Pliki CSS to zasoby blokujące renderowanie: muszą zostać załadowane i przetworzone, zanim przeglądarka zacznie renderować stronę. Strony internetowe zawierające niepotrzebnie duże arkusze stylów zajmują więcej czasu na renderowanie.
Z tego przewodnika dowiesz się, jak opóźniać niekrytyczne elementy kodu CSS, aby zoptymalizować ścieżkę renderowania krytycznego i poprawić wskaźnik First Contentful Paint (FCP).
Przykład: nieoptymalne wczytywanie kodu CSS
Ten przykład zawiera harmonijkę z 3 ukrytymi akapitami tekstu, z których każdy ma inny styl:
Ta strona wymaga pliku CSS z 8 klasami, ale nie wszystkie z nich są niezbędne do renderowania „widocznej” treści.
Celem tego przewodnika jest zoptymalizowanie strony tak, aby tylko ważne style były wczytywane synchronicznie, a pozostałe (w tym style akapitów) – w sposób niezablokowany.
Pomiary
Uruchom Lighthouse na stronie i otwórz sekcję Wydajność.
Raport pokazuje wskaźnik First Contentful Paint o wartości „1 s” oraz możliwość Usuń zasoby blokujące renderowanie, która wskazuje na plik style.css:
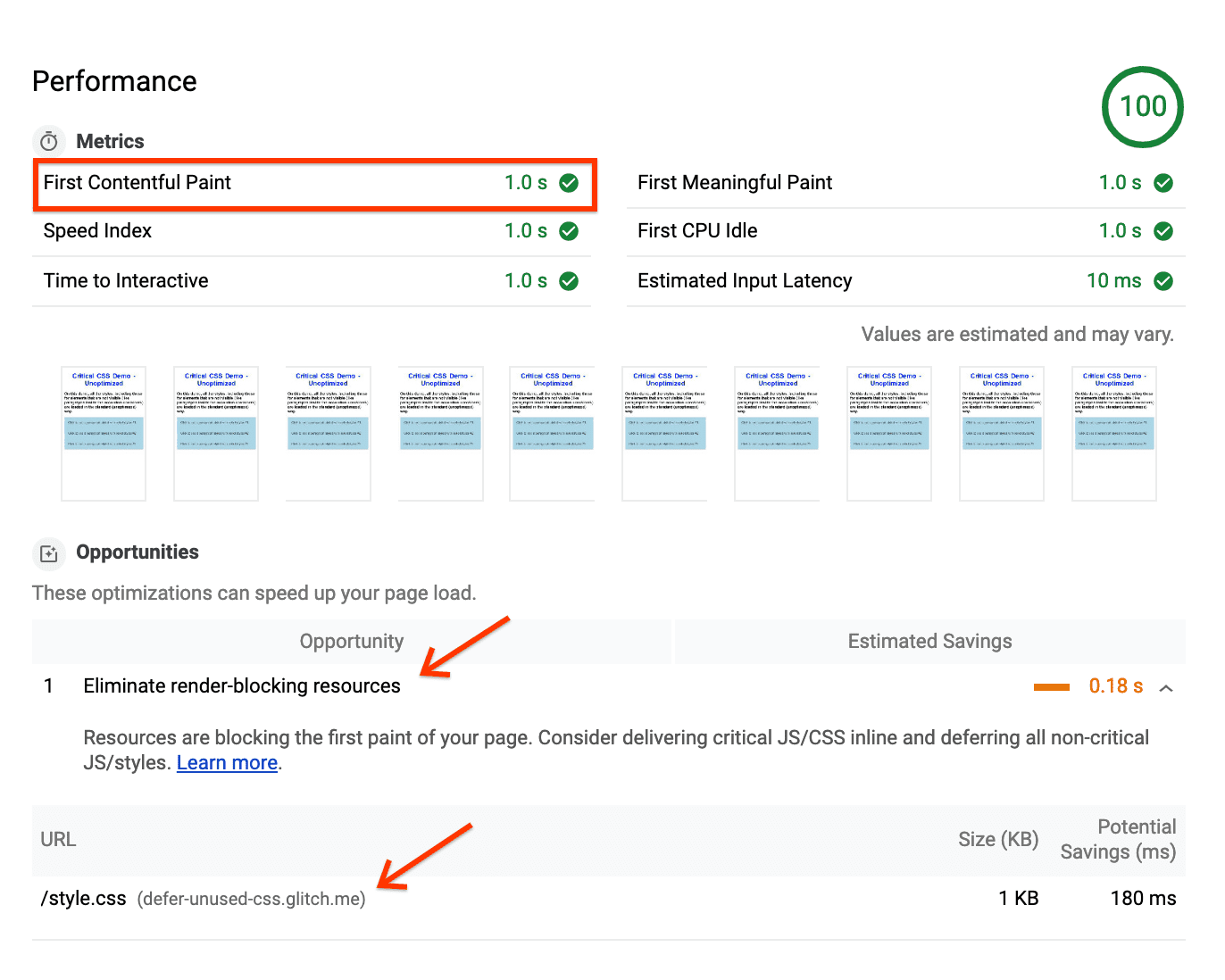
Aby zobaczyć, jak kod CSS blokuje renderowanie:
- Otwórz stronę w Chrome.
