Next.js आपके React ऐप्लिकेशन में कई ऑप्टिमाइज़ेशन करता है, ताकि आपको
Next.js, React के हिसाब से बनाया गया फ़्रेमवर्क है. इसमें परफ़ॉर्मेंस को ऑप्टिमाइज़ करने के कई तरीके पहले से मौजूद होते हैं. फ़्रेमवर्क के पीछे का मुख्य मकसद यह पक्का करना है कि ऐप्लिकेशन डिफ़ॉल्ट रूप से इन सुविधाओं के साथ शुरू हों और बेहतर परफ़ॉर्म करते रहें.
इस परिचय में, फ़्रेमवर्क की कई सुविधाओं के बारे में कम शब्दों में बताया गया है. इस कलेक्शन की अन्य गाइड में, इन सुविधाओं के बारे में ज़्यादा जानकारी दी गई है.
आपको क्या सीखने को मिलेगा?
Next.js, डिफ़ॉल्ट रूप से परफ़ॉर्मेंस को ऑप्टिमाइज़ करने के कई तरीके उपलब्ध कराता है. हालांकि, इन गाइड में इन तरीकों के बारे में ज़्यादा जानकारी दी गई है. साथ ही, इनमें यह भी बताया गया है कि इनका इस्तेमाल करके, तेज़ और बेहतर परफ़ॉर्मेंस वाला अनुभव कैसे बनाया जा सकता है.
आम तौर पर, React साइटों में कई ऑप्टिमाइज़ेशन जोड़े जा सकते हैं. ये ऑप्टिमाइज़ेशन, Next.js की मदद से बनाए गए ऐप्लिकेशन के लिए भी काम करेंगे. इनके बारे में नहीं बताया जाएगा, क्योंकि हमारा फ़ोकस खास तौर पर Next.js के फ़ायदों पर है. React के सामान्य ऑप्टिमाइज़ेशन के बारे में ज़्यादा जानने के लिए, हमारा React कलेक्शन देखें.
Next.js, React से किस तरह अलग है?
React एक लाइब्रेरी है, जिसकी मदद से कॉम्पोनेंट पर आधारित तरीके का इस्तेमाल करके, यूज़र इंटरफ़ेस बनाना आसान हो जाता है. React एक बेहतरीन लाइब्रेरी है, लेकिन यह खास तौर पर यूज़र इंटरफ़ेस (यूआई) के लिए है. कई डेवलपर, पूरी तरह से बने टूलचेन के लिए, अतिरिक्त टूल शामिल करते हैं. जैसे, मॉड्यूल बंडलर (उदाहरण के लिए, webpack) और ट्रांसपाइलर (उदाहरण के लिए, Babel).
React कलेक्शन में, हमने React ऐप्लिकेशन को तुरंत बनाने के लिए, Create React App (CRA) का इस्तेमाल किया. CRA, एक ही कमांड के साथ पूरी बिल्ड टूलचेन उपलब्ध कराकर, React ऐप्लिकेशन को सेट अप करने की परेशानी को कम करता है.
हालांकि, CRA में कुछ डिफ़ॉल्ट ऑप्टिमाइज़ेशन मौजूद हैं, लेकिन इस टूल का मकसद आसान और सीधा सेटअप उपलब्ध कराना है. डेवलपर यह तय कर सकते हैं कि उन्हें कॉन्फ़िगरेशन को हटाना है या नहीं. साथ ही, वे कॉन्फ़िगरेशन में बदलाव भी कर सकते हैं.
