Analiza statyczna to typ testowania, który umożliwia automatyczne sprawdzanie bez uruchamiania go i pisania automatycznego testu. Masz pewnie już widziałem(-am) takie testy, jeśli używasz IDE takiego jak VSCode. sprawdzanie wykonywane przez TypeScript jest rodzajem analizy statycznej i może pokazać, w postaci falistych linii pod błędami lub ostrzeżeniami.
ESLint
ESLint to narzędzie przekazujące informacje o potencjalnych problemach w bazy kodu. Te problemy mogą być bezpieczne,ale występują błędy lub niestandardowe zachowanie w swoich własnych prawach. ESLint pozwala zastosować szereg reguł, które są sprawdzane w bazie kodu, w tym wiele elementów w „zalecanych” ustawiony.
Dobrym przykładem reguły ESLint jest:
no-unsafe-finally
. Uniemożliwi to pisanie oświadczeń, które modyfikują
sterować przepływem w obrębie bloku finally
. To świetna zasada, bo w ten sposób
to nietypowy sposób napisania JavaScriptu, który może być trudny do śledzenia. Jest to jednak
a także coś, co powinien być w stanie wykryć dzięki prawidłowemu procesowi weryfikacji kodu.
try {
const result = await complexFetchFromNetwork();
if (!result.ok) {
throw new Error("failed to fetch");
}
} finally {
// warning - this will 'overrule' the previous exception!
return false;
}
W związku z tym ESLint nie zastępuje sprawnego procesu weryfikacji (oraz stylu w przewodniku określającym, jak powinna wyglądać baza kodu), ponieważ nie będzie aby uchwycić wszystkie nieortodoksyjne podejście, jakie deweloper może próbować wprowadzić w bazę kodu. Przewodnik Google dotyczący praktycznych ćwiczeń w języku angielskim zawiera krótką sekcję „Prostota”.
ESLint pozwala złamać regułę i oznaczyć kod jako „dozwolony”. Na przykład: może zezwalać na poprzednią logikę, dodając do niej adnotacje w taki sposób:
finally {
// eslint-disable-next-line no-unsafe-finally
return false;
}
Jeśli stale łamiesz jakąś regułę, rozważ jej wyłączenie. Te narzędzia zachęcają do pisania kodu w określony sposób, ale Twój zespół może być używany na pisanie kodu w inny sposób i zdawać sobie sprawę z zagrożeń, jak ważna jest pokora.
Natomiast włączenie narzędzi do analizy statycznej na dużej bazie kodu nieprzydatnego szumu (i pracy nad refaktoryzacją) w kodzie, który w innym przypadku działał w porządku. Łatwiej jest to zrobić na wczesnym etapie cyklu życia projektu.
Wtyczki ESLint do obsługi przeglądarki
Możesz dodać do ESLint wtyczkę, która sygnalizuje użycie interfejsów API, które nie są zbyt popularne lub nie są obsługiwane przez Twoją listę docelowych przeglądarek. Zgodność z wtyczką-eslint pakiet może ostrzegać, gdy interfejs API może być niedostępny dla użytkowników, więc Dzięki temu nie muszą ciągle śledzić swojej pracy.
Sprawdzanie typu na potrzeby analizy statycznej
Podczas nauki języka JavaScript nowi deweloperzy zwykle poznają ideę że jego język jest słabo wpisany. Oznacza to, że można zadeklarować jako jednego typu, a potem użyć tej samej lokalizacji w inny sposób. Jest to podobne do Pythona i innych języków skryptowych, ale w przeciwieństwie kompilowanych języków, takich jak C/C++ i Rust.
Ten język może być dobry na początek.
jest to prostota, dzięki której JavaScript stał się tak popularny. Jednak często jest to punkt porażki
dla niektórych baz kodu, a przynajmniej w sposób, który pozwala na błędy
mają miejsce. Na przykład przekazując number
, gdzie string
lub typ obiektu
oczekiwano, że nieprawidłowo wpisana wartość może zostać rozpowszechniona w różnych
przed wywołaniem dezorientującego TypeError
.
TypeScript
TypeScript to najbardziej popularne rozwiązanie tego, że JavaScript nie wymaga pisania i informacjami o nich. Jest on intensywnie wykorzystywany na tym kursie. To nie jest szkolenie na temat może być istotnym elementem zestawu narzędzi, ponieważ zapewnia analizę statyczną.
Krótki przykład to kod, który oczekuje wywołania zwrotnego akceptującego
Imię i nazwisko użytkownika string
, wiek: number
const callback = (name: string, age: string): void => {
console.info(name, 'is now', age, 'years old!');
};
onBirthday(callback);
Generuje ten błąd po wykonaniu skryptu TypeScript lub nawet po najechaniu kursorem w IDE:
bad.ts:4:12 - error TS2345: Argument of type '(name: string, age: string) => void' is not assignable to parameter of type '(name: string, age: number) => void'.
Types of parameters 'age' and 'age' are incompatible.
Type 'number' is not assignable to type 'string'.
