מה משותף לאפליקציית Google Assistant, לאפליקציית Slack, לאפליקציית Zoom וכמעט לכל אפליקציה אחרת הספציפית לפלטפורמה בטלפון או במחשב? נכון, תמיד יש משהו. גם אם אין חיבור לרשת, עדיין תוכלו לפתוח את אפליקציית Assistant, להיכנס ל-Slack או להפעיל את Zoom. יכול להיות שלא תקבלו משהו משמעותי במיוחד, או שאולי לא תוכלו להשיג את מה שרציתם להשיג, אבל לפחות תקבלו משהו והאפליקציה תהיה בשליטה.
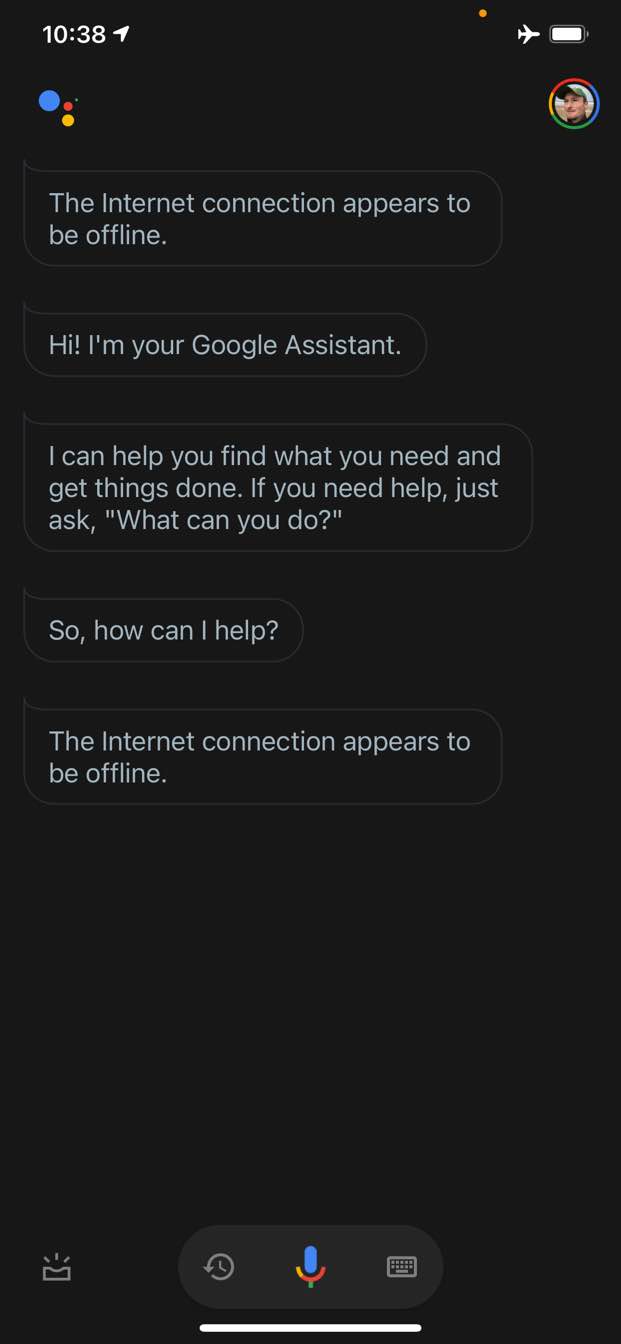
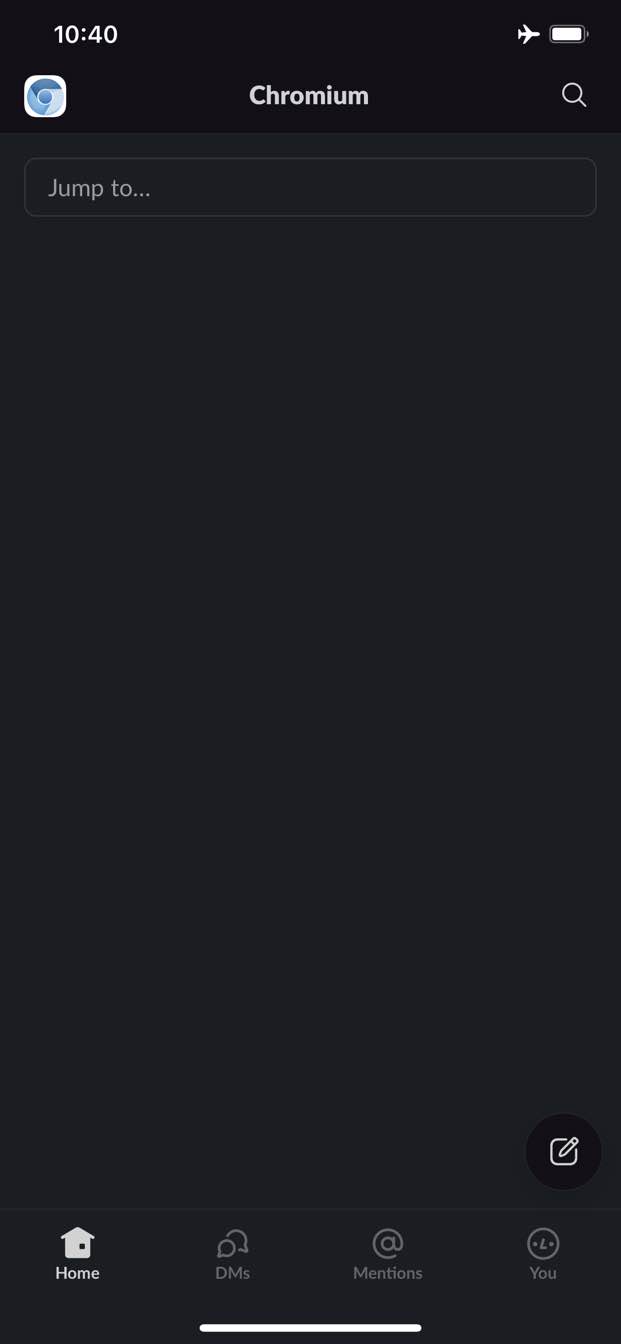
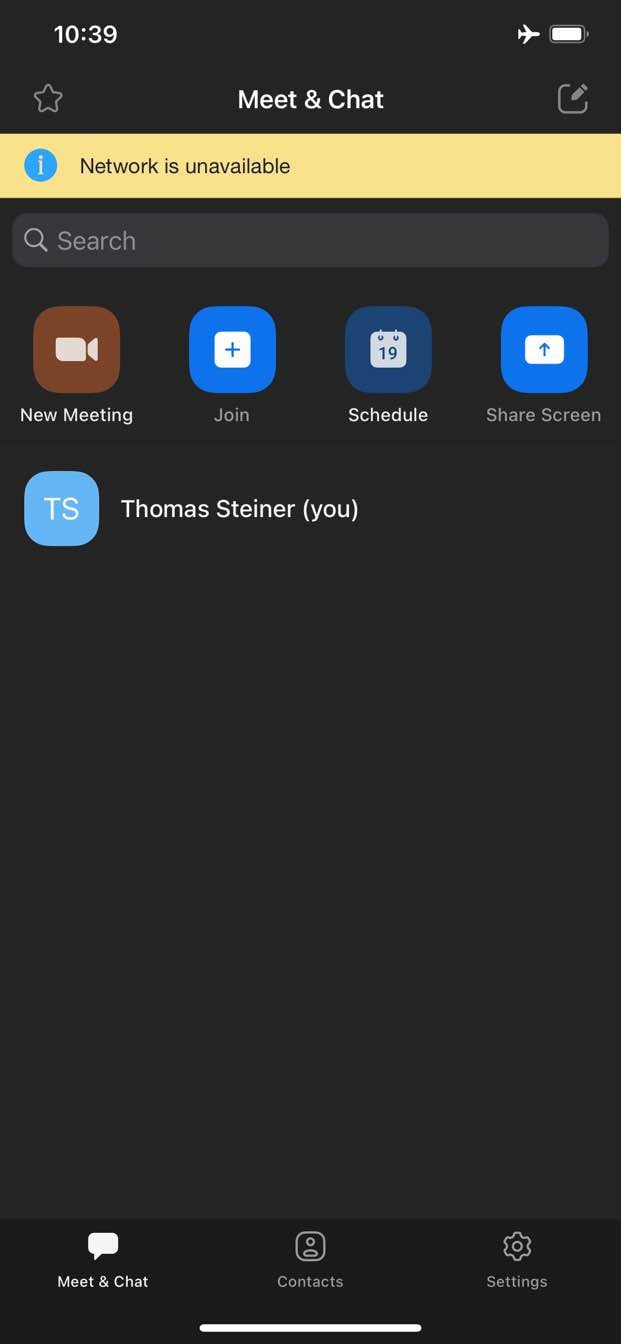
לעומת זאת, באינטרנט, בדרך כלל לא מקבלים שום דבר במצב אופליין. ב-Chrome יש משחק דינוזאורים אופליין, אבל זהו.
