Cómo Next.js acelera las navegaciones con la carga previa de rutas y cómo personalizarla
¿Qué aprenderás?
En esta publicación, aprenderás cómo funciona el enrutamiento en Next.js, cómo se optimiza para la velocidad y cómo personalizarlo para que se adapte mejor a tus necesidades.
El componente <Link>
En Next.js, no es necesario configurar el enrutamiento de forma manual.
Next.js usa enrutamiento basado en el sistema de archivos, lo que te permite crear archivos y carpetas dentro del directorio ./pages/
:
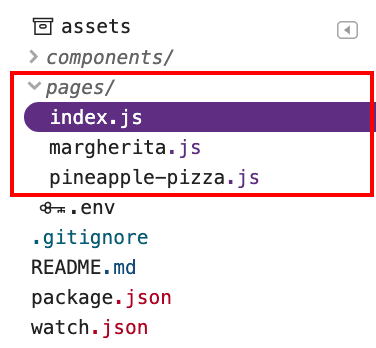
Para vincular a diferentes páginas, usa el componente <Link>
, de manera similar a como usarías el elemento <a>
:
<Link href="/margherita">
<a>Margherita</a>
</Link>
Cuando usas el componente <Link>
para la navegación, Next.js hace un poco más por ti. Por lo general, se descarga una página cuando sigues un vínculo a ella, pero
Next.js precarga automáticamente el código JavaScript necesario para renderizar la página.
Cuando cargas una página con algunos vínculos, es probable que, cuando sigas un vínculo, el componente detrás de él ya se haya recuperado. Esto mejora la capacidad de respuesta de la aplicación, ya que permite que las navegaciones a páginas nuevas sean más rápidas.
En la siguiente app de ejemplo, la página index.js
vincula a margherita.js
con un <Link>
:
Usa las Herramientas para desarrolladores de Chrome para verificar que margherita.js
se haya recuperado previamente:
1. Para obtener una vista previa del sitio, presiona Ver app. Luego, presiona Pantalla completa
.
- Presiona "Control + Mayúsculas + J" (o "Comando + Opción + J" en Mac) para abrir DevTools.
Haga clic en la pestaña Red.
Selecciona la casilla de verificación Inhabilitar la caché.
Vuelve a cargar la página.
Cuando cargas index.js
, la pestaña Red muestra que también se descargó margherita.js
:
Cómo funciona la precarga automática
Next.js precarga solo los vínculos que aparecen en el viewport y usa la API de Intersection Observer para detectarlos. También inhabilita la precarga cuando la conexión de red es lenta o cuando los usuarios activan Save-Data
. En función de estas verificaciones, Next.js inserta de forma dinámica etiquetas <link
rel="preload">
para descargar componentes para navegaciones posteriores.
Next.js solo recupera el código JavaScript, no lo ejecuta. De esa manera, no se descarga ningún contenido adicional que la página recuperada previamente pueda solicitar hasta que visites el vínculo.
Evita la carga previa innecesaria
Para evitar descargar contenido innecesario, puedes inhabilitar la carga previa de páginas que se visitan con poca frecuencia. Para ello, establece la propiedad prefetch
en <Link>
como false
:
<Link href="/pineapple-pizza" prefetch={false}>
<a>Pineapple pizza</a>
</Link>
En esta segunda app de ejemplo, la página index.js
tiene un <Link>
a pineapple-pizza.js
con prefetch
configurado como false
:
Para inspeccionar la actividad de red, sigue los pasos del primer ejemplo. Cuando cargas index.js
, la pestaña Red de DevTools muestra que se descargó margherita.js
, pero no pineapple-pizza.js
:
Precarga con enrutamiento personalizado
El componente <Link>
es adecuado para la mayoría de los casos de uso, pero también puedes compilar tu propio componente para realizar el enrutamiento. Next.js te facilita esto con la API del router disponible en next/router
.
Si quieres hacer algo (por ejemplo, enviar un formulario) antes de navegar a una ruta nueva, puedes definirlo en tu código de enrutamiento personalizado.
Cuando usas componentes personalizados para el enrutamiento, también puedes agregar la carga previa.
Para implementar la precarga en tu código de enrutamiento, usa el método prefetch
de useRouter
.
Observa components/MyLink.js
en esta app de ejemplo:
La precarga se realiza dentro del hook useEffect
. Si la propiedad prefetch
en un <MyLink>
se establece en true
, la ruta especificada en la propiedad href
se precargará cuando se renderice ese <MyLink>
:
useEffect(() => {
if (prefetch) router.prefetch(href)
});
Cuando haces clic en el vínculo, el enrutamiento se realiza en handleClick
. Se registra un mensaje en la consola, y el método push
navega a la nueva ruta especificada en href
:
const handleClick = e => {
e.preventDefault();
console.log("Having fun with Next.js.");
router.push(href);
};
En esta app de ejemplo, la página index.js
tiene un <MyLink>
a margherita.js
y pineapple-pizza.js
. La propiedad prefetch
se establece en true
en /margherita
y en false
en /pineapple-pizza
.
<MyLink href="/margherita" title="Margherita" prefetch={true} />
<MyLink href="/pineapple-pizza" title="Pineapple pizza" prefetch={false} />
Cuando cargas index.js
, la pestaña Red muestra que margherita.js
se descargó y pineapple-pizza.js
no:
Cuando haces clic en cualquiera de los vínculos, Console registra "Having fun with Next.js" y navega a la nueva ruta:
Conclusión
Cuando usas <Link>
, Next.js precarga automáticamente el código JavaScript necesario para renderizar la página vinculada, lo que hace que la navegación a páginas nuevas sea más rápida. Si usas enrutamiento personalizado, puedes usar la API del router de Next.js para implementar la carga previa por tu cuenta. Inhabilita la carga previa para las páginas que se visitan con poca frecuencia y evita descargar contenido innecesariamente.