試用新的 Lighthouse API,評估整個使用者流程中的效能和最佳做法。
Lighthouse 是一項實用的工具,可在初始網頁載入期間測試效能和最佳做法。不過,以往很難使用 Lighthouse 分析網頁生命週期的其他面向,例如:
- 使用快取的網頁載入作業
- 已啟用 Service Worker 的頁面
- 將潛在的使用者互動納入考量
這表示 Lighthouse 可能會遺漏重要資訊。網站體驗核心指標是根據所有網頁載入作業計算,不只限於快取資料為空的網頁。此外,您也可以在網頁開啟的整個期間,評估累計版面配置位移 (CLS) 等指標。
Lighthouse 提供新的使用者流程 API,可在網頁生命週期中的任何時間點進行實驗室測試。Puppeteer 可用於指令碼載入網頁及觸發合成的使用者互動,並透過多種方式叫用 Lighthouse,在互動期間取得關鍵深入分析資料。也就是說,您可以在網頁載入期間和與網頁互動期間評估成效。無障礙功能檢查可以在 CI 中執行,不只是在初始檢視畫面上,也可在結帳流程中深入檢查,確保不會發生迴歸問題。
幾乎所有為了確保有效使用者流程而編寫的 Puppeteer 指令碼,現在都能在任何時間點插入 Lighthouse,以便評估成效和最佳做法。本教學課程將逐步介紹新的 Lighthouse 模式,可評估使用者流程的不同部分:導覽、快照和時間範圍。
設定
使用者流程 API 目前仍處於預覽階段,但 Lighthouse 現已提供這項功能。如要試用下方的示範內容,您必須使用 Node 14 以上版本。建立空白目錄並執行以下指令:
# Default to ES modules.
echo '{"type": "module"}' > package.json
# Init npm project without the wizard.
npm init -y
# Dependencies for these examples.
npm install lighthouse puppeteer open
導覽
新的 Lighthouse「導覽」模式實際上是命名 (截至目前) 標準 Lighthouse 行為:分析網頁的冷負載。這是用於監控網頁載入效能的模式,但使用者流程也可能會帶來新的洞察資料。
若要編寫 Lighthouse 擷取網頁負載的指令碼:
- 使用 puppeteer 開啟瀏覽器。
- 開始 Lighthouse 使用者流程。
- 前往目標網址。
import fs from 'fs';
import open from 'open';