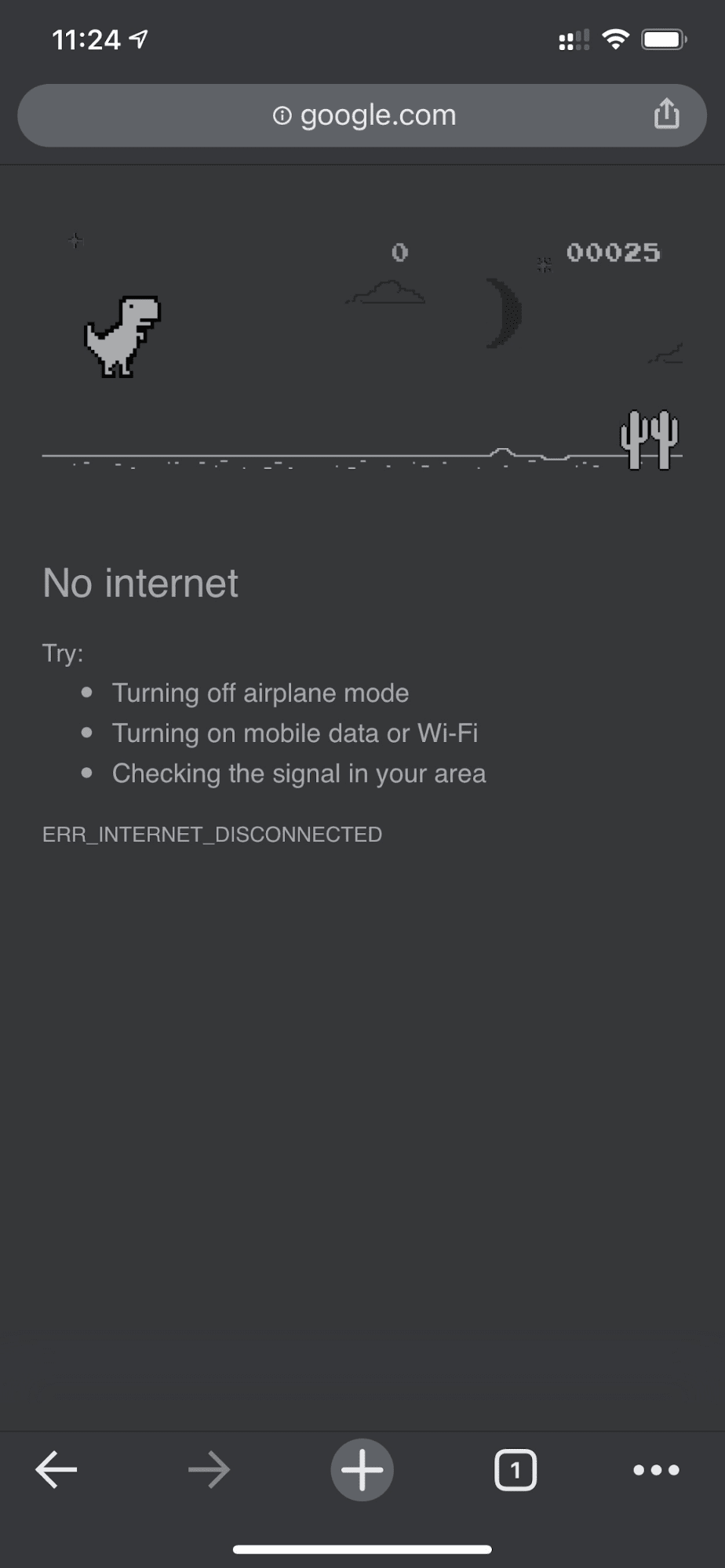
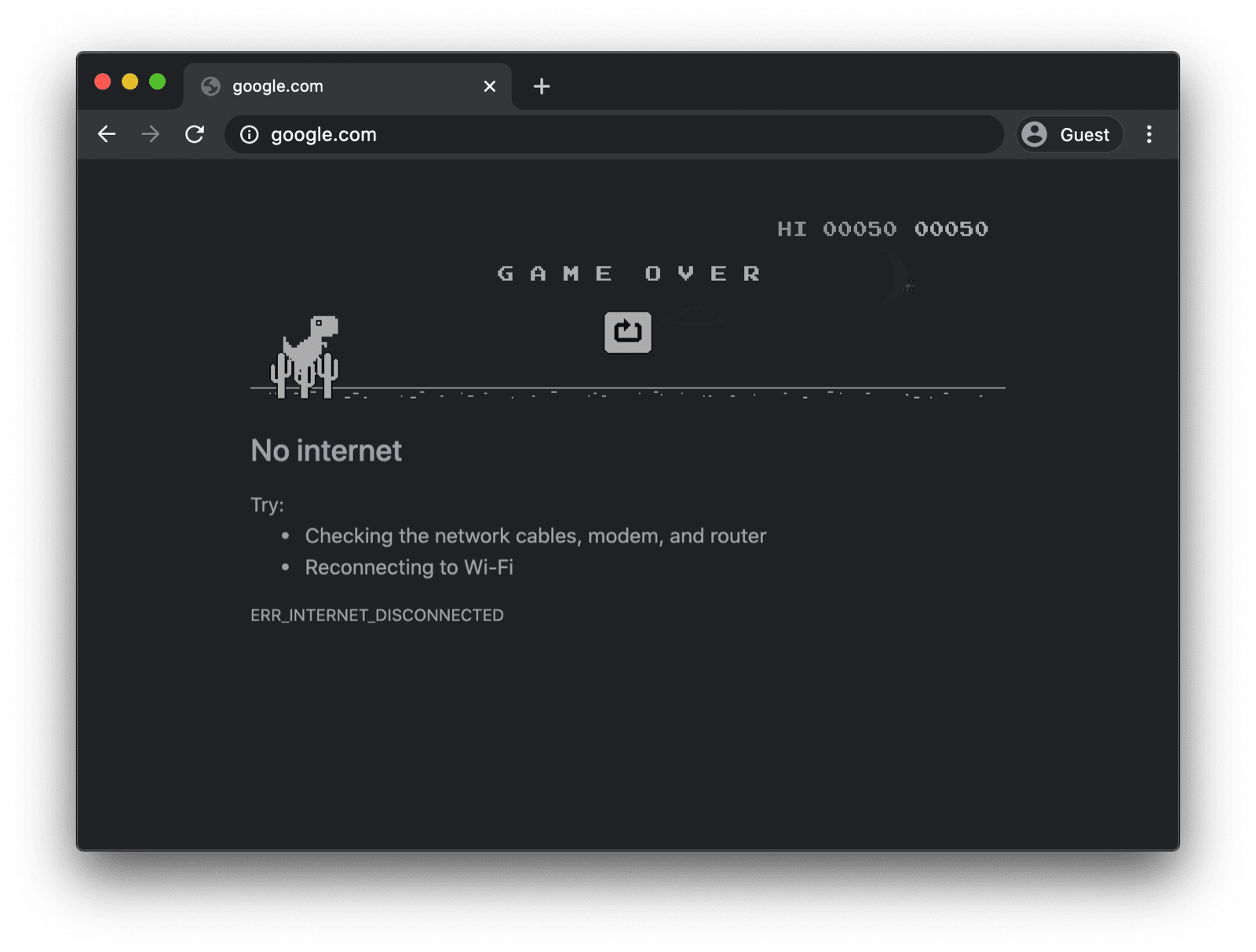
דף חלופי אופליין עם קובץ שירות (service worker) בהתאמה אישית
אבל זה לא חייב להיות כך. בעזרת service workers ו-Cache Storage API, אתם יכולים לספק למשתמשים חוויה מותאמת אישית אופליין. דף כזה יכול להיות דף פשוט ממותג שכולל את המידע שהמשתמש נמצא כרגע במצב אופליין, אבל הוא יכול להיות פתרון יצירתי יותר כמו משחק המבוך אופליין (trivago) אופליין המפורסם, שכולל לחצן Reconnect ידני וספירה לאחור של ניסיון התחברות מחדש אוטומטי.
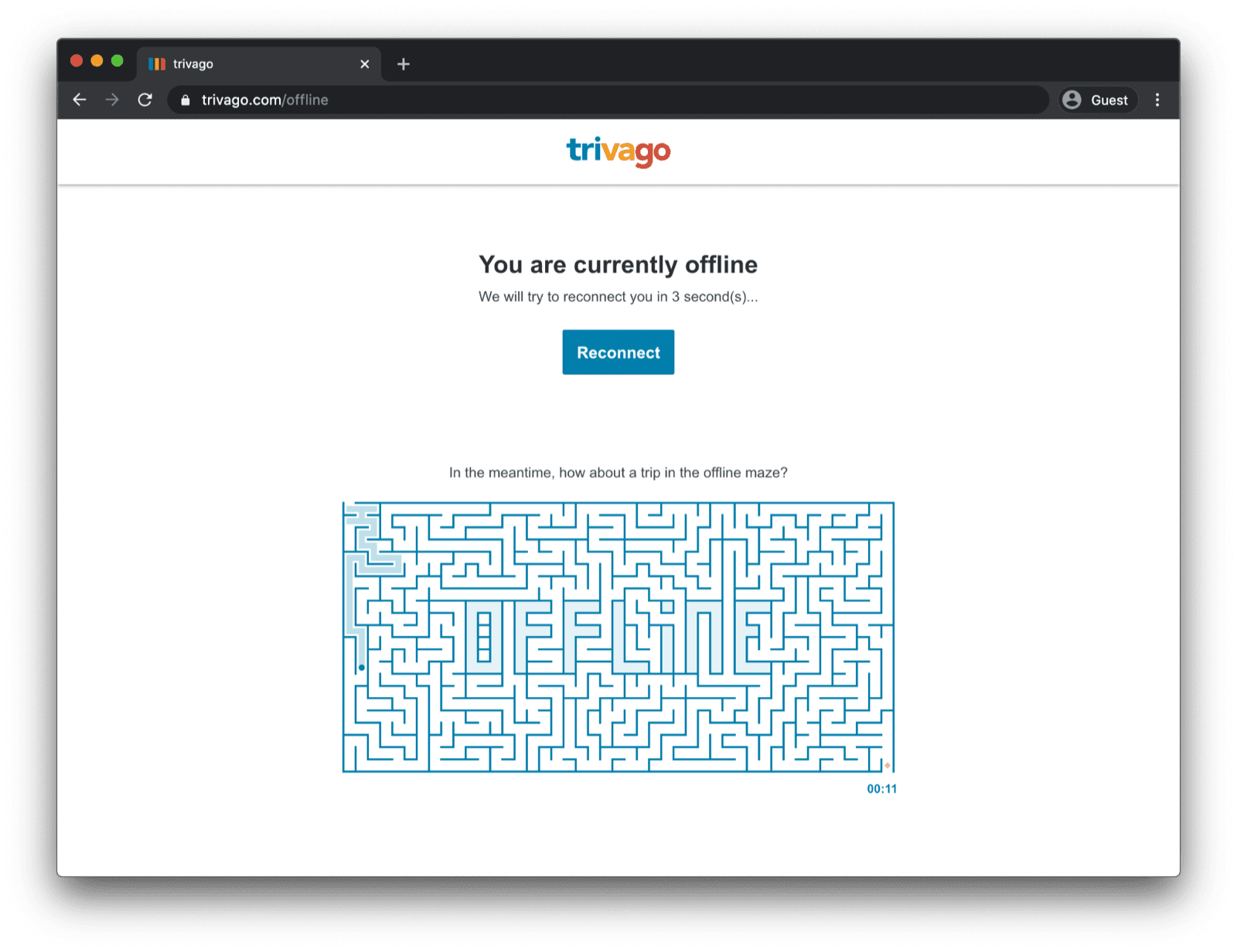
רישום קובץ השירות (service worker)
