Published: March 4, 2025
Baseline was originated by the Chrome team and is now defined by the WebDX Community Group. Baseline brings clarity to features that are interoperable between browsers. It's designed to help you identify what features you can use—or can't use—across all major browser engines. However, you need a way to identify what those features are.
Thankfully, you have the ability to query which features are Baseline Newly or Widely available using the Web Platform Dashboard—which is powered by the web-features
npm package. You can also access this information using its HTTP API to integrate Baseline data into your tooling workflow, and this guide explains how.
The Web Platform Dashboard's relevant Baseline query grammar
The Web Platform dashboard uses a specific query grammar to help you search web feature support. You can use this query grammar directly on the dashboard itself.
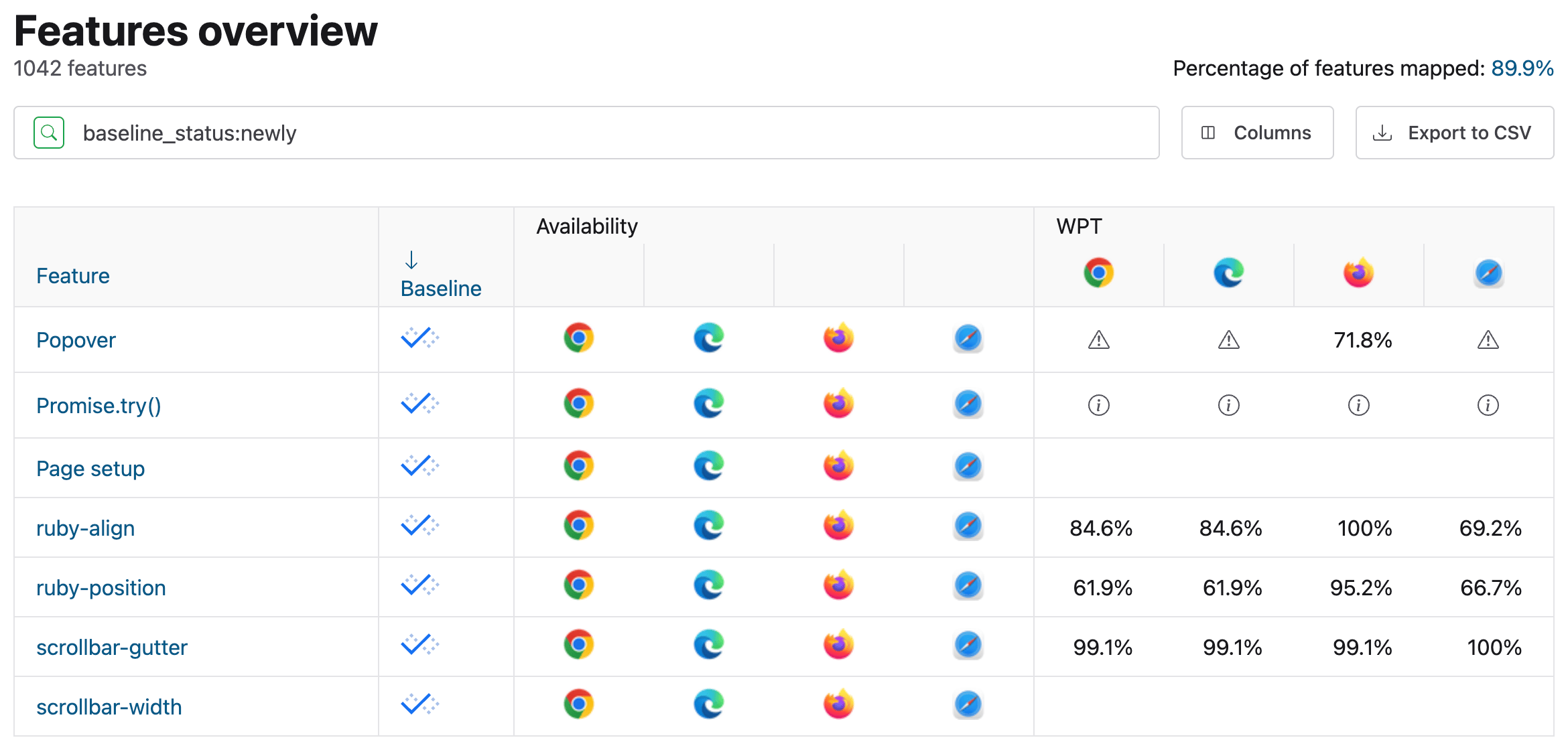
baseline_status:newly
search query.