import puppeteer from 'puppeteer';
import {startFlow} from 'lighthouse/lighthouse-core/fraggle-rock/api.js';
async function captureReport() {
const browser = await puppeteer.launch({headless: false});
const page = await browser.newPage();
const flow = await startFlow(page, {name: 'Single Navigation'});
await flow.navigate('https://web.dev/performance-scoring/');
await browser.close();
const report = await flow.generateReport();
fs.writeFileSync('flow.report.html', report);
open('flow.report.html', {wait: false});
}
captureReport();
這是最簡單的流程。開啟報表後,系統會顯示摘要檢視畫面,其中只包含單一步驟。按一下該步驟,即可查看該導覽的傳統 Lighthouse 報表。
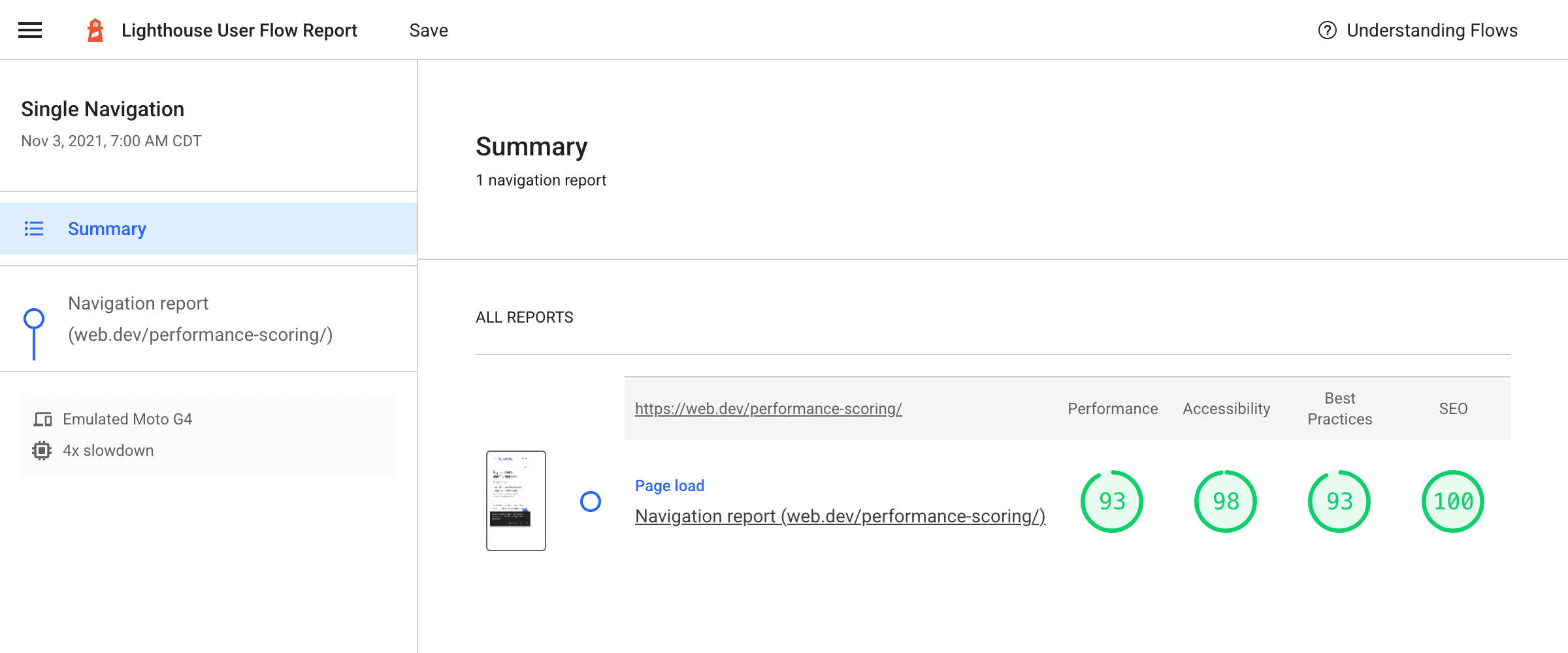
如同 Lighthouse 的一般做法,這個頁面會先清除任何快取或本機儲存空間,再進行載入作業,但實際造訪網站的使用者會同時產生冷快取和溫快取,因此在這種冷載入作業與使用者返回頁面時,仍保留溫快取的情況下,效能可能會出現大幅差異。
擷取暖負載
您也可以在這個指令碼中加入第二個導覽,這次請停用 Lighthouse 在導覽中預設會清除的快取和儲存空間。下一個範例會載入 web.dev 上的文章,看看快取功能帶來多少好處:
async function captureReport() {
const browser = await puppeteer.launch({headless: false});
const page = await browser.newPage();
const testUrl = 'https://web.dev/performance-scoring/';
const flow = await startFlow(page, {name: 'Cold and warm navigations'});