הדרך לעשות זאת היא באמצעות עובד שירות (service worker). תוכלו לרשום קובץ Service Worker מהדף הראשי כמו בדוגמת הקוד שבהמשך. בדרך כלל עושים זאת אחרי שהאפליקציה נטענת.
window.addEventListener("load", () => {
if ("serviceWorker" in navigator) {
navigator.serviceWorker.register("service-worker.js");
}
});
קוד קובץ השירות (service worker)
התוכן של קובץ ה-Service Worker נראה קצת מעורב במבט הראשון, אבל התגובות בדוגמה הבאה יבהירו לכם את העניין. הרעיון העיקרי הוא לשמור מראש קובץ בשם offline.html
שמוצג רק בבקשות ניווט נכשלות, ולאפשר לדפדפן לטפל בכל שאר המקרים:
/*
Copyright 2015, 2019, 2020, 2021 Google LLC. All Rights Reserved.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
// Incrementing OFFLINE_VERSION will kick off the install event and force
// previously cached resources to be updated from the network.
// This variable is intentionally declared and unused.
// Add a comment for your linter if you want:
// eslint-disable-next-line no-unused-vars
const OFFLINE_VERSION = 1;
const CACHE_NAME = "offline";
// Customize this with a different URL if needed.
const OFFLINE_URL = "offline.html";
self.addEventListener("install", (event) => {
event.waitUntil(
(async () => {
const cache = await caches.open(CACHE_NAME);
// Setting {cache: 'reload'} in the new request ensures that the
// response isn't fulfilled from the HTTP cache; i.e., it will be
// from the network.
await cache.add(new Request(OFFLINE_URL, { cache: "reload" }));
})()
);
// Force the waiting service worker to become the active service worker.
self.skipWaiting();
});
self.addEventListener("activate", (event) => {
event.waitUntil(
(async () => {
// Enable navigation preload if it's supported.
// See https://developers.google.com/web/updates/2017/02/navigation-preload
if ("navigationPreload" in self.registration) {
await self.registration.navigationPreload.enable();
}
})()
);
// Tell the active service worker to take control of the page immediately.
self.clients.claim();
});
self.addEventListener("fetch", (event) => {
// Only call event.respondWith() if this is a navigation request
// for an HTML page.
if (event.request.mode === "navigate") {
event.respondWith(
(async () => {
try {
// First, try to use the navigation preload response if it's
// supported.
const preloadResponse = await event.preloadResponse;
if (preloadResponse) {
return preloadResponse;
}
// Always try the network first.
const networkResponse = await fetch(event.request);
return networkResponse;
} catch (error) {
// catch is only triggered if an exception is thrown, which is
// likely due to a network error.