- Aby otworzyć Narzędzia dla programistów, naciśnij
Control+Shift+J
(lubCommand+Option+J
na Macu). - Kliknij kartę Skuteczność.
- W panelu Skuteczność kliknij Odśwież.
W wynikiem śledzeniu zobaczysz, że znacznik FCP jest umieszczony bezpośrednio po zakończeniu wczytywania CSS:
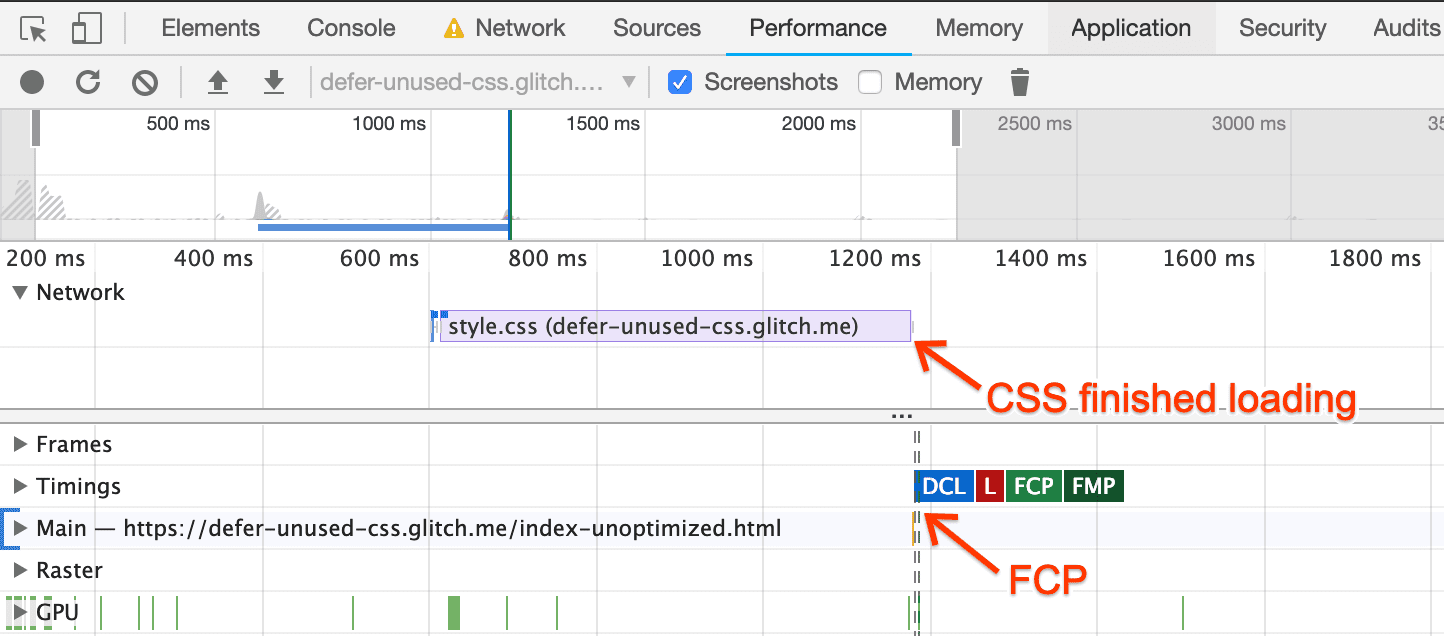
Oznacza to, że przeglądarka musi zaczekać, aż wszystkie pliki CSS zostaną załadowane i przetworzone, zanim na ekranie pojawi się choćby 1 piksel.