As you type in the search box at the top of the page, you'll be presented with a number of query parameters you can use to filter web features.
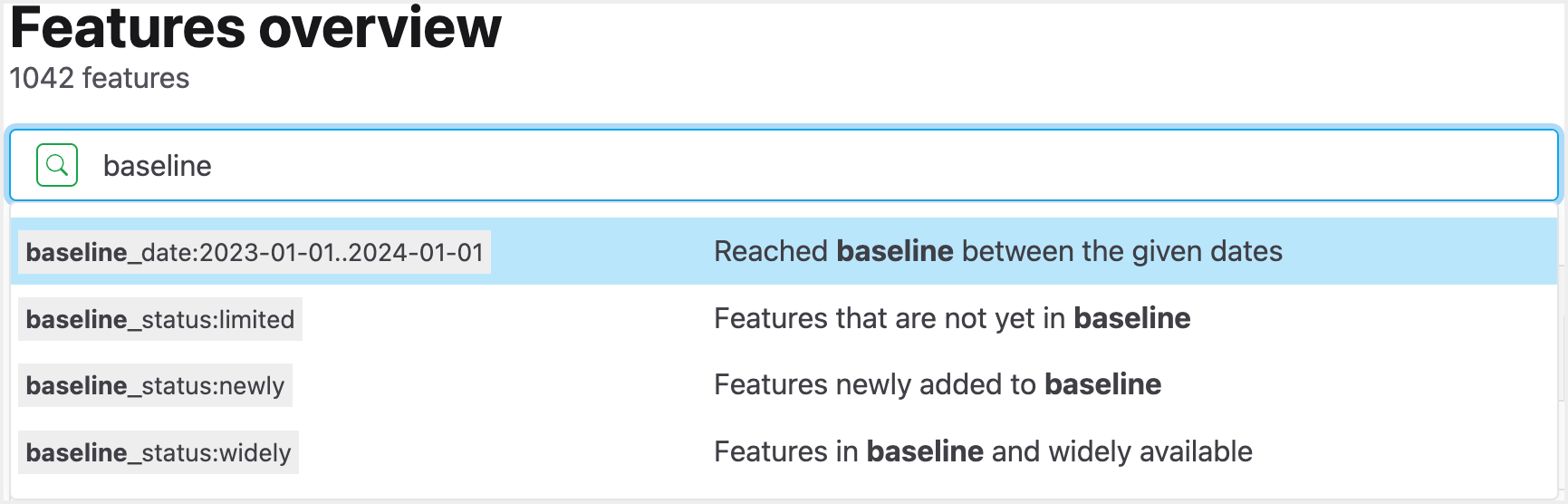
The query grammar is expressive, giving you a lot of flexibility in how to filter the web features that are displayed on the dashboard. The following screenshot shows how the id
query parameter can be used to narrow down to a specific feature:
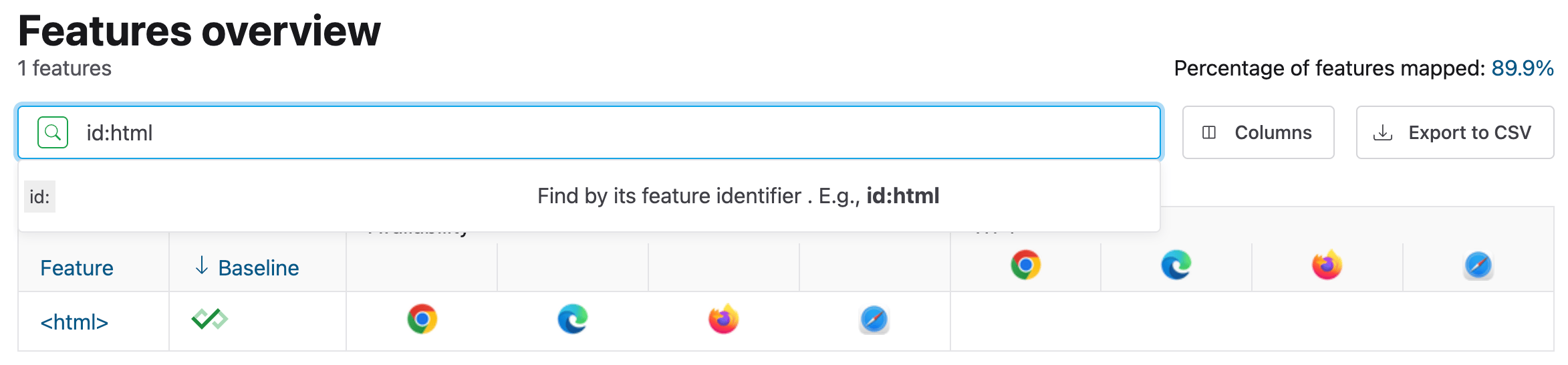
id
parameter in this example has a value of html
, which indicates support for the <html>
element, which is (unsurprisingly) Baseline Widely available.