await flow.navigate(testUrl, {
stepName: 'Cold navigation'
});
await flow.navigate(testUrl, {
stepName: 'Warm navigation',
configContext: {
settingsOverrides: {disableStorageReset: true},
},
});
await browser.close();
const report = await flow.generateReport();
fs.writeFileSync('flow.report.html', report);
open('flow.report.html', {wait: false});
}
captureReport();
產生的流程報表如下所示:
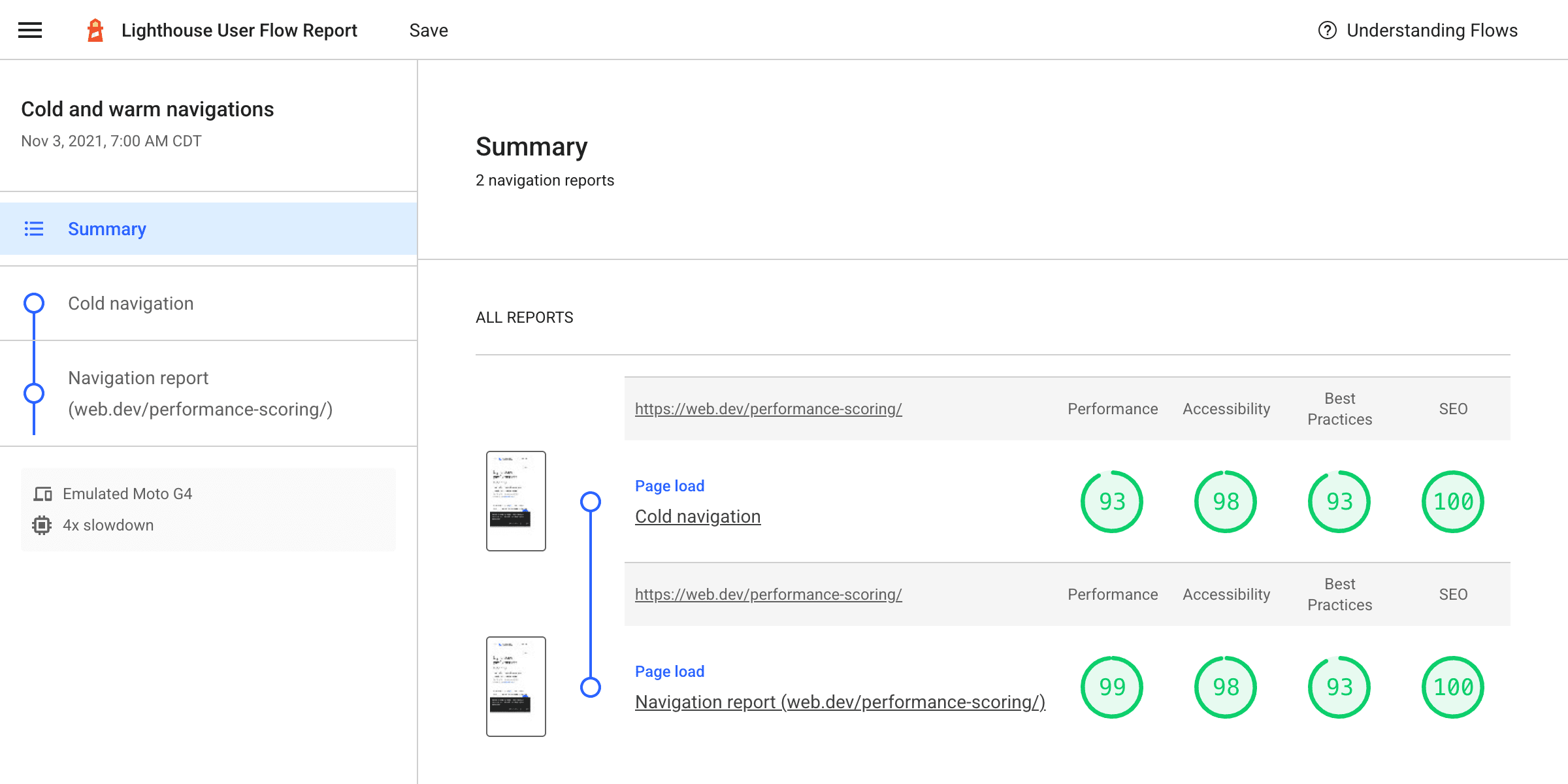
冷載入和暖載入結合使用,可讓您更全面地瞭解使用者的實際體驗。如果您的網站使用者在同一次造訪中載入了多個網頁,這或許能讓您更具體瞭解實際情境。
快照
快照是一種新模式,可在單一時間點執行 Lighthouse 稽核。與一般 Lighthouse 執行作業不同的是,系統不會重新載入網頁。這項功能可讓您設定網頁,並在確切狀態下測試網頁,例如開啟下拉式選單或部分填入表單。
在本範例中,假設您想檢查 Squoosh 中進階設定的部分新 UI 通過自動化 Lighthouse 檢查。只有在已載入圖片,且選項選單展開顯示進階設定時,才會看到這些設定。
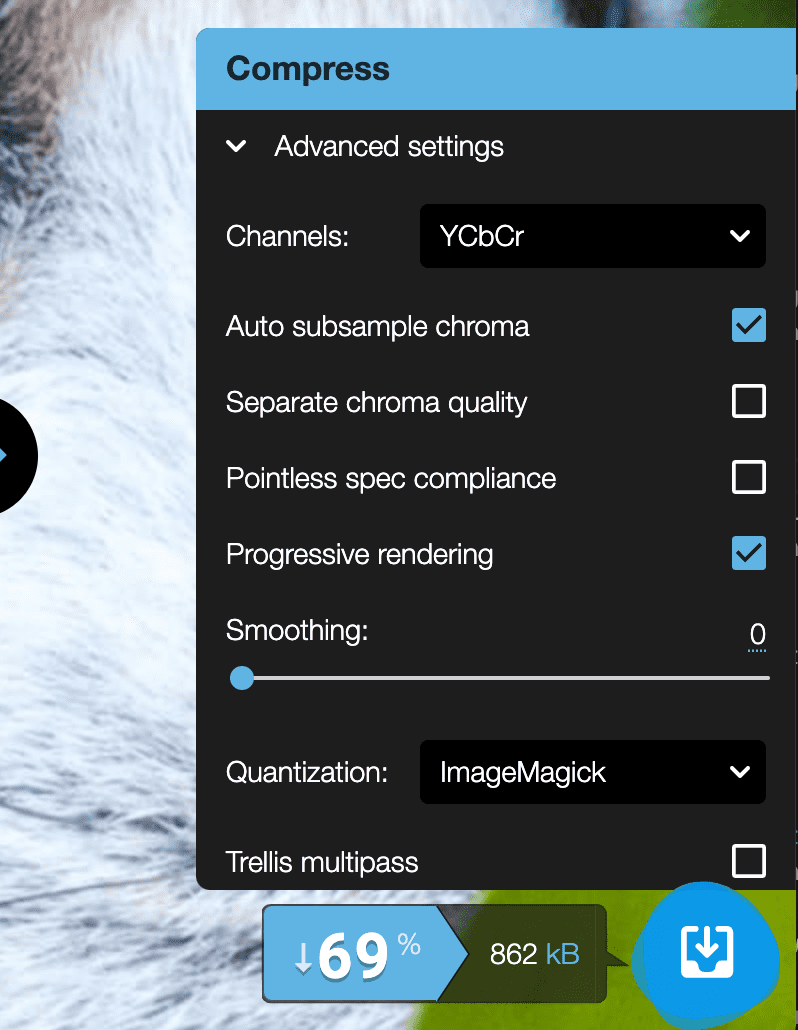
這個程序可透過 Puppeteer 編寫指令碼,您可以在每個步驟中拍攝 Lighthouse 快照:
async function captureReport() {
const browser = await puppeteer.launch({headless: false});
const page = await browser.newPage();
const flow = await startFlow(page, {name: 'Squoosh snapshots'});
await page.goto('https://squoosh.app/', {waitUntil: 'networkidle0'});
// Wait for first demo-image button, then open it.