Optymalizuj
Aby zoptymalizować tę stronę, musisz wiedzieć, które klasy są uważane za krytyczne. Aby to sprawdzić, użyj narzędzia do sprawdzania zasięgu:
- W Narzędziach dla programistów otwórz Menu poleceń, naciskając
Control+Shift+P
lubCommand+Shift+P
(Mac). - Wpisz „Zasięg” i kliknij Pokaż zasięg.
- Kliknij Odśwież, aby załadować ponownie stronę i rozpocząć rejestrowanie pokrycia.

Aby wyświetlić szczegóły, kliknij dwukrotnie raport:
- Klasy oznaczone na zielono są krytyczne. Przeglądarka potrzebuje ich do renderowania widocznych treści, w tym tytułu, napisów i przycisków harmonijki.
- Klasy oznaczone na czerwono są nieistotne i dotyczą tylko treści, które nie są widoczne od razu, takich jak ukryte akapity.
Za pomocą tych informacji zoptymalizuj pliki CSS, aby przeglądarka mogła rozpocząć przetwarzanie ważnych stylów natychmiast po załadowaniu strony, a nieistotnych przesunąć na później:
Wyodrębnij definicje klas oznaczone na zielono w raporcie pokrycia i umieścić te klasy w bloku
<style>
u góry strony:<style type="text/css"> .accordion-btn {background-color: #ADD8E6;color: #444;cursor: pointer;padding: 18px;width: 100%;border: none;text-align: left;outline: none;font-size: 15px;transition: 0.4s;}.container {padding: 0 18px;display: none;background-color: white;overflow: hidden;}h1 {word-spacing: 5px;color: blue;font-weight: bold;text-align: center;} </style>
Ładuj pozostałe klasy asynchronicznie, stosując ten wzór:
<link rel="preload" href="styles.css" as="style" onload="this.onload=null;this.rel='stylesheet'"> <noscript><link rel="stylesheet" href="styles.css"></noscript>
To nie jest standardowy sposób wczytywania CSS. Jak to działa:
link rel="preload" as="style"
prosi o arkusz stylów asynchronicznie. Więcej informacji opreload
znajdziesz w przewodniku Wstępne wczytywanie kluczowych komponentów.- Atrybut
onload
w tagulink
pozwala przeglądarce przetworzyć kod CSS po zakończeniu wczytywania arkusza stylów. - „Zanulenie” modułu obsługi
onload
po jego użyciu pomaga niektórym przeglądarkom uniknąć ponownego wywołania modułu obsługi po przełączeniu atrybuturel
. - Odwołanie do arkusza stylów wewnątrz elementu
noscript
stanowi rozwiązanie alternatywne dla przeglądarek, które nie wykonują kodu JavaScript.
Otrzymana strona wygląda dokładnie tak samo jak poprzednia wersja, nawet jeśli większość stylów wczytuje się asynchronicznie. Oto jak wyglądają w pliku HTML osadzone style i żądanie asynchroniczne do pliku CSS:
Monitorowanie
Użyj Narzędzi deweloperskich, aby przeprowadzić kolejne śledzenie Skuteczność na zoptymalizowanej stronie.