The query grammar is documented—but knowing all of it is not required to find Baseline data for features. You can use any of these options on the dashboard, but here are some that are especially useful:
baseline_status
: Use this parameter to filter features by three enumerated values:limited
: Returns features that have limited browser support. If you filter by this value, you'll only receive features that have not reached any Baseline status.newly
: Returns features that are Baseline Newly available.widely
: Returns features that are Baseline Widely available, which are features that have been Baseline for at least 30 months. These are features that you can expect to use without worrying about browser support or polyfills.
baseline_date
: Uses aYYYY-MM-DD..YYYY-MM-DD
format to specify an upper and lower bound for when features reached Baseline. For example, to find all features in the span of a year, use a value like2024-01-01..2025-01-01
.id
: The identifier for a given feature. These identifiers are defined in the web-features package. For example, the feature entry forPromise.try()
maps to an ID ofpromise-try
.group
: One of many enumerated group names for features. This is useful criteria if you only want to query for a certain subset of web platform features. For example, you could filter to a list of CSS features with a value ofcss
.
While the dashboard frontend is certainly useful, it rests on top of an HTTP API that you can query directly. For example, here's an endpoint that gets all Baseline features that are Newly available:
https://api.webstatus.dev/v1/features?q=baseline_status:newly
The JSON response structure
The JSON response you receive from the HTTP API has a consistent shape for every feature. The response returned contains a data
property at the top level. This property contains an array of all matching features. While this isn't exhaustive list of all the fields available in the JSON response, here are some useful ones when it comes to Baseline:
baseline
: Contains general information about the Baseline status of a given feature, with the following subfields:status
: The Baseline status for a feature. Values can be eitherlimited
,newly
, orwidely
. Note: this will be the only subfield ifstatus
has a value oflimited
.low_date
: This indicates the date on which the given feature became Baseline Newly available. This field only appears ifstatus
is eithernewly
orwidely
.high_date
: This indicates the date on which the given feature became Baseline Widely available. This field is only available ifstatus
iswidely
.
feature_id
: The ID for the feature. For example, for CSS grid, this would be a value of"grid"
.name
: The formatted name of the feature. This can be similar tofeature_id
in some cases, but is usually different. For example, thefeature_id
value forPromise.try()
is"promise-try"
, whereas thename
field for the same feature is"Promise.try()"
.spec
: This field contains a subfield namedlinks
, which is an array of links to specifications and other resources.
There are other fields, often containing information about when specific supporting browsers implemented the feature at which version, data about Web Platform Tests, and other things you may or may not care about.
Example queries
Now that you have a quick explanation of some of the available query parameters, take a look at some example queries that you can use in your tools and scripts to select web status features that could be useful for your workflow.
Get data for a single feature
A good way to get familiar with the API is to start with a basic example that gets data for a single web feature.
// Specify and encode the query for a query string:
const query = encodeURIComponent('id:grid');
// Construct the URL:
let url = `https://api.webstatus.dev/v1/features?q=${query}`;
// Fetch the resource:
const response = await fetch(url);
if (response.ok) {
// Convert the response to JSON:
const { data } = await response.json();
// Log data for each feature to the:
console.log(data);
}
In this case, we specify an id
parameter with a value of grid
to get feature support information for CSS grid, which is Baseline Widely available. You could use this information, for example, to detect usage of the feature and let users know that they can use it in all modern browser engines without worrying about support.