const demoImageSelector = 'ul[class*="demos"] button';
await page.waitForSelector(demoImageSelector);
await flow.snapshot({stepName: 'Page loaded'});
await page.click(demoImageSelector);
// Wait for advanced settings button in UI, then open them.
const advancedSettingsSelector = 'form label[class*="option-reveal"]';
await page.waitForSelector(advancedSettingsSelector);
await flow.snapshot({stepName: 'Demo loaded'});
await page.click(advancedSettingsSelector);
await flow.snapshot({stepName: 'Advanced settings opened'});
browser.close();
const report = await flow.generateReport();
fs.writeFileSync('flow.report.html', report);
open('flow.report.html', {wait: false});
}
captureReport();
產生的報表顯示結果普遍良好,但可能有部分無障礙標準需要手動檢查:
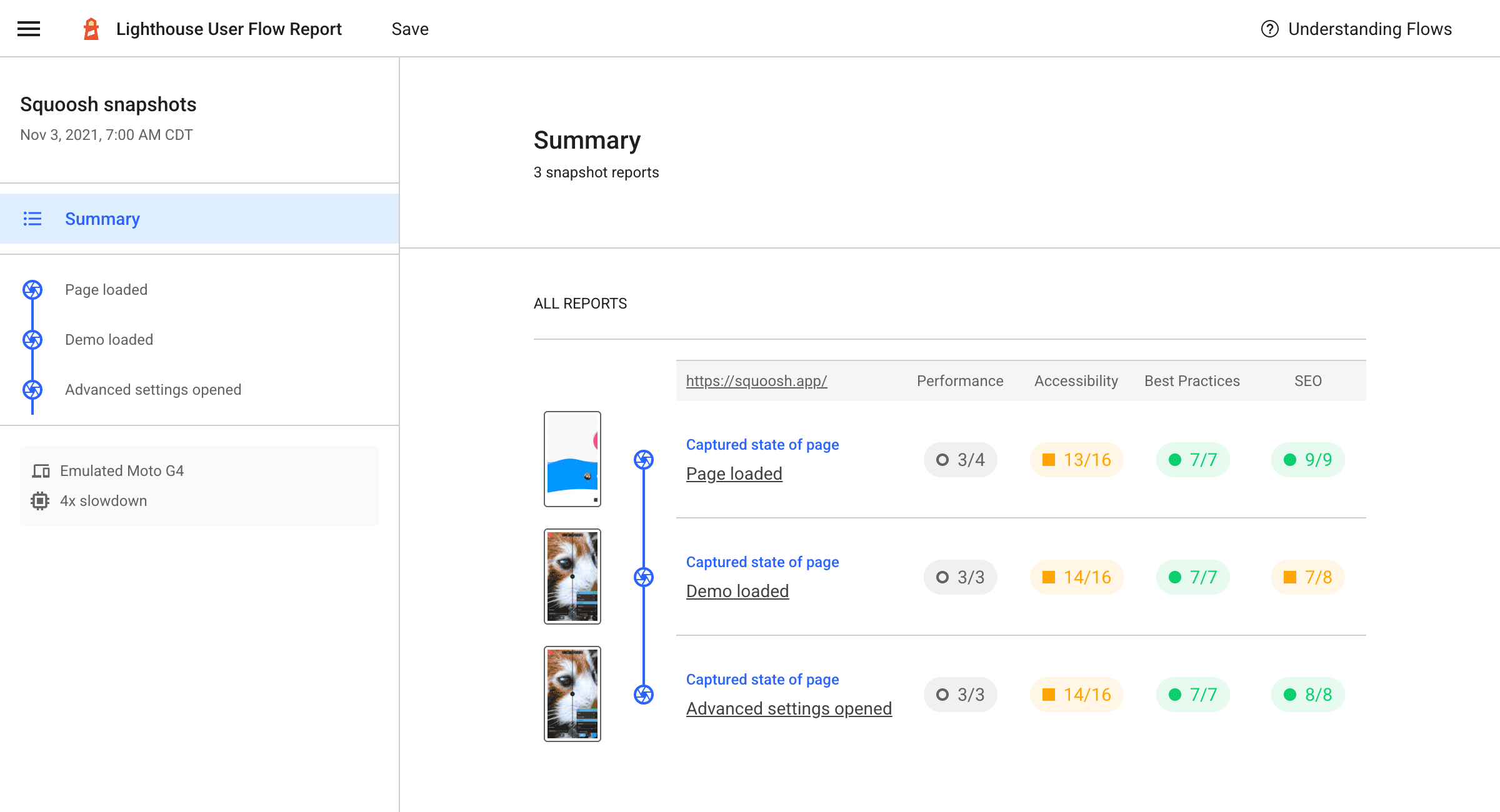
時間範圍
現場(例如 CrUX) 和實驗室 (例如 Lighthouse) 的效能結果之間最大的差異之一,就是缺乏使用者輸入內容。這時,時間範圍 (即最後的使用者流程模式) 就能派上用場。
時間範圍會在一段時間內執行 Lighthouse 稽核作業,可能會或未包含導覽。這個方法很適合用來擷取網頁在互動期間發生的情況。舉例來說,Lighthouse 預設會在網頁載入期間測量 CLS,但在欄位中預設測量 CLS,從初次瀏覽到關閉為止。如果使用者互動是 CLS 的原因,那麼 Lighthouse 先前無法擷取及協助修正。
