O que os apps Google Assistente, Slack, Zoom e quase qualquer outro app específico da plataforma no smartphone ou computador têm em comum? Certo, eles sempre dão pelo menos algo. Mesmo sem uma conexão de rede, ainda é possível abrir o app Assistente, entrar no Slack ou iniciar o Zoom. Talvez você não consiga nada particularmente significativo ou não consegue alcançar o que queria, mas pelo menos você consegue algo e o app está no controle.
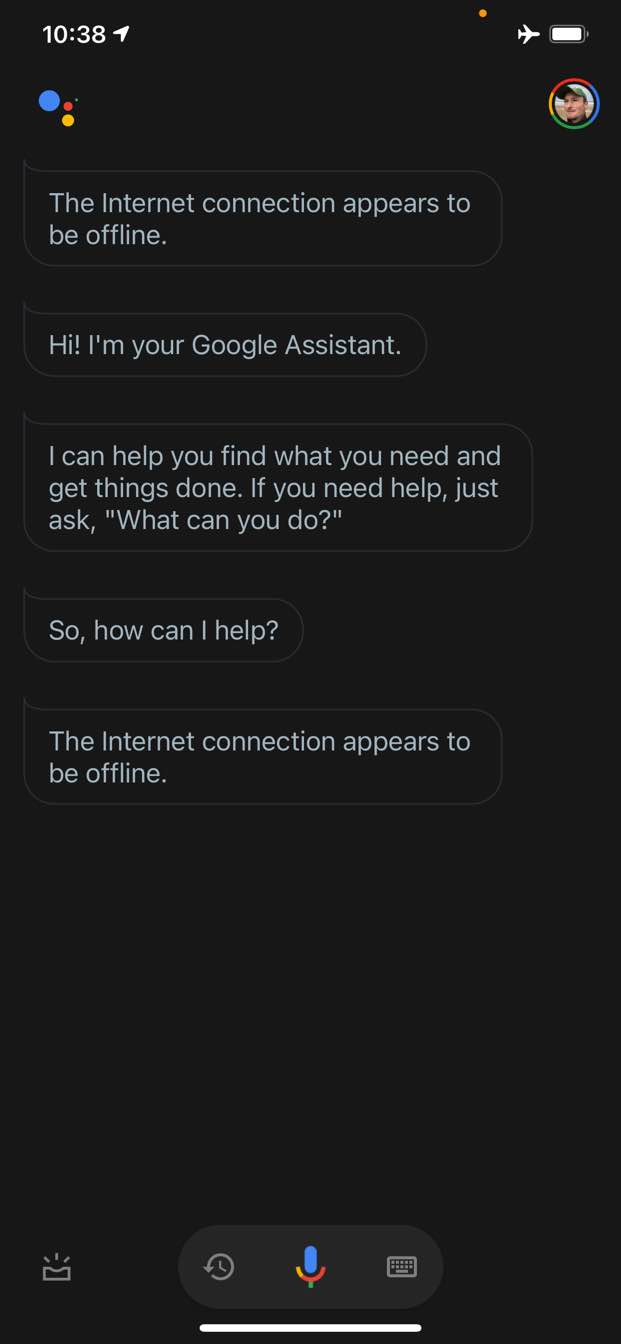
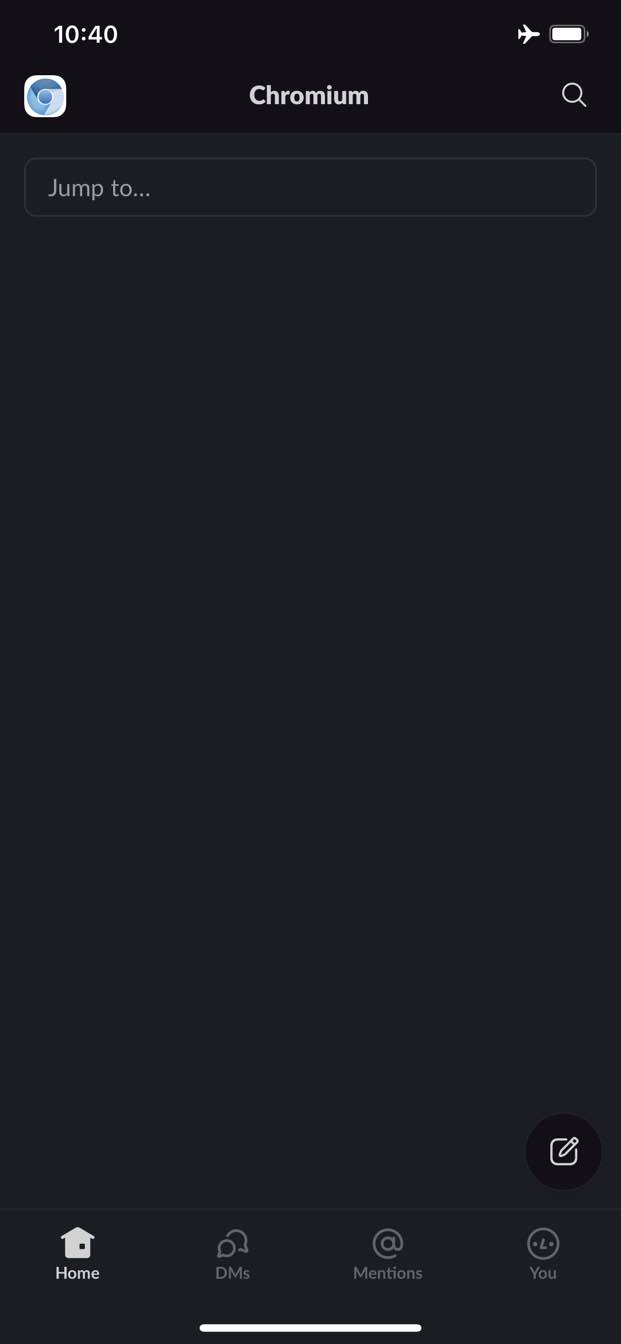
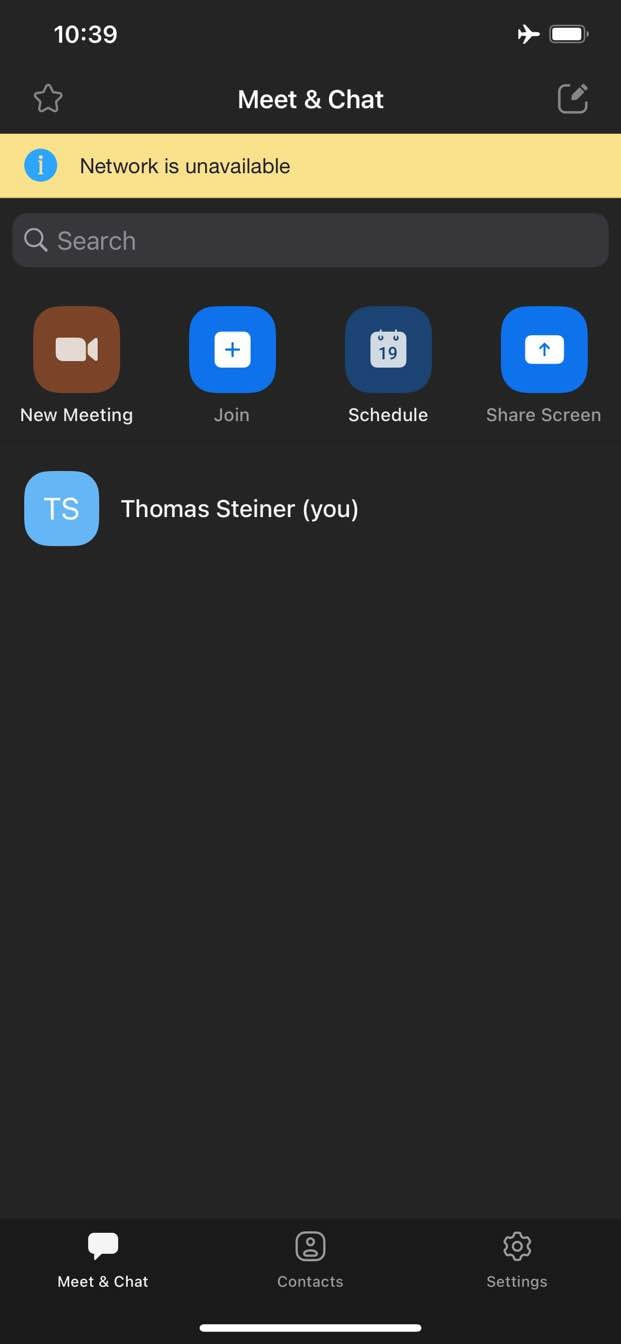
Por outro lado, na Web, tradicionalmente você não recebe nada quando está off-line. O Chrome oferece o jogo do dinossauro off-line, mas é isso.