// If fetch() returns a valid HTTP response with a response code in
// the 4xx or 5xx range, the catch() will NOT be called.
console.log("Fetch failed; returning offline page instead.", error);
const cache = await caches.open(CACHE_NAME);
const cachedResponse = await cache.match(OFFLINE_URL);
return cachedResponse;
}
})()
);
}
// If our if() condition is false, then this fetch handler won't
// intercept the request. If there are any other fetch handlers
// registered, they will get a chance to call event.respondWith().
// If no fetch handlers call event.respondWith(), the request
// will be handled by the browser as if there were no service
// worker involvement.
});
הדף החלופי במצב אופליין
בקובץ offline.html
תוכלו להפעיל את היצירתיות ולהתאים אותו לצרכים שלכם, ולהוסיף את המיתוג שלכם. הדוגמה הבאה ממחישה את המינימום האפשרי.
הדוגמה ממחישה גם טעינת נתונים מחדש באופן ידני על ידי לחיצה על לחצן, וגם טעינת נתונים מחדש באופן אוטומטי על סמך האירוע online
ועל סמך סקרים רגילים של השרת.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<title>You are offline</title>
<!-- Inline the page's stylesheet. -->
<style>
body {
font-family: helvetica, arial, sans-serif;
margin: 2em;
}
h1 {
font-style: italic;
color: #373fff;
}
p {
margin-block: 1rem;
}
button {
display: block;
}
</style>
</head>
<body>
<h1>You are offline</h1>
<p>Click the button below to try reloading.</p>
<button type="button">⤾ Reload</button>
<!-- Inline the page's JavaScript file. -->
<script>
// Manual reload feature.
document.querySelector("button").addEventListener("click", () => {
window.location.reload();
});
// Listen to changes in the network state, reload when online.
// This handles the case when the device is completely offline.
window.addEventListener('online', () => {
window.location.reload();
});
// Check if the server is responding and reload the page if it is.
// This handles the case when the device is online, but the server
// is offline or misbehaving.
async function checkNetworkAndReload() {
try {
const response = await fetch('.');
// Verify we get a valid response from the server
if (response.status >= 200 && response.status < 500) {
window.location.reload();
return;
}
} catch {
// Unable to connect to the server, ignore.
}
window.setTimeout(checkNetworkAndReload, 2500);
}
checkNetworkAndReload();
</script>
</body>
</html>
הדגמה (דמו)
אתם יכולים לראות את דף החלופה למצב אופליין בפעולה בהדגמה שמוטמעת בהמשך. אם אתם רוצים, אתם יכולים לעיין בקוד המקור ב-Glitch.
הערה נוספת לגבי הגדרת האפליקציה להתקנה
עכשיו, אחרי שהוספת לאתר דף חלופי אופליין, יכול להיות שתרצו לדעת מהם השלבים הבאים. כדי לאפשר התקנה של האפליקציה, צריך להוסיף מניפסט של אפליקציית אינטרנט, ואפשר גם לפתח אסטרטגיית התקנה.
הערה לגבי הצגת דף חלופי אופליין באמצעות Workbox.js
יכול להיות ששמעתם על Workbox. Workbox היא קבוצה של ספריות JavaScript להוספת תמיכה במצב אופליין לאפליקציות אינטרנט. אם אתם מעדיפים לכתוב פחות קוד של קובץ שירות בעצמכם, תוכלו להשתמש במתכון של Workbox לדף אופליין בלבד.
בשלב הבא נסביר איך מגדירים אסטרטגיית התקנות לאפליקציה.