Marker FCP pojawia się przed wysłaniem przez stronę żądania pliku CSS, co oznacza, że przeglądarka nie musi czekać na załadowanie pliku CSS przed wyrenderowaniem strony:
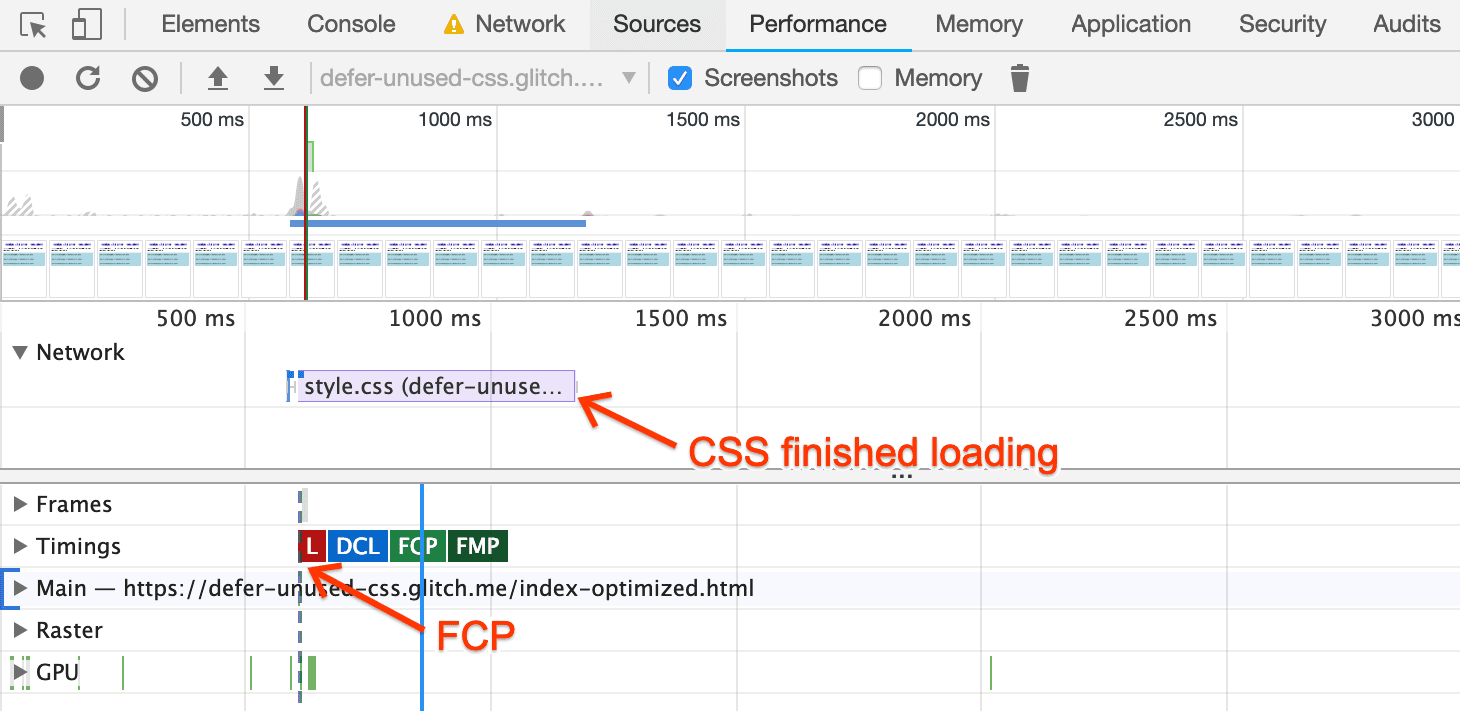
Na koniec uruchom Lighthouse na stronie zoptymalizowanej.