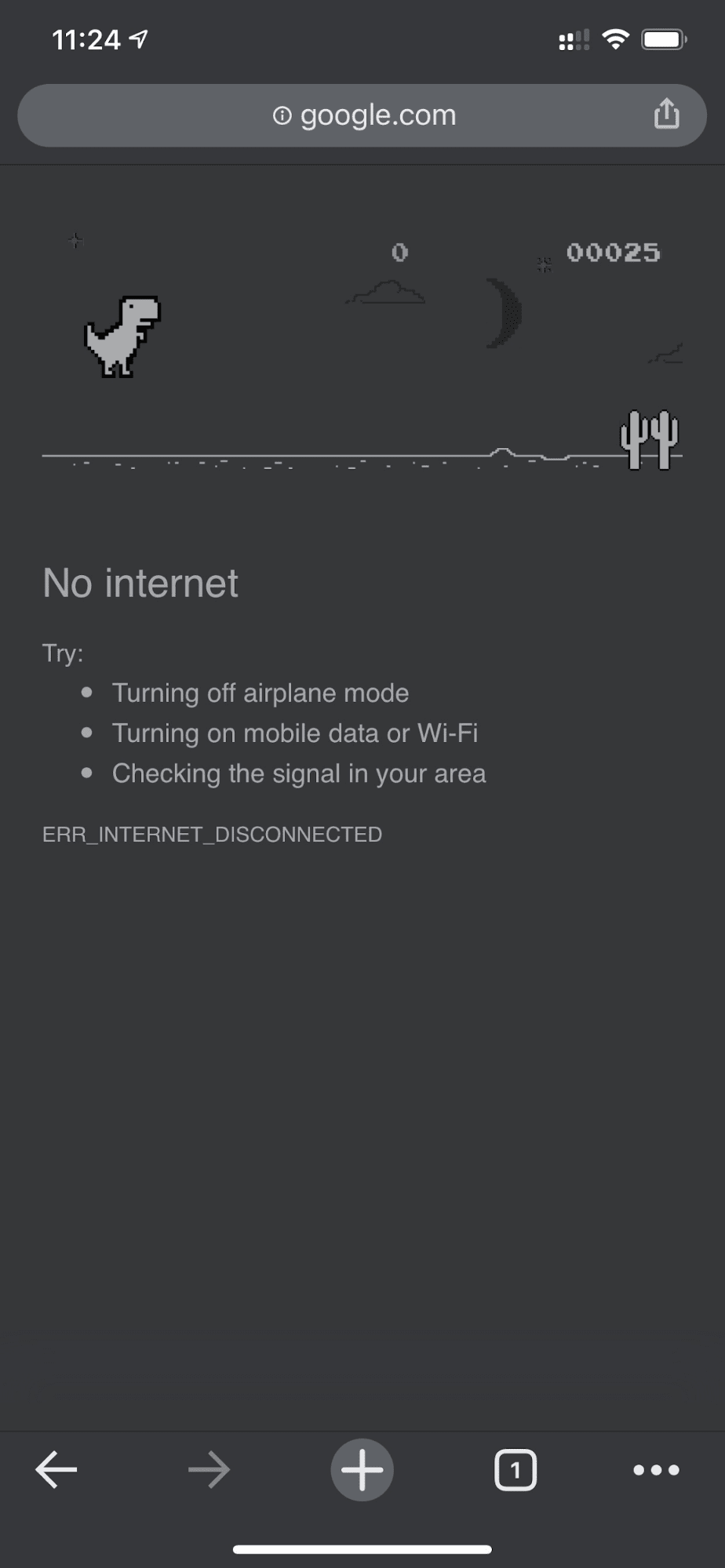
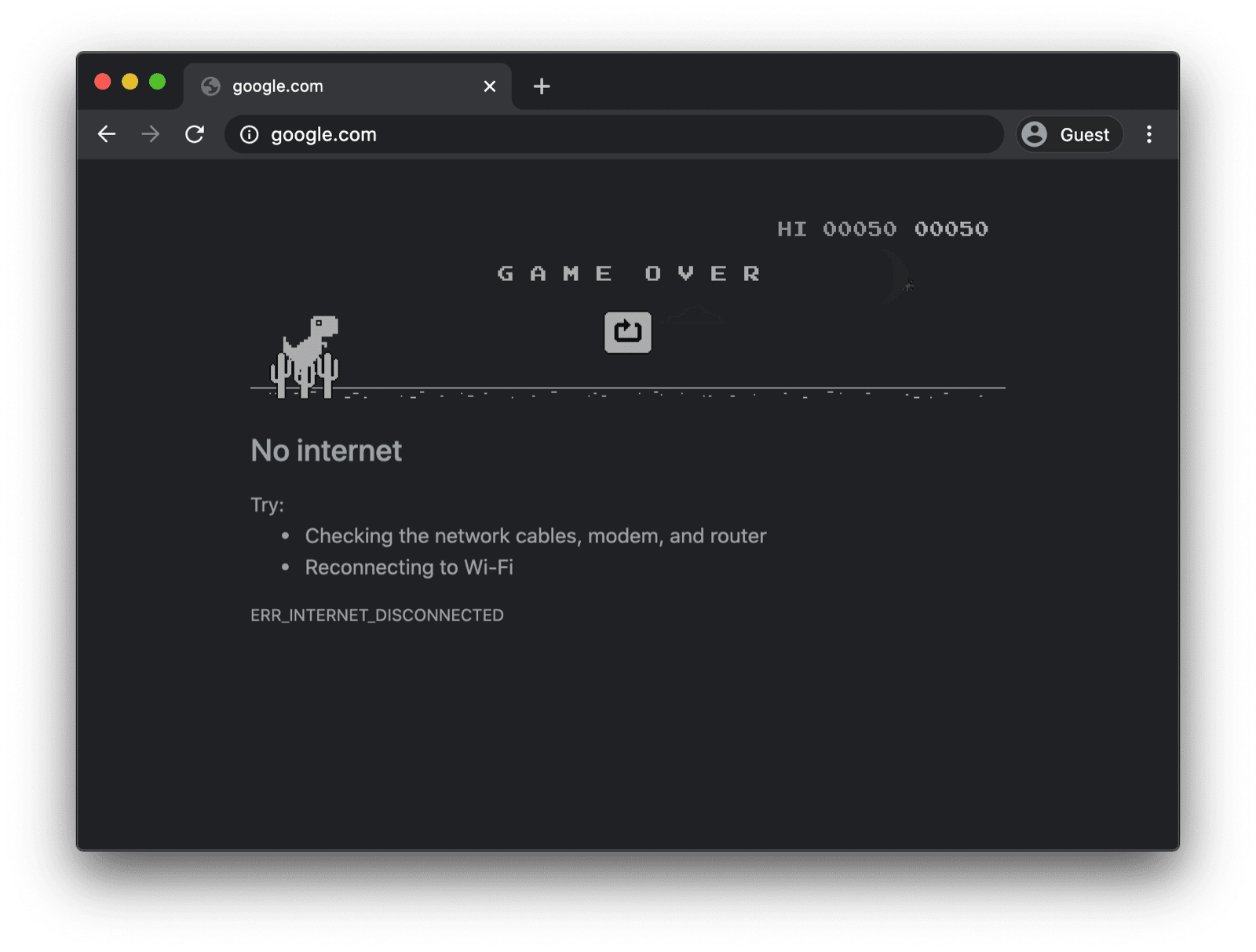
Uma página substituta off-line com um service worker personalizado
No entanto, não precisa ser assim. Graças aos service workers e à API Cache Storage, você pode fornecer uma experiência off-line personalizada para seus usuários. Pode ser uma página de marca simples com as informações de que o usuário está off-line no momento, mas também pode ser uma solução mais criativa, como, por exemplo, o famoso jogo de labirinto off-line trivago com um botão Reconectar manual e uma contagem regressiva automática de tentativas de reconexão.