4 onBirthday(callback);
~~~~~~~~
Found 1 error in bad.ts:4
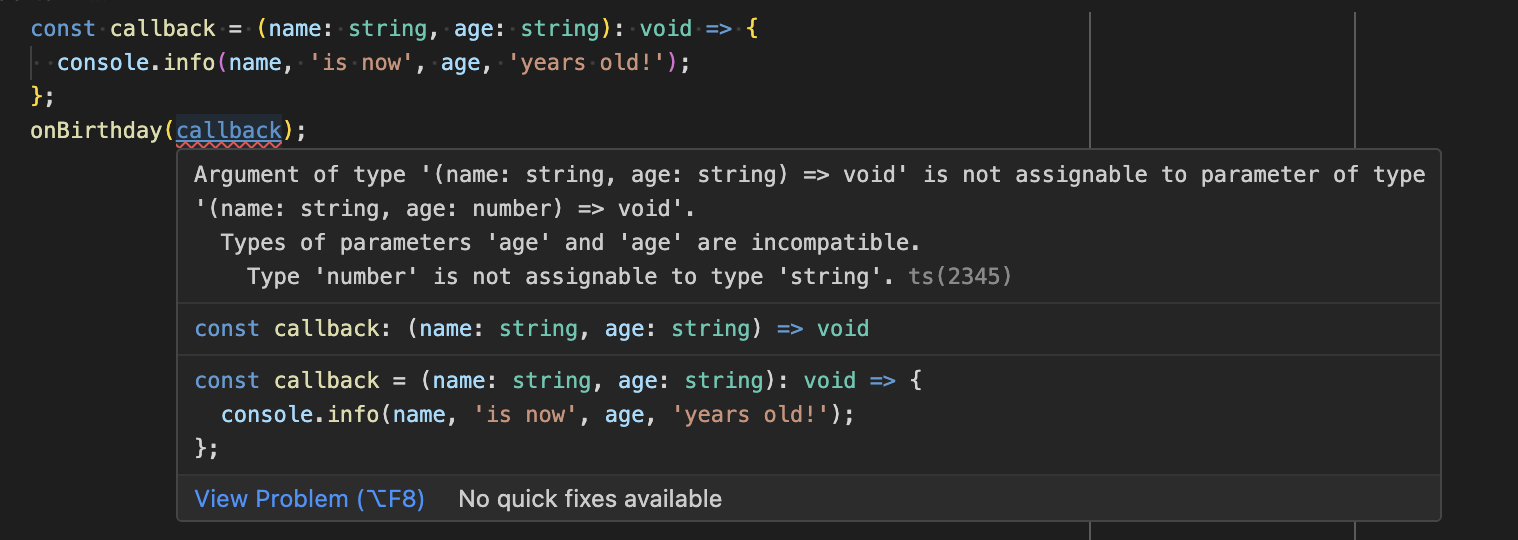
Ostatecznie celem użycia TypeScript jest zapobieganie takim błędom:
wiek powinien być wartością number
, a nie string
– wkradł się do Twojego projektu. Ten
ale mogą być trudne do wykrycia za pomocą innych rodzajów testów.
Dodatkowo system typów może udzielać opinii jeszcze przed napisaniem testu.
Może to ułatwić proces pisania kodu przez przekazanie opinii na wczesnym etapie.
o błędach typu w trakcie programowania, a nie o tym,
w końcu działa.
Największym wyzwaniem podczas korzystania z TypeScriptu jest jego prawidłowa konfiguracja. Co
projekt wymaga pliku tsconfig.json
, który jest używany głównie przez platformę tsc
narzędzia wiersza poleceń, jest również odczytywane przez IDE, takie jak VSCode,
narzędzia i narzędzia, takie jak Vitest. Ten plik zawiera setki
opcje i flagi. Przydatne materiały dotyczące ich konfiguracji znajdziesz tutaj:
- Co to jest plik tsconfig.json
- Scentralizowane rekomendacje dotyczące baz TypeScript
- Sprawdzanie pliku JS za pomocą narzędzia TS
Ogólne wskazówki dotyczące TypeScript
Podczas konfigurowania i używania skryptu TypeScript za pomocą pliku tsconfig.json
zachowaj
o których warto pamiętać:
- Upewnij się, że pliki źródłowe są rzeczywiście uwzględnione i sprawdzone. Jeśli plik „nie zawiera żadnych błędów”, prawdopodobnie dlatego, że nie jest sprawdzana.
- Bezpośrednie opis typów i interfejsów w plikach
.d.ts
, a nie niejawne ich opisanie podczas pisania funkcji może sprawić, co ułatwia testowanie bazy kodu. Łatwiej wymyślać „fałszywe” i „fałszywe” wersje gdy interfejsy są przejrzyste. .
TypeScript domyślnie dowolnego
Jedną z najwydajniejszych i najbardziej atrakcyjnych opcji konfiguracji TypeScript jest
flaga noImplicitAny
. Często jednak najtrudniejsze jest jej włączenie,
zwłaszcza jeśli masz już dużą bazę kodu. (Flaga noImplicitAny
to
włączona domyślnie, jeśli jesteś w trybie strict
. W przeciwnym razie nie będzie).
Ta flaga spowoduje, że funkcja zwróci błąd:
export function fibonacci(n) {
if (n <= 1) {
return 0;
} else if (n === 2) {
return 1;
}
return fibonacci(n - 1) + fibonacci(n - 2);
}
Chociaż dla czytelnika jest dość jasne, że n
musi być liczbą,
TypeScript nie może tego jednoznacznie potwierdzić. Jeśli używasz VSCode, najedź kursorem na
funkcja opisała go w ten sposób:
function fibonacci(n: any): any
Wywołujący tę funkcję będzie mógł przekazać wartość typu any
(typ, który dopuszcza dowolny inny typ), nie tylko number
. Włączając
noImplicitAny
, możesz zabezpieczyć ten rodzaj kodu podczas programowania,
bez konieczności pisania obszernych testów logiki biznesowej dla przekazywania kodu
nieprawidłowe typy danych w konkretnych miejscach.
Prostym rozwiązaniem jest oznaczenie argumentu n
i typu zwracanego przez fibonacci
jako number
.
Flaga noImplicitAny
nie uniemożliwia jednoznacznego pisania any
w
w bazie kodu. Nadal możesz napisać funkcję, która przyjmuje lub zwraca
typu any
. Zapewnia to tylko przypisanie typu każdej zmiennej.