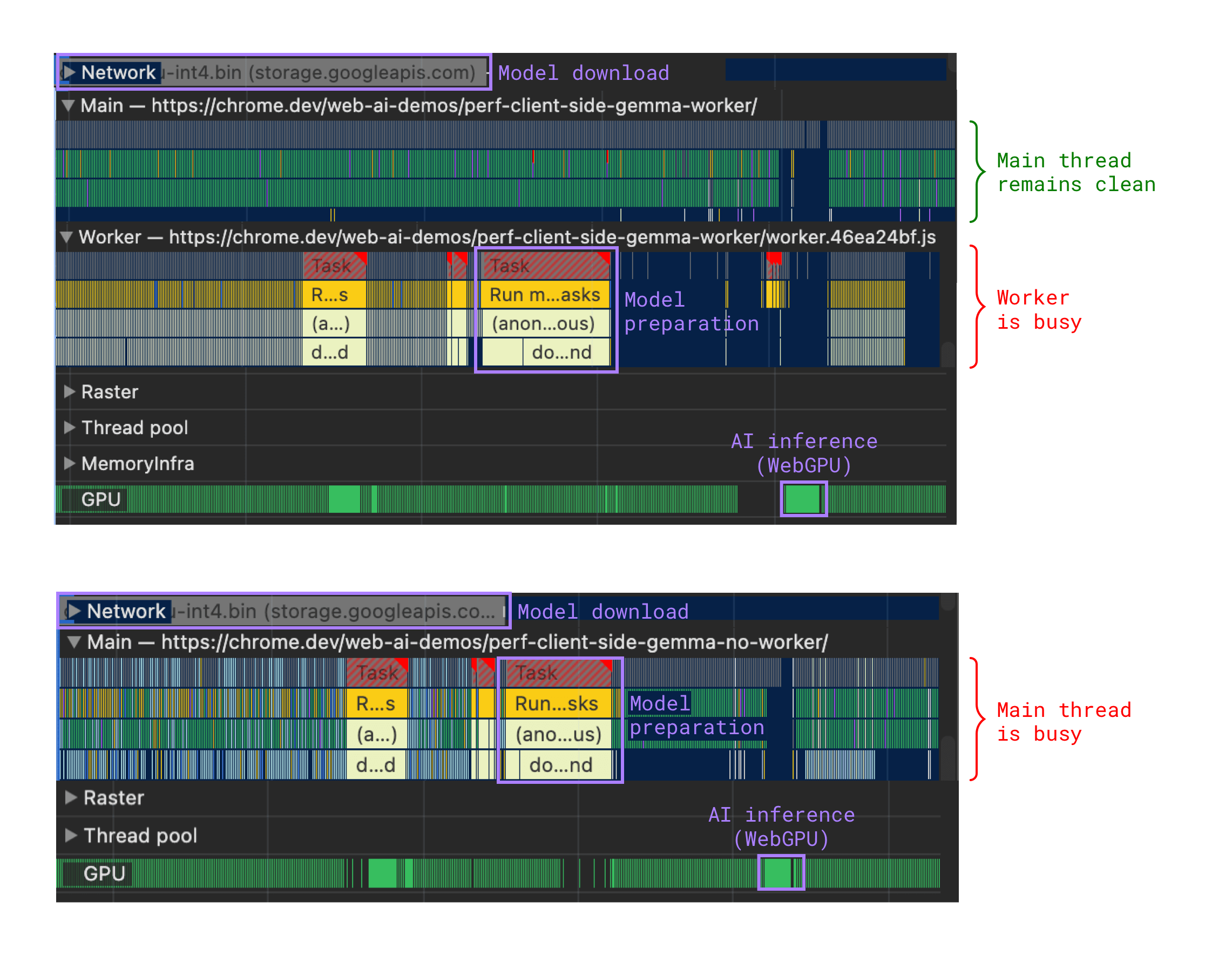
أثناء الاستنتاج
بعد تنزيل النموذج واستعداده، يمكنك إجراء الاستنتاج. يمكن أن تكون الاستنتاجات مكلّفة من الناحية الحسابية.
نقل الاستنتاج إلى عامل ويب
إذا حدثت الاستنتاجات من خلال WebGL أو WebGPU أو WebNN، تعتمد على وحدة معالجة الرسومات. ويعني ذلك أنّه يحدث في عملية منفصلة لا تحظر واجهة المستخدم.
أمّا بالنسبة إلى عمليات التنفيذ المستندة إلى وحدة المعالجة المركزية (مثل Wasm، الذي يمكن أن يكون بديلاً لسمة WebGPU، إذا لم تكن WebGPU متوافقة)، فيؤدي نقل الاستنتاج إلى مشغّل ويب إلى إبقاء صفحتك سريعة الاستجابة، تمامًا كما هو الحال أثناء إعداد النموذج.
قد يكون التنفيذ أبسط إذا كان كل الرمز برمجي المرتبط بالذكاء الاصطناعي (جلب النموذج، وإعداد النموذج، والاستنتاج) متوفّرًا في المكان نفسه. وبالتالي، يمكنك اختيار web worker، سواء كانت وحدة معالجة الرسومات قيد الاستخدام أم لا.
معالجة الأخطاء
على الرغم من أنّك تأكّدت من أنّه من المفترض أن يعمل النموذج على الجهاز، قد يشغّل المستخدم عملية أخرى تستهلك الموارد بشكل كبير لاحقًا. للحدّ من هذا:
- معالجة أخطاء الاستنتاج احط الاستنتاج في وحدات
try
/catch
، وعالج أخطاء وقت التشغيل المقابلة. - معالجة أخطاء WebGPU، سواء كانت غير متوقّعة أو GPUDevice.lost، التي تحدث عند إعادة ضبط وحدة معالجة الرسومات بسبب مواجهة الجهاز لمشاكل.
الإشارة إلى حالة الاستنتاج
إذا استغرقت الاستنتاج وقتًا أطول مما قد يبدو عليه بشكل فوري، أشر إلى المستخدم بأنّ النموذج يفكر. استخدِم الرسومات المتحرّكة لتسهيل فترة الانتظار وإقناع المستخدم بأنّ التطبيق يعمل على النحو المطلوب.
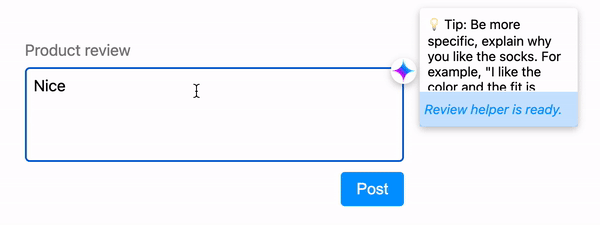
جعل الاستنتاج قابلاً للإلغاء
اسمح للمستخدم بتحسين طلب البحث أثناء التشغيل، بدون أن يهدر النظام موارده في إنشاء رد لن يراه المستخدم أبدًا.