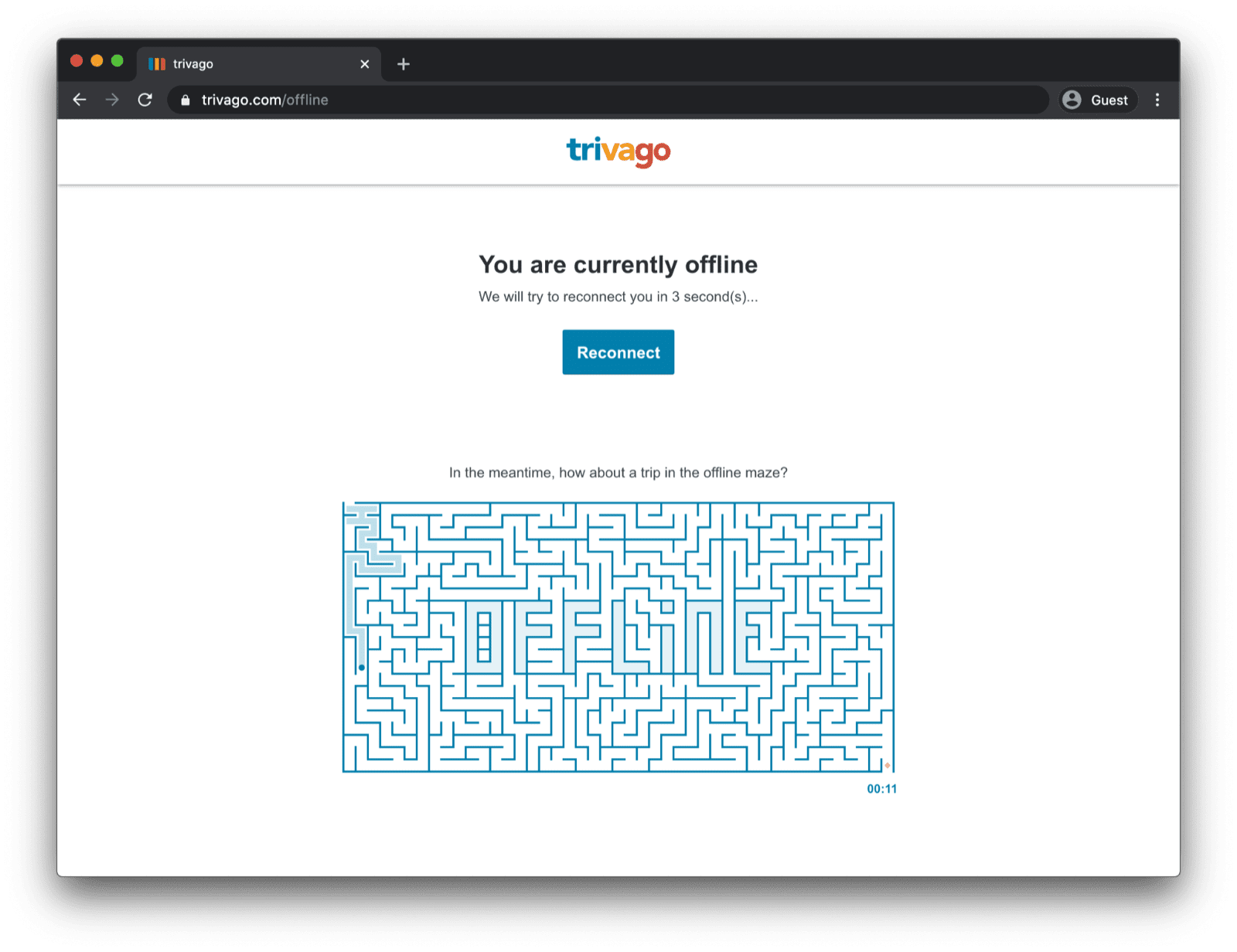
Como registrar o service worker
Para isso, use um service worker. É possível registrar um service worker na página principal, como no exemplo de código abaixo. Normalmente, isso é feito depois que o app é carregado.
window.addEventListener("load", () => {
if ("serviceWorker" in navigator) {
navigator.serviceWorker.register("service-worker.js");
}
});
O código do service worker
O conteúdo do arquivo do service worker real pode parecer um pouco envolvido à primeira vista, mas os
comentários no exemplo abaixo devem esclarecer tudo. A ideia principal é pré-armazenar em cache um arquivo chamado
offline.html
, que é veiculado apenas em solicitações de navegação com falha, e permitir que o navegador gerencie
todos os outros casos:
/*
Copyright 2015, 2019, 2020, 2021 Google LLC. All Rights Reserved.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
// Incrementing OFFLINE_VERSION will kick off the install event and force
// previously cached resources to be updated from the network.
// This variable is intentionally declared and unused.