Next.js का इस्तेमाल, नया React ऐप्लिकेशन बनाने के लिए भी किया जा सकता है. हालांकि, यह एक अलग तरीके से काम करता है. यह तुरंत कई सामान्य ऑप्टिमाइज़ेशन उपलब्ध कराता है. कई डेवलपर को ये ऑप्टिमाइज़ेशन चाहिए होते हैं, लेकिन उन्हें सेट अप करने में मुश्किल होती है. जैसे:
- सर्वर साइड रेंडरिंग
- कोड को अपने-आप अलग-अलग हिस्सों में बांटना
- रास्ते की जानकारी को पहले से लोड करना
- फ़ाइल-सिस्टम रूटिंग
- CSS-in-JS स्टाइल (
styled-jsx
)
सेट अप किया जा रहा है
नया Next.js ऐप्लिकेशन बनाने के लिए, यह कमांड चलाएं:
npx create-next-app new-app
इसके बाद, डायरेक्ट्री पर जाएं और डेवलपमेंट सर्वर शुरू करें:
cd new-app
npm run dev
यहां दिए गए एम्बेड में, नए Next.js ऐप्लिकेशन का डायरेक्ट्री स्ट्रक्चर दिखाया गया है.
- प्रोजेक्ट में बदलाव करने के लिए, बदलाव करने के लिए रीमिक्स करें पर क्लिक करें.
- साइट की झलक देखने के लिए, ऐप्लिकेशन देखें दबाएं. इसके बाद, फ़ुलस्क्रीन
दबाएं.
ध्यान दें कि pages/
डायरेक्ट्री, एक फ़ाइल: index.jsx
के साथ बनाई गई है. Next.js, फ़ाइल-सिस्टम रूटिंग के तरीके का इस्तेमाल करता है. इसमें, इस डायरेक्ट्री के हर पेज को एक अलग रूट के तौर पर दिखाया जाता है. इस डायरेक्ट्री में कोई नई फ़ाइल बनाने पर, जैसे कि about.js
, अपने-आप एक नया रूट (/about
) बन जाएगा.
कॉम्पोनेंट को किसी भी दूसरे React ऐप्लिकेशन की तरह बनाया और इस्तेमाल किया जा सकता है. components/
डायरेक्ट्री पहले से ही एक कॉम्पोनेंट, nav.js
के साथ बनाई जा चुकी है. इसे पहले से ही index.js
में इंपोर्ट किया जा चुका है. डिफ़ॉल्ट रूप से, Next.js में इस्तेमाल किए गए हर इंपोर्ट को सिर्फ़ तब फ़ेच किया जाता है, जब वह पेज लोड होता है. इससे कोड को अपने-आप अलग-अलग हिस्सों में बांटने के फ़ायदे मिलते हैं.
इसके अलावा, Next.js में हर शुरुआती पेज लोड को सर्वर साइड रेंडर किया जाता है. DevTools में नेटवर्क पैनल खोलने पर, आपको दस्तावेज़ के लिए किए गए शुरुआती अनुरोध के तौर पर, पूरी तरह से सर्वर से रेंडर किया गया पेज दिखेगा.