This is just a start, though, and the HTTP API can do much more for you than get data for a single feature.
Get all Baseline Newly and Widely available features
Say you'd like to have a script that pulls in all features that are either Baseline Newly or Widely available. This could be useful, for example, if you have a script that runs on some interval and you'd like to get an updated list that changes as features emerge from limited availability to either Baseline status:
const query = encodeURIComponent('-baseline_status:limited');
let url = `https://api.webstatus.dev/v1/features?q=${query}`;
const response = await fetch(url);
if (response.ok) {
const { data } = await response.json();
console.log(data);
}
This query doesn't get all Baseline Newly and Widely available features, but only the first 100. If the number of features retrieved exceeds this, there is a metadata
field at the top level of the JSON response that can contain up to two subfields:
next_page_token
: A string containing a token you can add to the query string of theGET
request to the backend. When you use it and re-fetch data from the webstatus.dev backend, it will return the next batch of matching features. Note: This field will be unavailable if the current query returns less than 100 results, or if the current query is at the end of the result set.total
: An integer indicating the total number of features available for the current query.
These fields are useful for pagination. With them, we can try the following code to get all of the results for a query that returns a large amount of data:
async function queryWebStatusDashboard (query, token) {
const urlBase = 'https://api.webstatus.dev/v1/features?q=';
let queryUrl = `${urlBase}${encodeURIComponent(query)}`;
if (token) {
queryUrl += `&page_token=${encodeURIComponent(token)}`;
}
const response = await fetch(queryUrl);
if (response.ok) {
const { data, metadata } = await response.json();
console.log(data);
// See if there's a page token in this query:
if ('next_page_token' in metadata) {
const { next_page_token } = metadata;
queryWebStatusDashboard(query, next_page_token);
} else {
console.log('All results collected');
}
}
}
// Make the first query, and if there are more
// than 100 entries, the function will run
// recursively until all features are fetched
queryWebStatusDashboard('-baseline_status:limited');
Get all Baseline Newly available CSS features
Say you're an engineer with a special focus on CSS, and you're interested to know which CSS features become Baseline Newly available features. This is a perfect use case for the group
query value, as well as how to use the AND
operator when querying webstatus.dev:
const query = encodeURIComponent('baseline_status:newly AND group:css');
let url = `https://api.webstatus.dev/v1/features?q=${query}`;
const response = await fetch(url);
if (response.ok) {
const { data } = await response.json();
console.log(data);
}
When specifying a value of css
for group
, you can query for all Baseline Newly available features. If you wanted to expand the scope to include Baseline Widely available CSS features as well, you could use the approach from the last code sample and use the negation operator with a query such as -baseline_status:limited AND group:css'
.
Another field you can query is the snapshot
field, which is useful for finding JavaScript features that are part of a specific set of ECMAScript features. The following code checks for all Baseline Newly available features that are part of the ecmascript-2023
snapshot:
const query = encodeURIComponent('baseline_status:newly AND snapshot:ecmascript-2023');
let url = `https://api.webstatus.dev/v1/features?q=${query}`;
const response = await fetch(url);
if (response.ok) {
const { data } = await response.json();
console.log(data);
}
Get all Baseline features within a date range
The baseline_date
field can be queried to find all features that became Baseline within a specific date range:
const query = encodeURIComponent('baseline_status:widely AND baseline_date:2022-01-01..2022-12-31 AND group:css');
let url = `https://api.webstatus.dev/v1/features?q=${query}`;
const response = await fetch(url);
if (response.ok) {
const { data } = await response.json();
console.log(data);
}
The preceding code sample will query for CSS features that became Baseline Widely available at any point in 2022. The query syntax for baseline_date
lets you specify a beginning and ending date, separated by ..
.
Conclusion
The queries in this guide are meant to be a starting point for you to begin experimenting with how to query the webstatus.dev backend. With any number of available query parameters, you should be able to get results that are specific to your application.
Knowing how to query the Web Platform Dashboard's HTTP API gives you the ability to build tools that might be useful for your work, and keep you informed as to whether the features you want to use for your project have broad enough browser support. This means you can build web applications using modern browser features that you can safely use, providing a much more enjoyable developer experience.