為說明這項功能,我們建立了一個測試網站,模擬在捲動時將廣告插入文章,但未為廣告預留空間的情況。在長串資訊卡中,當版位進入可視區域時,偶爾會新增紅色方塊。由於沒有為這些紅色方塊保留空間,因此下方的資訊卡會偏離位置,導致版面配置偏移。
一般 Lighthouse 導覽的 CLS 為 0。不過,頁面捲動頁面後,頁面會出現版面配置位移的問題,而且 CLS 值也會上升。
以下指令碼會產生包含兩個動作的使用者流程報表,以顯示差異。
async function captureReport() {
const browser = await puppeteer.launch({headless: false});
const page = await browser.newPage();
// Get a session handle to be able to send protocol commands to the page.
const session = await page.target().createCDPSession();
const testUrl = 'https://pie-charmed-treatment.glitch.me/';
const flow = await startFlow(page, {name: 'CLS during navigation and on scroll'});
// Regular Lighthouse navigation.
await flow.navigate(testUrl, {stepName: 'Navigate only'});
// Navigate and scroll timespan.
await flow.startTimespan({stepName: 'Navigate and scroll'});
await page.goto(testUrl, {waitUntil: 'networkidle0'});
// We need the ability to scroll like a user. There's not a direct puppeteer function for this, but we can use the DevTools Protocol and issue a Input.synthesizeScrollGesture event, which has convenient parameters like repetitions and delay to somewhat simulate a more natural scrolling gesture.