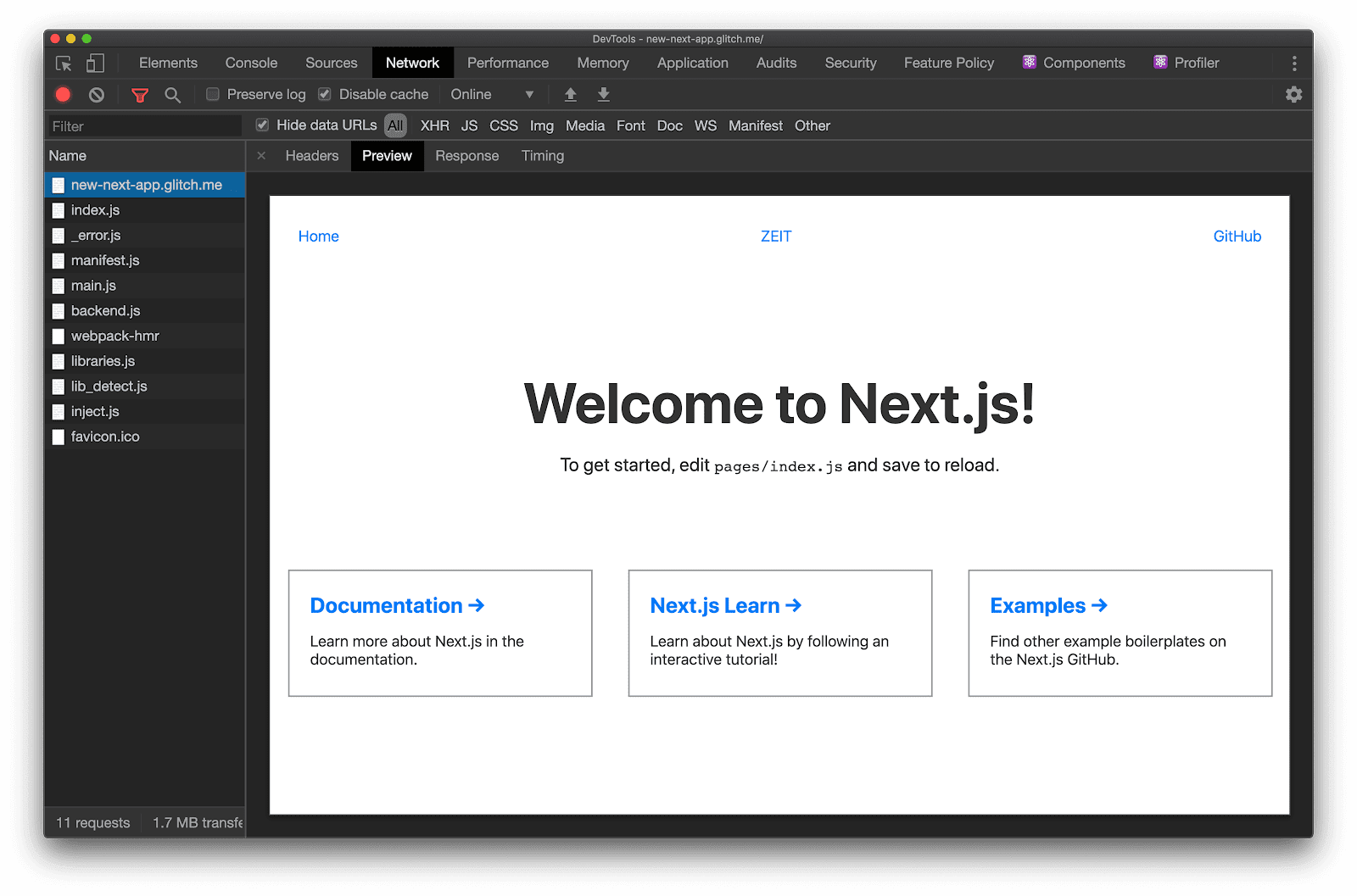
Next.js की कई सुविधाएं अपने-आप काम करती हैं. इनमें से ये सिर्फ़ कुछ सुविधाएं हैं. कई टास्क को अपनी ज़रूरत के हिसाब से बनाया जा सकता है. साथ ही, अलग-अलग कामों के लिए उनमें बदलाव किया जा सकता है.
आगे क्या करना है?
जान-बूझकर किया गया है 😛
इस कलेक्शन में मौजूद हर दूसरी गाइड में, Next.js की किसी खास सुविधा के बारे में पूरी जानकारी दी जाएगी:
- पेज नेविगेशन की रफ़्तार बढ़ाने के लिए, रास्ते को पहले से लोड करना
- सर्च इंजन से तेज़ी से लोड होने के लिए, हाइब्रिड और सिर्फ़ एएमपी पेजों को दिखाना
- JavaScript फ़ुटप्रिंट को कम करने के लिए, डाइनैमिक इंपोर्ट के साथ कोड को अलग-अलग करने वाले कॉम्पोनेंट का इस्तेमाल करना