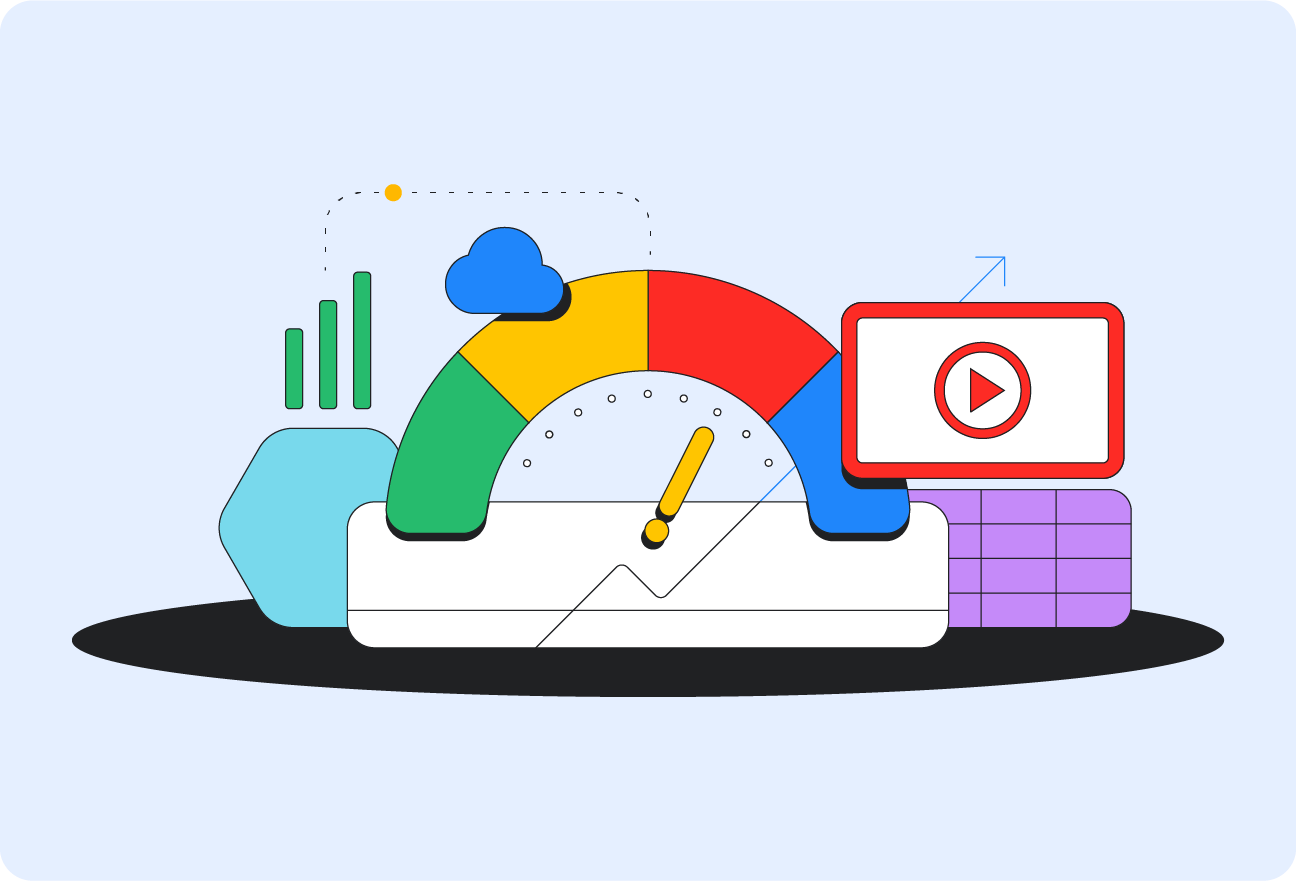
Web performance
Jump ahead on this page:
Learn performance
Core Web Vitals
Optimize Core Web Vitals
Dive further into Core Web Vitals
Baseline Newly available performance features
Case studies
Debug performance with Chrome DevTools
New to performance?
Web performance is a complex and multi-faceted subject matter area—but it's not impossible to learn! If you're new to performance, our course will help you to hit the ground running by first introducing you to performance basis, allowing you to work your way through more advanced topics. Once you've completed the course, you'll be able to apply what you've learned in no time!
Core Web Vitals
The Core Web Vitals metrics are a set of three metrics focused on the user experience. They measure the performance and usability of pages, including perceived load time, visual stability, and responsiveness to user input. If you're new to Core Web Vitals, these guides will familiarize you with how they work, and give you a starting point on how to optimize them.
Largest Contentful Paint (LCP)
Cumulative Layout Shift (CLS)
Interaction to Next Paint (INP)
Optimize Core Web Vitals
Optimize Largest Contentful Paint (LCP)
Optimize Cumulative Layout Shift (CLS)
Optimize Interaction to Next Paint (INP)
Dive further into Core Web Vitals
Case studies
Disney+ Hotstar
PubTech
Taboola
Baseline Newly available web performance features
Baseline signals to web developers when web platform features can be safely used in all major browser engines. Here are some web performance-related features that are now Baseline Newly available.
Debug performance with Chrome DevTools
Chrome DevTools is a suite of tools for developers to debug web applications, including tools to help you understand and improve the performance of your web applications.