W raporcie zobaczysz, że czas wczytywania strony FCP został skrócony o 0,2 s (to aż 20% osiągniętej poprawy):
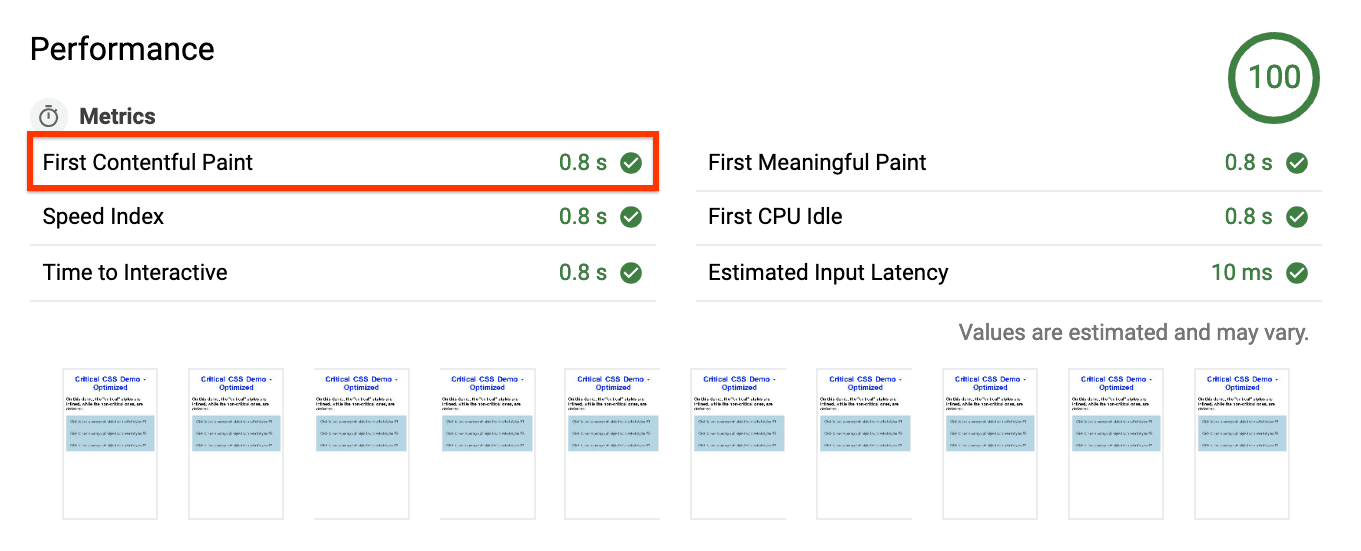
Sugerowanie Usuń zasoby blokujące renderowanie nie pojawia się już w sekcji Możliwości, ale w sekcji Przeprowadzone kontrole:
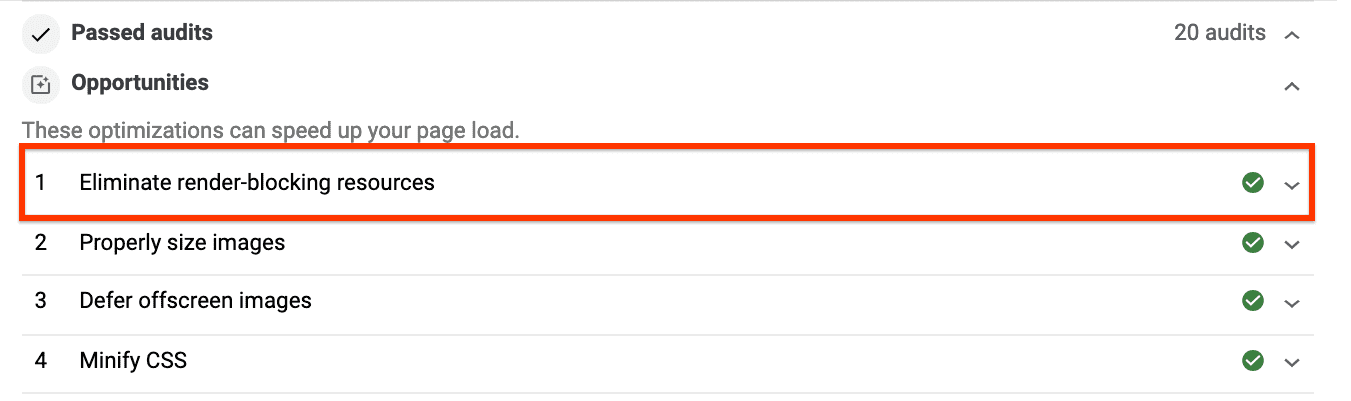
Dalsze kroki i materiały dodatkowe
Z tego przewodnika dowiesz się, jak opóźnić niekrytyczne pliki CSS, ręcznie wyodrębniając nieużywany kod na stronie. W przypadku bardziej złożonych środowisk produkcyjnych przewodnik po wyodrębnianiu kluczowego kodu CSS zawiera informacje o najpopularniejszych narzędziach do wyodrębniania kluczowego kodu CSS. Znajdziesz w nim też laboratorium kodu, w którym możesz sprawdzić, jak te narzędzia działają w praktyce.