// https://chromedevtools.github.io/devtools-protocol/tot/Input/#method-synthesizeScrollGesture
await session.send('Input.synthesizeScrollGesture', {
x: 100,
y: 600,
yDistance: -2500,
speed: 1000,
repeatCount: 2,
repeatDelayMs: 250,
});
await flow.endTimespan();
await browser.close();
const report = await flow.generateReport();
fs.writeFileSync('flow.report.html', report);
open('flow.report.html', {wait: false});
}
captureReport();
這會產生報表,比較一般導覽與時間範圍,其中包含導覽和捲動:
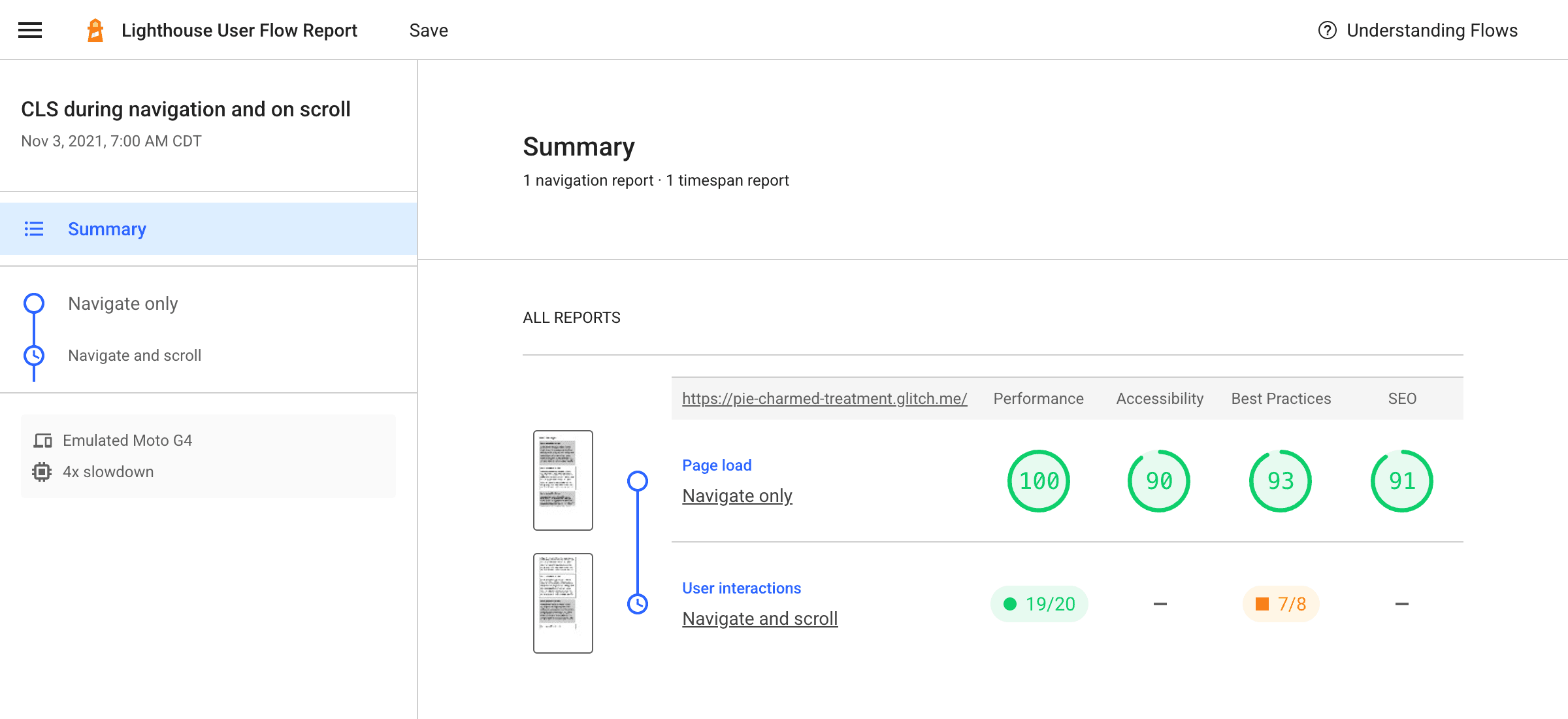
深入探討各個步驟,僅導覽的步驟顯示的 CLS 為 0。網站很棒!
