Por que usar notificações push?
As notificações apresentam pequenos pedaços de informação para o usuário. Os apps da Web podem usar notificações para informar os usuários sobre eventos importantes ou ações que precisam ser realizadas.
A aparência das notificações varia de acordo com a plataforma. Exemplo:
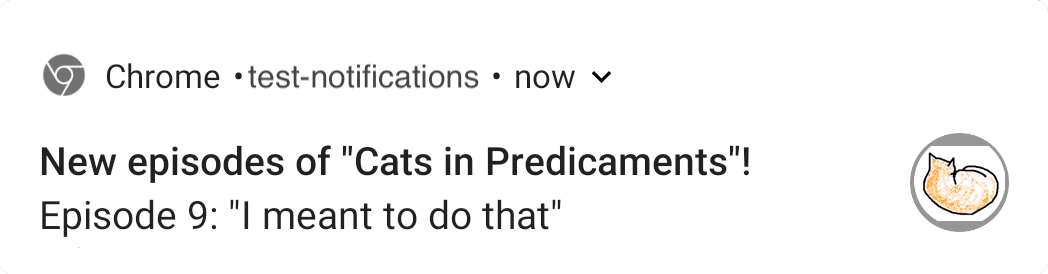

Tradicionalmente, os navegadores da Web precisavam iniciar a troca de informações entre o servidor e o cliente fazendo uma solicitação. A tecnologia de push da Web, por outro lado, permite configurar o servidor para enviar notificações quando for apropriado para o app. Um serviço push cria URLs exclusivos para cada service worker assinado. O envio de mensagens para o URL de um service worker gera eventos nesse service worker, fazendo com que ele exiba uma notificação.
As notificações push podem ajudar os usuários a aproveitar ao máximo o app, pedindo que eles o abram e o usem com base nas informações mais recentes.
Criar e enviar notificações
Crie notificações usando a API Notifications. Um objeto Notification
tem uma string title
e um objeto options
. Exemplo:
let title = 'Hi!';
let options = {
body: 'Very Important Message',
/* other options can go here */
};
let notification = new Notification(title, options);
O title
aparece em negrito quando a notificação está ativa, enquanto o body
contém texto adicional.
Receber permissão para enviar notificações
Para mostrar uma notificação, o app precisa receber permissão do usuário:
Notification.requestPermission();
Como funcionam as notificações push?
O verdadeiro poder das notificações vem da combinação de service workers e tecnologia push:
Os service workers podem ser executados em segundo plano e mostrar notificações mesmo quando o app não está visível na tela.
A tecnologia push permite configurar o servidor para enviar notificações quando for apropriado para o app. Um serviço push cria URLs exclusivos para cada worker de serviço inscrito. O envio de mensagens para o URL de um service worker gera eventos nesse service worker, fazendo com que ele exiba uma notificação.
No exemplo de fluxo abaixo, um cliente registra um worker de serviço e se inscreve em notificações push. Em seguida, o servidor envia uma notificação para o endpoint da assinatura.
O cliente e o worker de serviço usam JavaScript vanilla sem bibliotecas extras. O servidor é criado com o pacote npm express
no Node.js e usa o pacote npm web-push
para enviar notificações. Para enviar informações ao servidor, o cliente faz uma chamada para um URL POST exposto pelo servidor.
Parte 1: registrar um service worker e se inscrever em push
Um app cliente registra um service worker com
ServiceWorkerContainer.register()
. O service worker registrado vai continuar em execução em segundo plano quando o cliente estiver inativo.Código do cliente:
navigator.serviceWorker.register('sw.js');
O cliente solicita permissão do usuário para enviar notificações.
Código do cliente:
Notification.requestPermission();
Para ativar as notificações push, o service worker usa
PushManager.subscribe()
para criar uma assinatura de push. Na chamada paraPushManager.subscribe()
, o worker de serviço fornece a chave de API do app como um identificador.Código do cliente:
navigator.serviceWorker.register('sw.js').then(sw => {
sw.pushManager.subscribe({ /* API key */ });
});Os serviços push, como o Firebase Cloud Messaging, operam em um modelo de assinatura. Quando um worker de serviço se inscreve em um serviço de push chamando
PushManager.subscribe()
, o serviço de push cria um URL exclusivo para esse worker. O URL é conhecido como endpoint de assinatura.O cliente envia o endpoint de assinatura ao servidor do app.
Código do cliente:
navigator.serviceWorker.register('sw.js').then(sw => {
sw.pushManager.subscribe({ /* API key */ }).then(subscription => {
sendToServer(subscription, '/new-subscription', 'POST');
});
});Código do servidor:
app.post('/new-subscription', (request, response) => {
// extract subscription from request
// send 'OK' response
});
Parte 2: enviar uma notificação
O servidor da Web envia uma notificação para o endpoint de assinatura.
Código do servidor:
const webpush = require('web-push');
let options = { /* config info for cloud messaging and API key */ };
let subscription = { /* subscription created in Part 1*/ };
let payload = { /* notification */ };
webpush.sendNotification(subscription, payload, options);As notificações enviadas para o endpoint de assinatura acionam eventos push com o worker de serviço como destino. O service worker recebe a mensagem e a exibe para o usuário como uma notificação.
Código do worker de serviço:
self.addEventListener('push', (event) => {
let title = { /* get notification title from event data */ }
let options = { /* get notification options from event data */ }
showNotification(title, options);
})O usuário interage com a notificação, ativando o app da Web, caso ainda não esteja.
Próximas etapas
Na próxima etapa, implemente as notificações push.
Criamos uma série de codelabs para orientar você em cada etapa do processo.