// Add a comment for your linter if you want:
// eslint-disable-next-line no-unused-vars
const OFFLINE_VERSION = 1;
const CACHE_NAME = "offline";
// Customize this with a different URL if needed.
const OFFLINE_URL = "offline.html";
self.addEventListener("install", (event) => {
event.waitUntil(
(async () => {
const cache = await caches.open(CACHE_NAME);
// Setting {cache: 'reload'} in the new request ensures that the
// response isn't fulfilled from the HTTP cache; i.e., it will be
// from the network.
await cache.add(new Request(OFFLINE_URL, { cache: "reload" }));
})()
);
// Force the waiting service worker to become the active service worker.
self.skipWaiting();
});
self.addEventListener("activate", (event) => {
event.waitUntil(
(async () => {
// Enable navigation preload if it's supported.
// See https://developers.google.com/web/updates/2017/02/navigation-preload
if ("navigationPreload" in self.registration) {
await self.registration.navigationPreload.enable();
}
})()
);
// Tell the active service worker to take control of the page immediately.
self.clients.claim();
});
self.addEventListener("fetch", (event) => {
// Only call event.respondWith() if this is a navigation request
// for an HTML page.
if (event.request.mode === "navigate") {
event.respondWith(
(async () => {
try {
// First, try to use the navigation preload response if it's
// supported.
const preloadResponse = await event.preloadResponse;
if (preloadResponse) {
return preloadResponse;
}
// Always try the network first.
const networkResponse = await fetch(event.request);
return networkResponse;
} catch (error) {
// catch is only triggered if an exception is thrown, which is
// likely due to a network error.
// If fetch() returns a valid HTTP response with a response code in
// the 4xx or 5xx range, the catch() will NOT be called.
console.log("Fetch failed; returning offline page instead.", error);
const cache = await caches.open(CACHE_NAME);
const cachedResponse = await cache.match(OFFLINE_URL);
return cachedResponse;
}
})()
);
}
// If our if() condition is false, then this fetch handler won't
// intercept the request. If there are any other fetch handlers
// registered, they will get a chance to call event.respondWith().
// If no fetch handlers call event.respondWith(), the request
// will be handled by the browser as if there were no service
// worker involvement.
});
Página substituta off-line
O arquivo offline.html
é onde você usa a criatividade, adapta o elemento às suas necessidades e adiciona seu
branding. O exemplo abaixo mostra o mínimo do que é possível.
Ele demonstra o recarregamento manual com base no pressionamento de um botão e o recarregamento automático
com base no evento online
e na pesquisa regular do servidor.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<title>You are offline</title>
<!-- Inline the page's stylesheet. -->
<style>
body {
font-family: helvetica, arial, sans-serif;
margin: 2em;
}
h1 {
font-style: italic;
color: #373fff;
}
p {
margin-block: 1rem;
}
button {
display: block;
}
</style>
</head>
<body>
<h1>You are offline</h1>
<p>Click the button below to try reloading.</p>
<button type="button">⤾ Reload</button>
<!-- Inline the page's JavaScript file. -->
<script>
// Manual reload feature.
document.querySelector("button").addEventListener("click", () => {
window.location.reload();
});
// Listen to changes in the network state, reload when online.
// This handles the case when the device is completely offline.
window.addEventListener('online', () => {
window.location.reload();
});
// Check if the server is responding and reload the page if it is.
// This handles the case when the device is online, but the server
// is offline or misbehaving.
async function checkNetworkAndReload() {
try {
const response = await fetch('.');
// Verify we get a valid response from the server
if (response.status >= 200 && response.status < 500) {
window.location.reload();
return;
}
} catch {
// Unable to connect to the server, ignore.
}
window.setTimeout(checkNetworkAndReload, 2500);
}
checkNetworkAndReload();
</script>
</body>
</html>
Demonstração
Veja a página de substituição off-line em ação na demonstração incorporada abaixo. Se você estiver interessado, pode explorar o código-fonte no Glitch.
Observação sobre como tornar seu app instalável
Agora que seu site tem uma página substituta off-line, talvez você esteja se perguntando sobre as próximas etapas. Para tornar o app instalável, é necessário adicionar um manifesto de app da Web e, opcionalmente, criar uma estratégia de instalação.
Observação sobre como veicular uma página substituta off-line com o Workbox.js
Talvez você já tenha ouvido falar do Workbox. Workbox é um conjunto de bibliotecas JavaScript para adicionar suporte off-line a apps da Web. Se você preferir programar menos código de service worker, use o roteiro do Workbox para uma página somente off-line.
A seguir, saiba como definir uma estratégia de instalação para seu app.