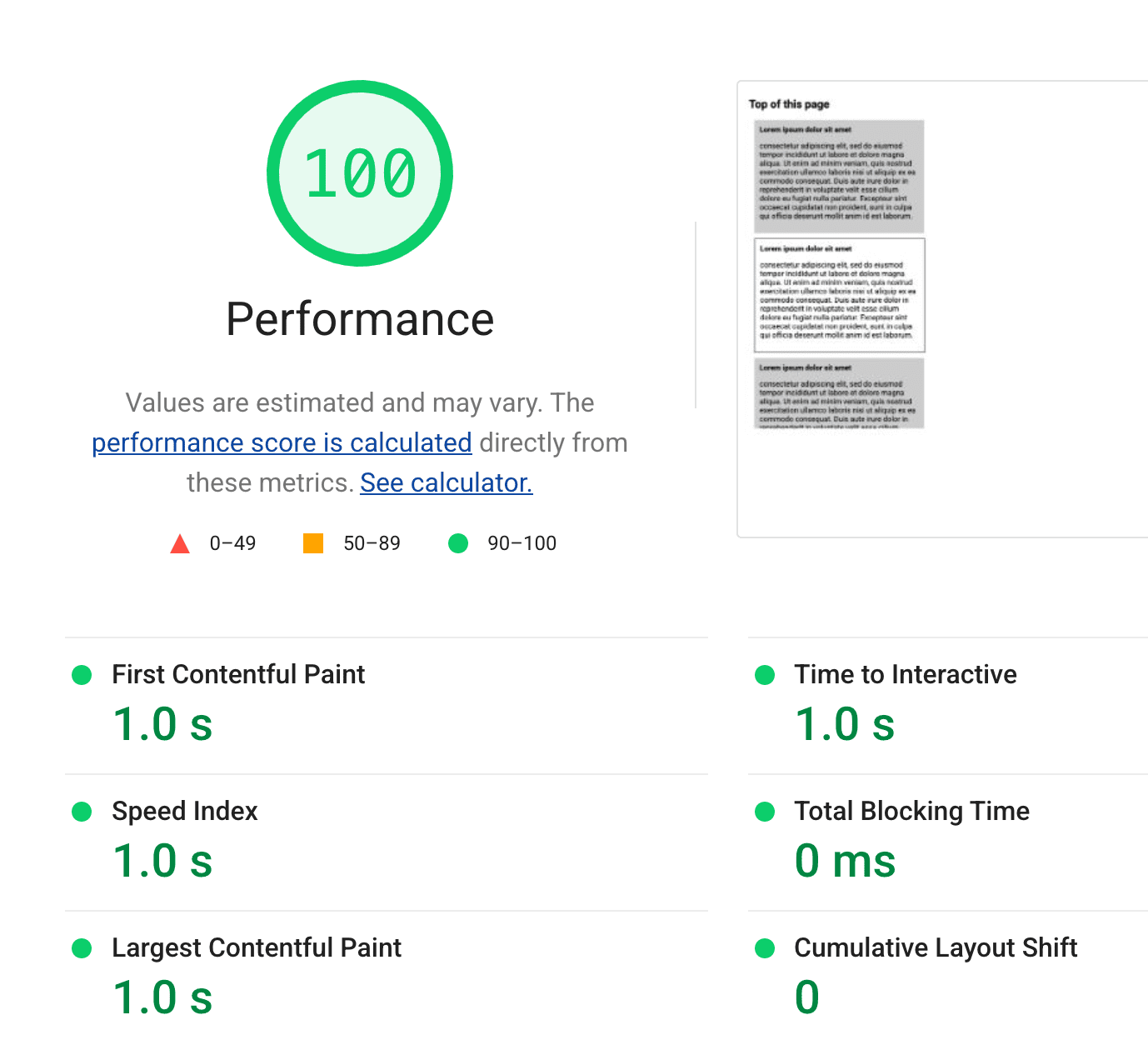
不過「導覽及捲動」步驟則講述不同故事。目前只有「總阻斷時間」和「累計版面配置位移」可用於時間範圍,但這個頁面上延後載入的內容顯然會降低網站的 CLS。
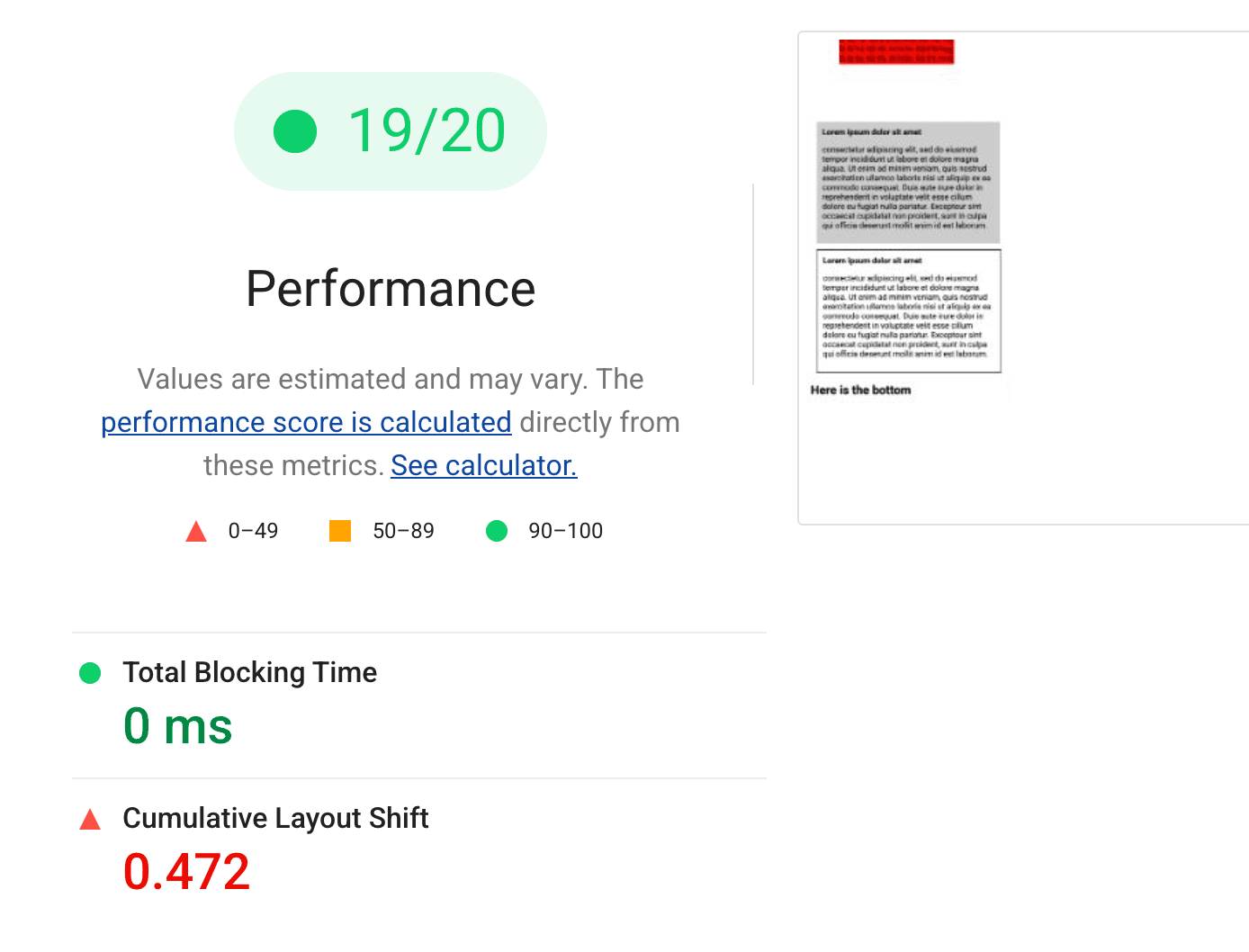
先前 Lighthouse 無法識別這個有問題的 CLS 行為,但這幾乎肯定會出現在實際使用者的體驗中。對事先擬好腳本的互動能力測試可大幅提升研究室的保真度。
尋求意見回饋
Lighthouse 中的新使用者流程 API 可執行許多新功能,但評估使用者遇到的情況類型仍可能相當複雜。
如有任何疑問,請在 Lighthouse 論壇中與我們聯絡,並透過 Issue Tracker 回報錯誤或提出建議。